Contents
Python Pillow – Convert Image to Numpy Array
You can convert an image into a NumPy array using Python Pillow (PIL) library and NumPy library in Python.
To convert an image into NumPy array, open the image using Image.open() of Pillow library, and then pass the returned Image object as argument to the numpy.array() of NumPy library. The numpy.array() returns a NumPy array created from the Image.
Let us go through some examples of converting given image into NumPy array.
1. Converting image into NumPy array using Pillow and NumPy in Python
We are given an image input_image.jpg. We have to convert this image into a NumPy array.
Steps
- Open given image using Image.open(). The open() function returns an Image object created from the image at given file path.
original_image = Image.open('input_image.jpg')
- Pass the Image object as argument to the numpy.array() function. The numpy.array() function returns a NumPy array created from the color channels of the given Image object.
image_array = np.array(image)
- You can use this NumPy array for further transformations, or image processing operations, or for anything based on your requirement. But, here let us just print the shape of the array.
print(image_array.shape)
Program
The complete program to convert the given image into NumPy array using Pillow is given below.
Python Program
from PIL import Image
import numpy as np
# Open the image file
image = Image.open('input_image.jpg')
# Convert the image to a NumPy array
image_array = np.array(image)
# Close the image
image.close()
# Now you can work with the NumPy array
print(image_array.shape)
Copyinput_image.jpg
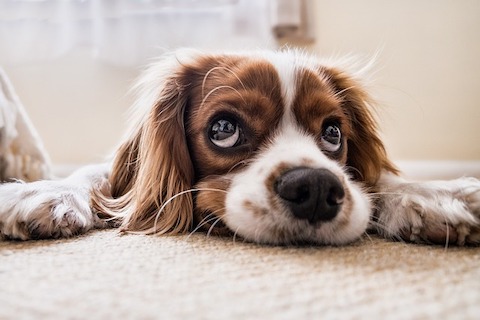
Output
(320, 480, 3)
Summary
In this Python Pillow tutorial, we learned how to how to convert a given image into a NumPy array using Pillow and NumPy libraries of Python, with examples. In the example, we have taken an input image, then converted this image to a NumPy array, and accessed the shape of NumPy array.