Python Pillow – Open image from URL
To open an image from a URL using the Python Pillow (PIL) library, you can use the requests library to fetch the image from the URL, and then use Pillow to open and manipulate the image.
In the following example, we shall see a step by step process to take a URL for an image, and then open that image using Pillow library.
1. Open image from URL using requests library and Pillow library in Python
In this example, we are given a URL for an image. We have to read the image at the given URL using Python Pillow library.
Steps
- Given image URL in image_url viable.
- Using requests library, call requests.get() method and pass the image url as argument.
response = requests.get(image_url)
- If the response is 200, which means that the request was successful, read the image data from the response object.
image_data = BytesIO(response.content)
- Once we have the image data, call Image.open() and pass the image data as argument. Image.open() method returns an Image object.
image = Image.open(image_data)
- Now, you can do whatever operations or transformations you need on this image object. As this is just a demonstration of reading image from URL, we shall just show the image.
Program
The complete program to read an image from a URL using Pillow is given below.
Python Program
from PIL import Image
import requests
from io import BytesIO
# URL of the image
image_url = 'http://pythonexamples.org/wp-content/uploads/2023/10/image_3.jpg'
# Send a GET request to the URL to fetch the image
response = requests.get(image_url)
# Check if the request was successful (status code 200)
if response.status_code == 200:
# Read the image data from the response
image_data = BytesIO(response.content)
# Open the image using Pillow
image = Image.open(image_data)
# Show the image, or you can write custom code to manipulate the image
image.show()
# Close the image
image.close()
else:
print("Failed to fetch the image. Status code:", response.status_code)
Output
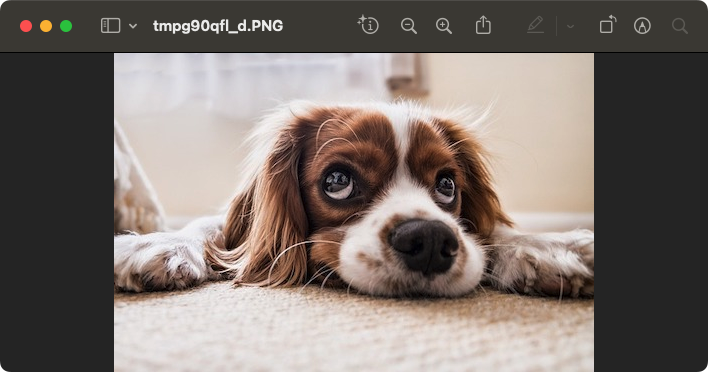
Summary
In this Python Pillow tutorial, we learned how to open an image from URL using Pillow library in Python, with examples. We have taken the help of requests module to make a GET request with the URL, and then retrieved the image data from the response content.