How to Invert Colors of Image with Pillow in Python
Pillow - Invert colors of image
To invert colors of an image using Pillow, you can use ImageOps.invert() function of ImageOps module.
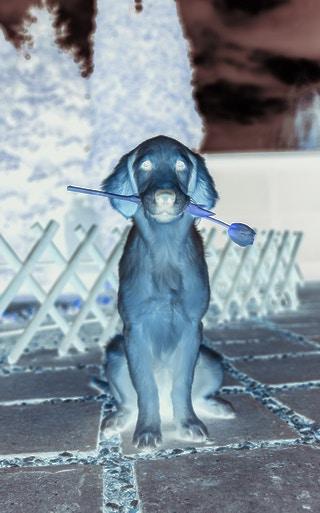
Steps to invert an image
Follow these steps to invert the colors of an image.
- Import Image, and ImageOps modules from Pillow library.
- Read input image using
Image.open()
function. - Pass the read input Image object as argument to the
ImageOps.invert()
function. The function returns inverted image as PIL.Image.Image object. - Save the returned image to required location using
Image.save()
function.
Syntax of invert() function
The syntax of invert() function from PIL.ImageOps module is
PIL.ImageOps.invert(image)
Parameter | Description |
---|---|
image | An PIL.Image.Image object. This is the source or original image which we would like to invert the colors. |
Returns
The function returns a PIL.Image.Image object.
The original image is unchanged.
Examples
1. Invert colors of given image
In the following example, we read an image test_image.jpg
, invert its colors using ImageOps.invert() function, and save the resulting image as inverted_image.jpg
.
Python Program
from PIL import Image, ImageOps
# Open the image
image = Image.open("test_image.jpg")
# Invert the colors of the image
inverted_image = ImageOps.invert(image)
# Save the resulting image
inverted_image.save("inverted_image.jpg")
Original Image [test_image.jpg]
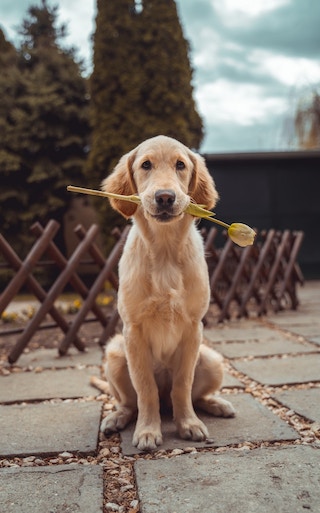
Resulting Image with Inverted Colors [inverted_image.jpg]
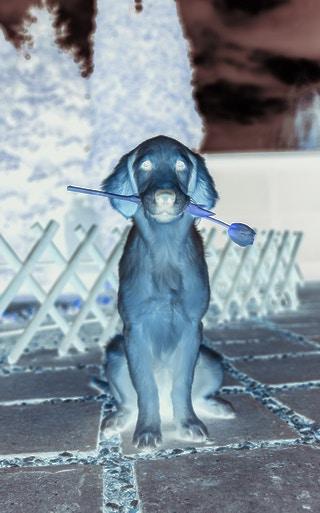
Summary
In this Python Pillow Tutorial, we learned how to invert the colors of a given image using PIL.ImageOps.invert() function, with the help of examples.