Python Pillow – Convert Numpy Array to Image
You can convert a NumPy array to image using Python Pillow (PIL) library in Python.
To convert a given NumPy array to an image using Pillow library in Python, call Image.fromarray() function and pass the given NumPy array as argument. The function returns a PIL Image object. You can then save this Image object as an image file in the disk storage.
Let us go through some examples of converting given NumPy array into an image.
1. Converting NumPy array into image using Pillow Image.fromarray() in Python
We are given a NumPy array in arr
. We have to convert array into image using Pillow library.
Steps
- Given NumPy array in arr. For demonstration purpose, we shall create a NumPy array of our own, and take it in arr.
- Pass the NumPy as argument to the Image.fromarray() function of Pillow library. The fromarray() function returns a Pillow Image object.
image = Image.fromarray(numpy_array)
- You can use save this image to disk storage using Image.save() function.
image.save('output_image.png')
- Now, you may close the image.
image.close()
Program
The complete program to convert the given NumPy array into an image using Pillow is given below.
Python Program
from PIL import Image
import numpy as np
# Create a NumPy array
numpy_array = np.ones((300, 400, 3), dtype=np.uint8)
# Setting green channel to 255
numpy_array[:,:,1] = 155
# Convert the NumPy array to an image
image = Image.fromarray(numpy_array)
# Save the image to disk storage
image.save('output_image.jpg', format="JPEG")
# Close the image
image.close()
output_image.jpg
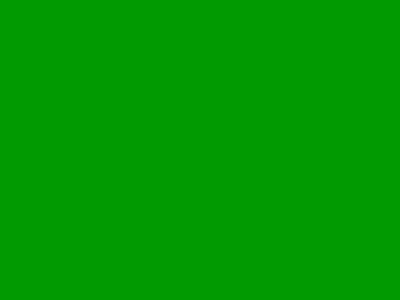
Summary
In this Python Pillow tutorial, we learned how to how to convert a given NumPy array into an image using Pillow library of Python, with examples. In the example, we have taken a sample numpy array that we created by ourselves, then converted this NumPy array to an image, and saved it to storage.