Pillow – Change Image Contrast
Contrast is the kind of distance between the colors. If you increase the contrast, the colors are more vivid.
In this tutorial, we shall learn how to change the contrast of image using ImageEnhance class of PIL library.
Steps to adjust image contrast
To adjust image contrast using Python Pillow,
- Read the image using Image.open().
- Create ImageEnhance.Contrast() enhancer for the image.
- Enhance the image contrast using enhance() method, by the required factor.
By adjusting the factor you can adjust the contrast of the image.
While a factor of 1 gives original image. Making the factor towards 0 makes the image greyer, while factor>1 increases the contrast of the image.
Examples
1. Increase the contrast of given image
In the following example, we increase the contrast of given image by a factor of 1.5, which increases the image’s contrast.
Python Program
from PIL import Image, ImageEnhance
#read the image
im = Image.open("sample-image.png")
#image brightness enhancer
enhancer = ImageEnhance.Contrast(im)
factor = 1 #gives original image
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 1.5 #increase contrast
im_output = enhancer.enhance(factor)
im_output.save('more-contrast-image.png')
Original Image
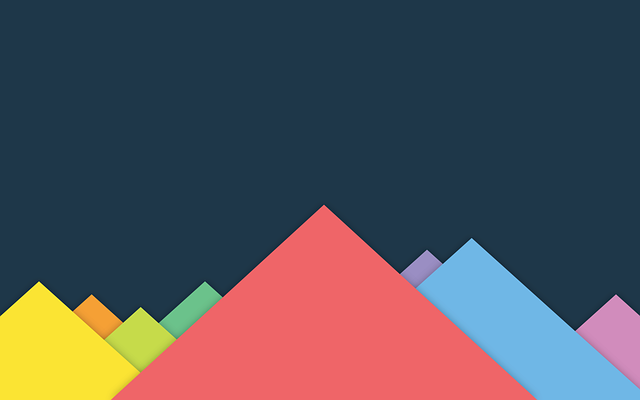
Image with Increase in Contrast
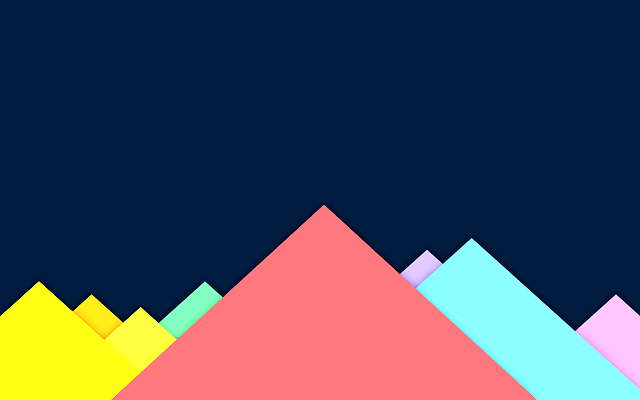
2. Decrease the contrast of given image
In the following example, we decrease the contrast of given image by a factor of 0.5, which greys out the image.
Python Program
from PIL import Image, ImageEnhance
#read the image
im = Image.open("sample-image.png")
#image brightness enhancer
enhancer = ImageEnhance.Contrast(im)
factor = 1 #gives original image
im_output = enhancer.enhance(factor)
im_output.save('original-image.png')
factor = 0.5 #decrease constrast
im_output = enhancer.enhance(factor)
im_output.save('less-contrast-image.png')
Original Image
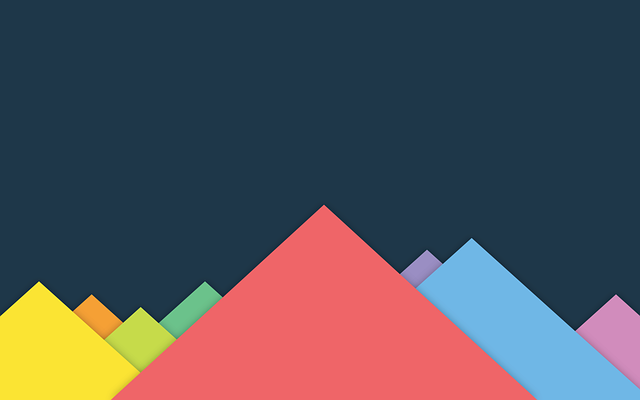
Dull Image – With decreased contrast
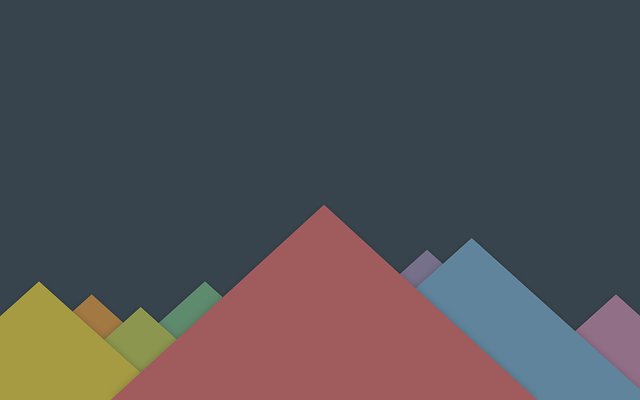
Summary
In this tutorial of Python Examples, we learned how to change the contrast of image, using Pillow library, with the help of well detailed example programs.