Contents
Python Pillow – Compress Image
You can compress an image using Pillow library in Python to a relatively lesser size in memory.
To compress an image using Pillow, call Image.save() method on the Image object, and specify True for optimize parameter, and an integer value for quality parameter.
A quick code snippet would be as shown in the following.
input_image.save('compressed_image.jpg', optimize=True, quality=75)
CopyThe integer value given to quality parameter specifies the level of compression. For example, if quality is set to 75, then only 75% of the quality is retained and the image is compress in size accordingly. Similarly, for a quality of 25, only 25% quality of the image is retained and the image is compressed higher.
Lesser the quality, more is the compression.
We shall use the following image as input for image compression using Pillow.
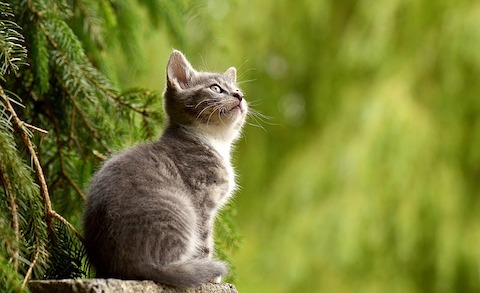
1. Compress image with 75% quality using Pillow
In this example, we shall write a Python program that uses Pillow library to compress given input image with a quality of 75%.
We shall then compare the disk space occupied by the input and output images.
Python Program
from PIL import Image
# Input image file
input_image = Image.open('input_image.jpg')
# Compress image
input_image.save('compressed_image.jpg', optimize=True, quality=75)
# Close input image
input_image.close()
CopyCompressed Image
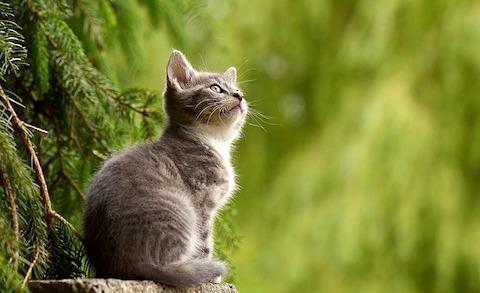
File size in disk
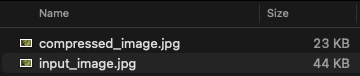
The compressed image is of size 23KB while the input image is of size 44KB. We got around 47% compression (1 -(23/44))*100 in terms of file size, with the quality of 75%.
2. Compress image with 40% quality using Pillow
In this example, we shall compress the given input image with a quality of 40%. We have given a relatively lesser quality to be retained in the output image. Therefore, the image compression must be higher than that of in previous example.
Python Program
from PIL import Image
# Input image file
input_image = Image.open('input_image.jpg')
# Compress image
input_image.save('compressed_image.jpg', optimize=True, quality=40)
# Close input image
input_image.close()
CopyCompressed Image
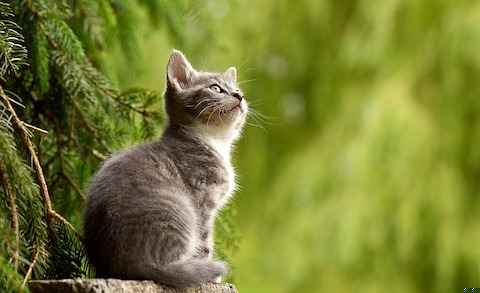
File size in disk
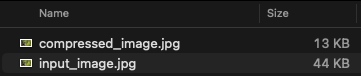
The compressed image is of size 14KB while the input image is of size 44KB. We got around 70% compression (1 -(14/44))*100 in terms of file size, with the quality of 40%.
3. Compress image with 5% quality using Pillow
Let us go a little greedy and check the image output and image compression for a quality of 10%.
Python Program
from PIL import Image
# Input image file
input_image = Image.open('input_image.jpg')
# Compress image
input_image.save('compressed_image.jpg', optimize=True, quality=5)
# Close input image
input_image.close()
CopyCompressed Image
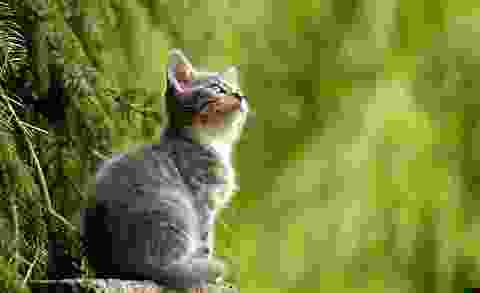
The image looks pixelated.
File size in disk
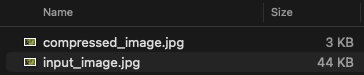
The compressed image is of size 3KB while the input image is of size 44KB. We got around 93% compression (1 -(3/44))*100 in terms of file size, with the quality of 40%.
Summary
In this Python Pillow tutorial, we learned how to compress an image using Pillow library in Python, with examples. We have taken an input image and observed the compression image, and the image compression for different quality values. You may choose required quality based on the compression requirement that you have in your application.