Pillow – Find image edges
To find the edges in an image using Pillow library, you can use Image.filter() function with ImageFilter.FIND_EDGES kernel.
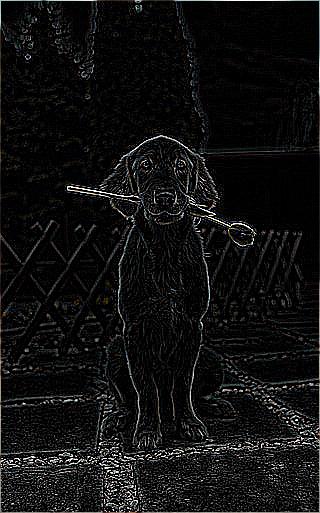
Steps to find edges in an image
Follow these steps to find edges in a given image.
- Import Image, and ImageFilter modules from Pillow library.
- Read input image using
Image.open()
function. - Call
filter()
function on the read Image object and passImageFilter.FIND_EDGES
as argument to the function. The function returns the edges image as PIL.Image.Image object. - Save the returned image to required location using
Image.save()
function.
Syntax of filter() function
The syntax of filter() function from PIL.Image module is
PIL.Image.filter(filter_kernel)
Parameter | Description |
---|---|
filter_kernel | A filter kernel that applies to the given image. To find edge of this image, you can use ImageFilter.FIND_EDGES filter kernel. |
Returns
The function returns a PIL.Image.Image object.
Examples
1. Find edges in given image
In the following example, we read an image test_image.jpg
, find edges in this image using Image.filter() function, and save the edge image as edges.jpg
.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# Find edges of the image
edges = image.filter(ImageFilter.FIND_EDGES)
# Save the resulting image
edges.save("edges.jpg")
Original Image [test_image.jpg]
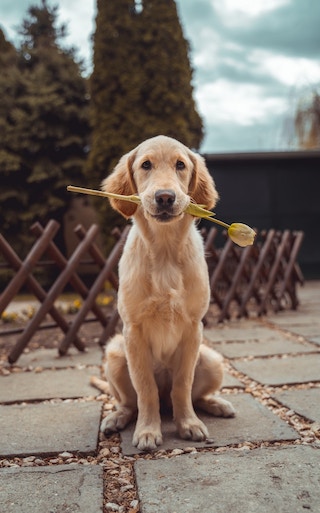
Resulting Image with FIND_EDGES filter [edges.jpg]
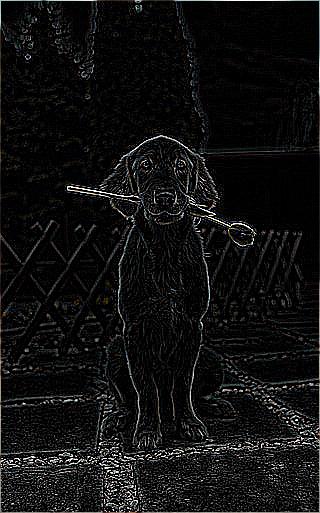
Summary
In this Python Pillow Tutorial, we learned how to find edges in a given image using PIL.Image.filter() function, with the help of examples.