How to sharpen an image in Pillow in Python
Pillow - Sharpen image
To sharpen an image using Pillow library, you can use Image.filter() function with ImageFilter.SHARPEN kernel filter or a custom kernel filter.
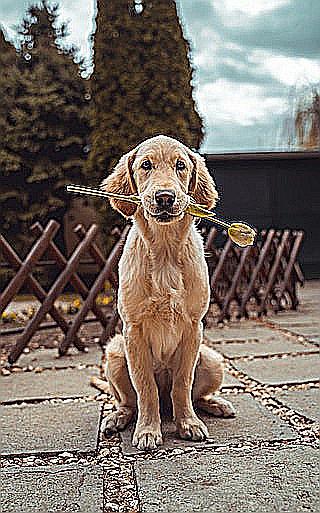
Steps to sharpen an image
Steps to sharpen a given image using builtin filter.
- Import Image, and ImageFilter modules from Pillow library.
- Read input image using
Image.open()
function. - Call
filter()
function on the read Image object and passImageFilter.SHARPEN
as argument to the function, or a custom kernel filter given below. The function returns sharpened image as PIL.Image.Image object. - Save the sharpen image to required location using
Image.save()
function.
Steps to sharpen a given image using custom filter.
- Import Image, and ImageFilter modules from Pillow library.
- Read input image using
Image.open()
function. - Create a sharpening kernel filter using ImageFilter.Kernel.
- Call
filter()
function on the read Image object and pass ImageFilter.Kernel object as argument to the function. The function returns sharpened image as PIL.Image.Image object. - Save the sharpen image to required location using
Image.save()
function.
Examples
1. Sharpen image using predefined filter
In the following example, we read an image test_image.jpg
, sharpen this image using Image.filter() function and predefined ImageFilter, that is ImageFilter.SHARPEN, and save the sharpened image as sharpened_image.jpg
.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# Sharpen the image
sharpened_image = image.filter(ImageFilter.SHARPEN)
# Save the resulting image
sharpened_image.save("sharpened_image.jpg")
Original Image [test_image.jpg]
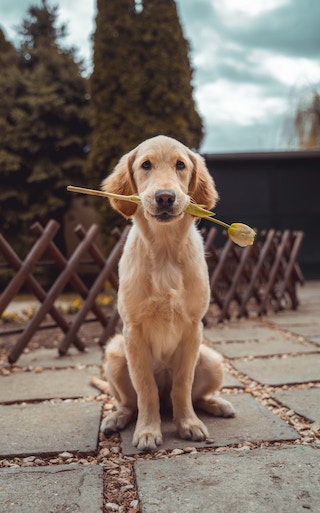
Resulting Image with SHARPEN effect [sharpened_image.jpg]
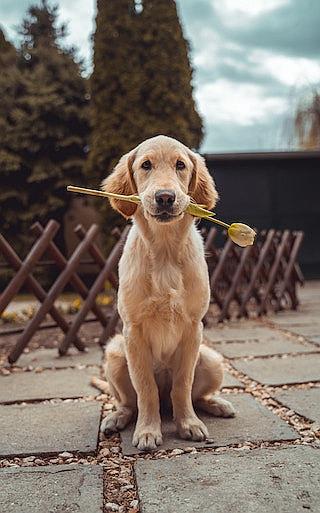
2. Sharpen image using custom kernel filter
Custom Kernel to sharpen an image.
kernel = [-1, -1, -1,
-1, 9, -1,
-1, -1, -1]
We have given more weight to the current pixel, and negative weight to the surrounding pixels. You can change the weights, but you get the idea right! More weight to current pixel, and less/negative weight to neighbouring pixels.
Also, you have specify the scale parameter in filter() function with the sum of values in the kernel matrix.
Please note that the kernel is a one dimensional array. If you are using a two dimensional array or a 2D numpy array, you may have to flatten the kernel.
In the following example, we pass a custom kernel filter to Image.filter() function, and sharpen the image.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# Sharpening kernel
kernel = [-1, -1, -1,
-1, 9, -1,
-1, -1, -1]
kernel_filter = ImageFilter.Kernel((3, 3), kernel, scale=1, offset=0)
# Sharpen the image
sharpened_image = image.filter(kernel_filter)
# Save the resulting image
sharpened_image.save("sharpened_image.jpg")
Sharpened Image [sharpened_image.jpg]
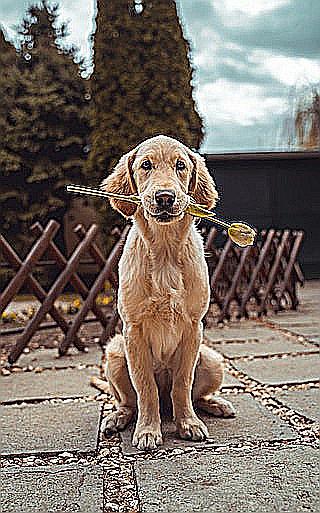
Summary
In this Python Pillow Tutorial, we learned how to sharpen a given image using PIL.Image.filter() function, with the help of examples.