Python Pillow - Create Image
Python Pillow - Create Image
To create a new image using Python Pillow PIL library, use Image.new() method.
In this tutorial we will learn the syntax of Image.new() method, and how to use this method to create new images in Python.
Syntax of Image.new()
The syntax of Image.new() method is
new(mode, size, color=0)
where
- mode is the image mode. For example RGB, RGBA, CMYK, etc.
- size is the tuple with width and height of the image as elements. Width and height values are in pixels.
- color is for painting all the pixels with. Based on the mode, values can be provided for each band, altogether as a tuple. color parameter is optional and the default value of color is 0.
Examples
1. Create a new RGB image of size 400x300
In this example, we will create a new image with RGB mode, (400, 300) as size. We shall not give any color, so new() methods considers the default value of 0 for color. 0 for RGB channels would be black color.
Python Program
from PIL import Image
width = 400
height = 300
img = Image.new( mode = "RGB", size = (width, height) )
img.show()
Output
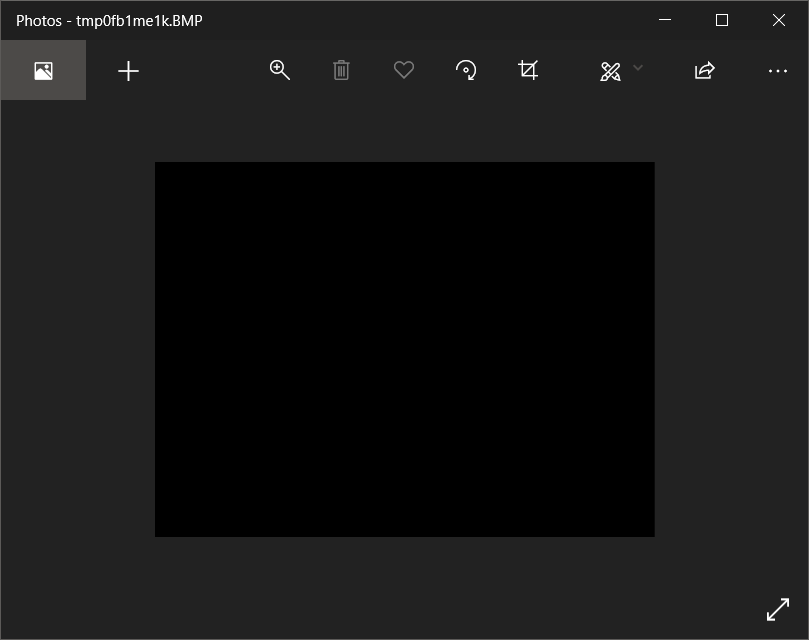
show() method will display the image in your PC using default image viewing application.
2. Create an image with specific background color
In this example, we will create a new image with RGB mode, (400, 300) as size and a color of (209, 123, 193) corresponding to Red, Green and Blue channels respectively.
Python Program
from PIL import Image
width = 400
height = 300
img = Image.new( mode = "RGB", size = (width, height), color = (209, 123, 193) )
img.show()
Output
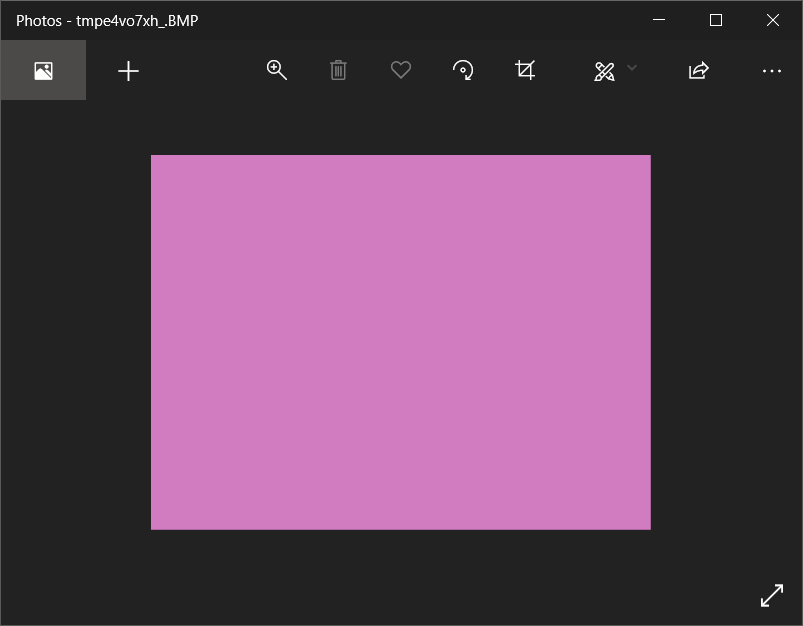
3. Create image with specific color mode
In our previous examples, we have taken RGB mode to create image. Let us try with another mode like CMYK.
Python Program
from PIL import Image
width = 400
height = 300
img = Image.new( mode = "CMYK", size = (width, height), color = (209, 123, 193, 100) )
img.show()
Output
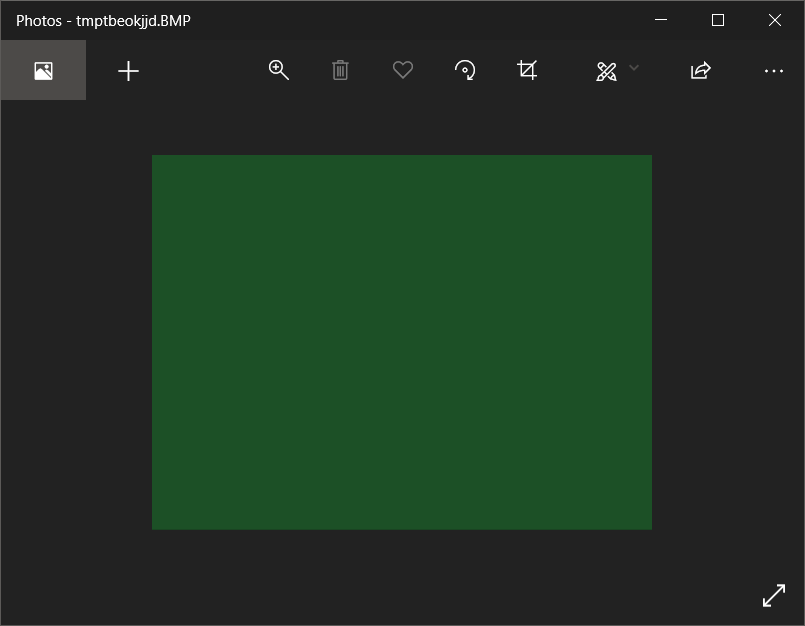
Summary
In this tutorial of Python Examples, we learned how to create a new image using Pillow library's Image.new() method, with the help of example programs.