Python Pillow - Image.size - Get Image Size
Python Pillow - Image Size
To retrieve the size of an image using Python Pillow, use the size property of the Image object. This property returns the width and height of the image as a tuple.
In this tutorial, we will learn how to get the size (width and height) of an image using the Pillow library.
Syntax - Image size property
The syntax to use the size property of the PIL Image object, referred to as image, is as follows:
image.size
Examples
In the following examples, we will demonstrate how to get the size of an image using the Image.size property.
1. Get size of an image using Pillow
In this example, we are given an image located at input_image.jpg. We will retrieve its size.
First, we open the image using the Image.open()
function, which returns an Image object. Then, we access the size
property of the Image object. This property returns a tuple containing the width and height of the image. You can use these values as needed.
Python Program
from PIL import Image
# Open the image file
image = Image.open('input_image.jpg')
# Get image_size
image_size = image.size
# Print image size
print(image_size)
Explanation:
- The image is opened using
Image.open()
, which loads the image into theimage
variable. - The
size
property is accessed to retrieve the dimensions of the image. It returns a tuple with the width and height. - The size of the image is printed in the format (width, height), as shown in the output.
input_image.jpg
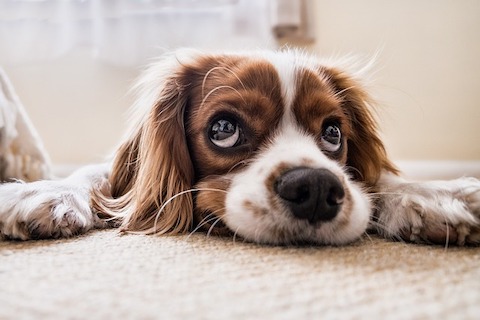
Output
(480, 320)
2. Access width and height from image size using Pillow
In the previous example, the size property returned a tuple of the image's width and height. You can access these values separately by referencing the appropriate index in the tuple.
In the following program, we will retrieve the width and height from the size tuple and print them separately.
Python Program
from PIL import Image
# Open the image file
image = Image.open('input_image.jpg')
# Get image_size
image_size = image.size
# Get width and height
image_width = image_size[0]
image_height = image_size[1]
# Print width and height
print(f"Width : {image_width}")
print(f"Height : {image_height}")
Explanation:
- The
size
property of the image is used to retrieve the dimensions as a tuple. - The width is accessed by referencing the first element of the tuple (index 0), and the height is accessed by referencing the second element (index 1).
- Both width and height are printed separately.
Output
Width : 480
Height : 320
3. Handle images with different orientations
When dealing with images in portrait or landscape orientations, the size property can still be accessed to retrieve the dimensions. The width and height will vary depending on the image's orientation.
For example, in the following program, we will open a portrait image and print its size.
Python Program
from PIL import Image
# Open a portrait image
image = Image.open('portrait_image.jpg')
# Get image_size
image_size = image.size
# Print image size
print(image_size)
Explanation:
- The portrait image is opened using the
Image.open()
method. - The
size
property is accessed to get the image's width and height, which will reflect the portrait orientation. - The size is printed as a tuple, where the first element represents the width and the second element represents the height.
Summary
In this tutorial, we learned how to retrieve the size of an image using the size
property in Python's Pillow library. Additionally, we demonstrated how to access the width and height separately, as well as how the size of portrait and landscape images is handled. These examples will help you effectively work with image dimensions in your projects.