Python - Resize Image using PIL
Python - Resize Image using Pillow Library
To resize an image with Python Pillow, you can use the resize() method of the PIL.Image.Image class. This method allows you to specify the desired image size, the resampling filter to use, and the region of the source image to be considered for resizing.
In this tutorial, we will explore how to resize an image using the Pillow library with practical examples.
Syntax of PIL Image.resize()
The syntax of the resize()
method is as follows:
Image.resize(size, resample=0, box=None)
where
- size is a tuple (width, height) representing the desired size of the output image after resizing.
- resample is an optional parameter specifying the resampling filter to use. You can choose from the following options:
- PIL.Image.NEAREST
- PIL.Image.BOX
- PIL.Image.BILINEAR
- PIL.Image.HAMMING
- PIL.Image.BICUBIC
- PIL.Image.LANCZOS
- box is an optional 4-tuple specifying the region of the source image to be considered for resizing. The values should be in the format (left, upper, right, lower) within the image's width and height. If omitted or set to None, the entire image is used.
By providing appropriate values for the size parameter, you can either enlarge or reduce the size of the image.
Examples
1. Resize an Image with Default Values
In this example, we will resize an image to a fixed size of (200, 200) pixels.
Python Program
from PIL import Image
# Read the image
im = Image.open("sample-image.png")
# Desired image size
size = (200, 200)
# Resize image
out = im.resize(size)
# Save resized image
out.save('resize-output.png')
Explanation:
- The image is opened using
Image.open()
. - The desired output size is specified as
(200, 200)
. - The
resize()
method is used to resize the image, and the resized image is saved to the disk.
Input Image: sample-image.png
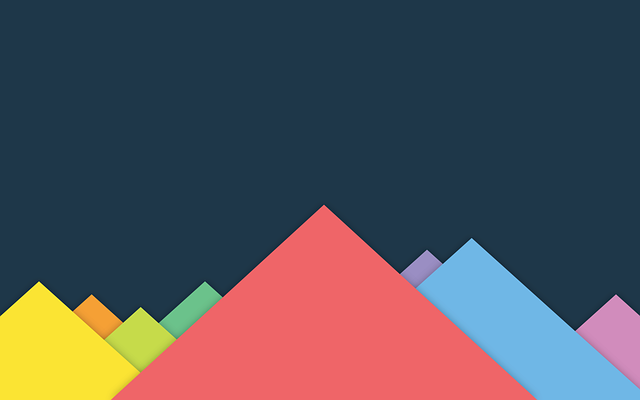
Output Image - resize-output.png
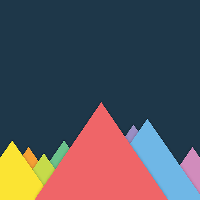
The entire image is resized to the specified dimensions, as no box parameter was provided.
2. Resize Image Using Only a Box Region
In this example, we specify a box region to crop the input image before resizing it.
Python Program
from PIL import Image
# Read the image
im = Image.open("sample-image.png")
# Desired image size
size = (200, 200)
# Define the box region (left, upper, right, lower)
box = (100, 100, 500, 400)
# Resize image using box region
out = im.resize(size, box=box)
# Save resized image
out.save('resize-output.png')
Explanation:
- The image is opened using
Image.open()
. - A box region is defined with coordinates (left, upper, right, lower) to specify which part of the image to resize.
- The
resize()
method is called with the box parameter, and the resized image is saved to the disk.
Output Image - resize-output.png
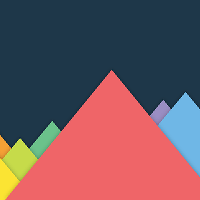
In this case, only the part of the image defined by the box region is resized.
3. Resize an Image with Different Resampling Filters
In this example, we will resize the image using different resampling filters.
Python Program
from PIL import Image
# Read the image
im = Image.open("sample-image.png")
# Desired image size
size = (300, 300)
# Resize image using BICUBIC filter
out = im.resize(size, resample=Image.BICUBIC)
# Save resized image
out.save('resize-output-bicubic.png')
Explanation:
- The image is opened and resized to the size
(300, 300)
using the BICUBIC resampling filter. - The BICUBIC filter is a higher-quality option for resizing images compared to NEAREST or BILINEAR filters.
- The resized image is saved as resize-output-bicubic.png.
Summary
In this tutorial, we covered how to resize images using the resize()
method of the Pillow library. We explored resizing images with and without the box parameter and discussed various resampling filters such as NEAREST, BILINEAR, and BICUBIC. These techniques are useful for resizing images in a variety of scenarios.