Python Pillow – Flip Image
To flip an Image vertically or horizontally with Python pillow, use transpose() method on the PIL Image object.
In this tutorial, we shall learn how to transpose or flip an image, with the help of example programs.
Syntax of Image.transpose()
The syntax of transpose() method is:
Image.transpose(method)
where
- method – Possible values of method are
- PIL.Image.FLIP_LEFT_RIGHT
- PIL.Image.FLIP_TOP_BOTTOM
- PIL.Image.ROTATE_90
- PIL.Image.ROTATE_180
- PIL.Image.ROTATE_270
- PIL.Image.TRANSPOSE or PIL.Image.TRANSVERSE.
In this tutorial, we will only deal with
- PIL.Image.FLIP_LEFT_RIGHT which flips the image with respect to vertical axis.
- PIL.Image.FLIP_TOP_BOTTOM which flips the image with respect to horizontal axis.
Examples
1. Flip given image w.r.t. vertical axis
In the following example, we pass PIL.Image.FLIP_LEFT_RIGHT as argument to the transpose method to flip the image vertically or flip left to right and right to left.
Python Program
import PIL
from PIL import Image
#read the image
im = Image.open("sample-image.png")
#flip image
out = im.transpose(PIL.Image.FLIP_LEFT_RIGHT)
out.save('transpose-output.png')
Input Image
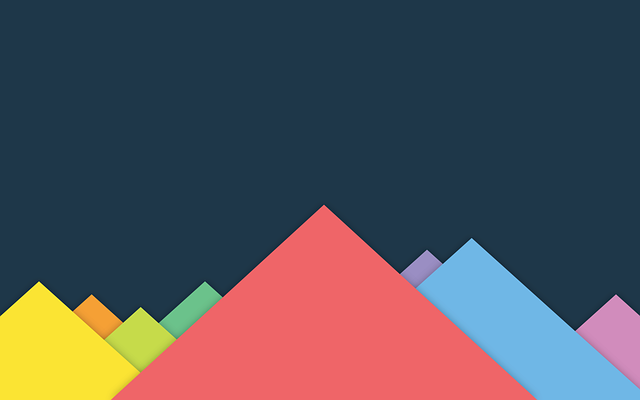
Output Image
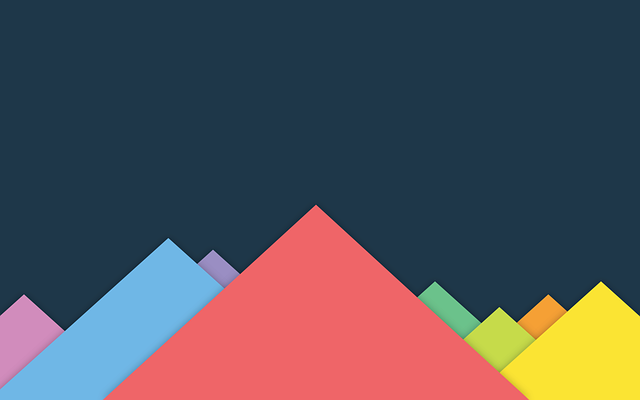
2. Flip Image w.r.t. horizontal axis
In the following example, we pass PIL.Image.FLIP_TOP_BOTTOM as argument to the transpose method to flip the image w.r.t. horizontal axis or flip top to bottom and bottom to top.
Python Program
import PIL
from PIL import Image
#read the image
im = Image.open("sample-image.png")
#flip image
out = im.transpose(PIL.Image.FLIP_TOP_BOTTOM)
out.save('transpose-output.png')
Output Image
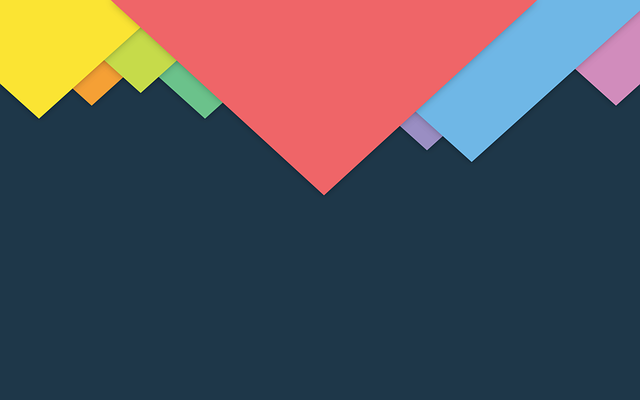
Summary
In this tutorial of Python Examples, we learned how to transpose an image, and thus get the flipping effect along vertical and horizontal axes.