Python Pillow – Save image as WebP
You can save an image in WebP format with .webp extension using Pillow library in Python.
WebP format is generally used for the images delivered over web. This is a next generation image format that provides very good image compression with relatively less quality loss. This is to reduce the amount of data transferred over the internet.
To save an Image object in WebP format using Pillow, call Image.save() method on the Image object, and specify 'WebP'
for format parameter.
A quick code snippet to save an Image object input_image in WebP format would be as shown in the following.
input_image.save('output_image.webp', format='WebP')
Let us go through some examples.
We shall use the below image as input image in the following examples.
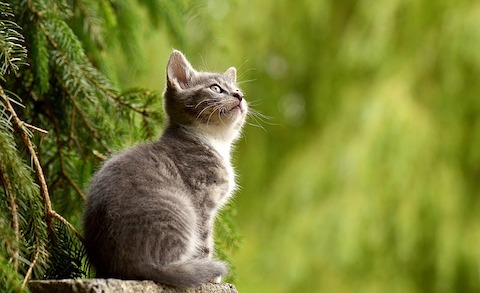
1. Save Image as WebP using Image.save() in Pillow
In this example, we shall read an input image using Image.open() into a variable input_image. Then, we save this image object in input_image as a WebP image using Image.save().
Since this example is for only demonstrating how to save an Image object as a WebP image we are not modifying the input_image. But, in realtime applications, you might be doing additional operations on the input_image Image object. Or, if you are just converting your images into WebP format, then this is a perfect example.
Python Program
from PIL import Image
# Input image file
input_image = Image.open('input_image.jpg')
# Specify the output format as 'WebP'
output_format = 'WebP'
# Save the image as WebP (replace 'output_image.webp' with your desired output filename)
input_image.save('output_image.webp', format=output_format)
# Close the image
input_image.close()
WebP Image
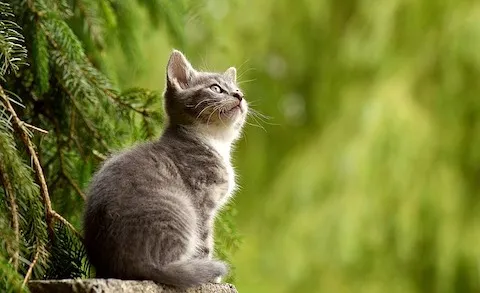
We mentioned that WebP does a pretty good compression to reduce the image size. Let us see how much it saved in memory.
File size in disk
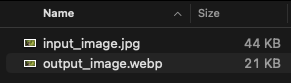
The numbers are out there. The input image is of size 44KB, while the WebP formatted image is just 21KB.
Summary
In this Python Pillow tutorial, we learned how to save an image in WebP format using Pillow library in Python, with examples. We have taken an input image and saved the image in WebP format using Image.save() method from Pillow library.