Pillow – Enhance edges of image
We can enhance the edges of an image in Pillow, by calling PIL.Image.filter() function on the given image and passing the predefined filter PIL.ImageFilter.EDGE_ENHANCE.
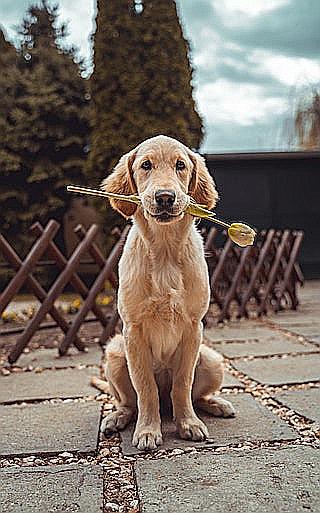
Steps to enhance edges of an image
Follow these steps to enhance the edges of a given image.
- Import Image, and ImageFilter modules from Pillow library.
- Read input image using
Image.open()
function. - Call
filter()
function on the read Image object and passImageFilter.EDGE_ENHANCE
as argument to the function, or if you want more of this, then you may passImageFilter.EDGE_ENHANCE_MORE
. The function returns edge enhanced image as PIL.Image.Image object. - Save the resulting image to required location, or you may process the image further.
Examples
1. Enhance edges using ImageFilter.EDGE_ENHANCE
In the following example, we read an image test_image.jpg
into an Image object. Then, we enhance the edges of this image using Image.filter() function and ImageFilter.EDGE_ENHANCE filter.
We save the resulting image to a file enhanced_image.jpg
.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# Apply edge enhancement filter on the image
enhanced_image = image.filter(ImageFilter.EDGE_ENHANCE)
# Save the enhanced image
enhanced_image.save("enhanced_image.jpg")
Original Image [test_image.jpg]
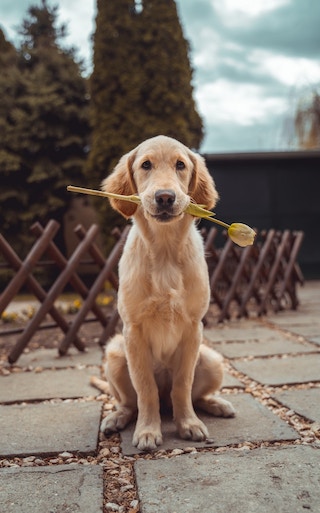
Output Image [enhanced_image.jpg]
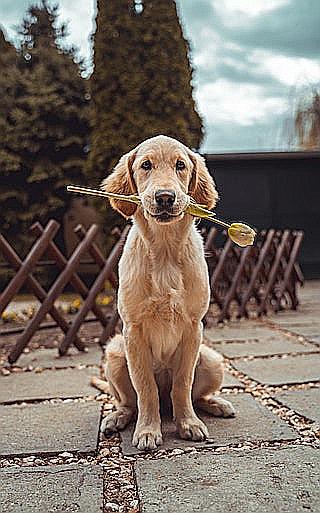
2. Enhance edges using ImageFilter.EDGE_ENHANCE_MORE
In the following example, we read an image test_image.jpg
into an Image object. Then, we enhance the edges of this image using Image.filter() function and ImageFilter.EDGE_ENHANCE_MORE filter.
We save the resulting image to a file enhanced_image.jpg
.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# Apply edge enhancement filter on the image
enhanced_image = image.filter(ImageFilter.EDGE_ENHANCE_MORE)
# Save the enhanced image
enhanced_image.save("enhanced_image.jpg")
Original Image [test_image.jpg]
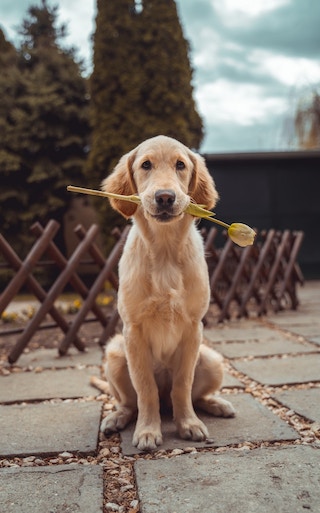
Output Image [enhanced_image.jpg]
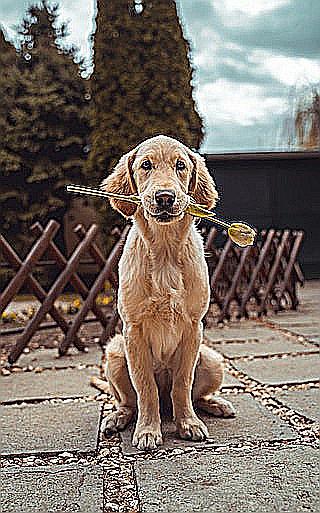
Summary
In this Python Pillow Tutorial, we learned how to enhance the edges of given image using PIL.Image.filter() function, with the help of examples.