Python Pillow - Split Image
Python Pillow - Split Image
You can split an image into smaller parts using Pillow library in Python.
Splitting of an image is generally used in preparing of images for training data in machine learning applications for feature extraction.
To split an image into smaller parts or tiles using Python Pillow (PIL), you can use slicing to get the split regions, then crop the regions from original image, and finally save the cropped images as separate images.
Let us go through some examples of splitting an image into tiles.
We shall use the following image as input image in the following examples.
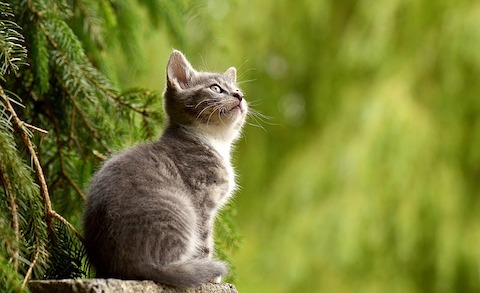
1. Split image into tiles using slicing and Image.crop() in Pillow
We are given an image input_image.jpg
. We have to split this image into tiles, where tile is of size 100 x 100
.
Steps
- Open original image using Image.open().
original_image = Image.open('input_image.jpg')
- Take tile width and tile height.
tile_width = 100
tile_height = 100
- We use nested For loop to iterate through the image and extract tiles based on the specified dimensions. The outer For loop iterates over the horizontal axis from left to right in steps of tile width. The inner For loop iterates over the vertical axis from top to bottom in steps of tile height.
for x in range(0, image_width, tile_width):
for y in range(0, image_height, tile_height):
- Inside the nested For loop, get the crop region of the tile, and crop the original image to get the tile. Then save the tile to local storage.
Program
The complete program to split image into tiles using Pillow is given below.
Python Program
from PIL import Image
# Open the image
input_image = Image.open('input_image.jpg')
# The size of each tile (in pixels)
tile_width = 100
tile_height = 100
# Get the dimensions of the input image
image_width, image_height = input_image.size
# Loop through the image and split it into tiles
for x in range(0, image_width, tile_width):
for y in range(0, image_height, tile_height):
left = x
upper = y
right = x + tile_width
lower = y + tile_height
# Crop the tile from the original image
tile = input_image.crop((left, upper, right, lower))
# Save the tile as a separate image
tile.save(f'tile_{x}_{y}.jpg')
# Close the input image
input_image.close()
Tiles
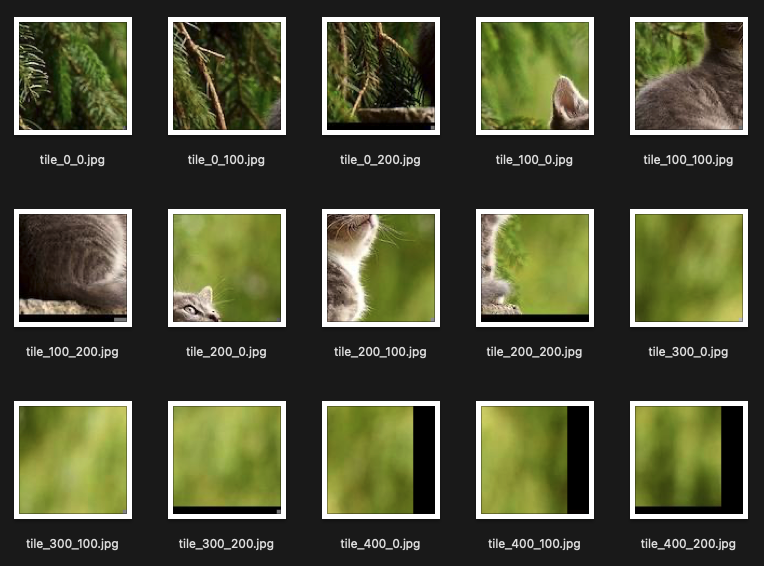
Let us change the tile dimensions to 150 x 150
and split the image.
Python Program
from PIL import Image
# Open the image
input_image = Image.open('input_image.jpg')
# The size of each tile (in pixels)
tile_width = 150
tile_height = 150
# Get the dimensions of the input image
image_width, image_height = input_image.size
# Loop through the image and split it into tiles
for x in range(0, image_width, tile_width):
for y in range(0, image_height, tile_height):
left = x
upper = y
right = x + tile_width
lower = y + tile_height
# Crop the tile from the original image
tile = input_image.crop((left, upper, right, lower))
# Save the tile as a separate image
tile.save(f'tile_{x}_{y}.jpg')
# Close the input image
input_image.close()
Tiles
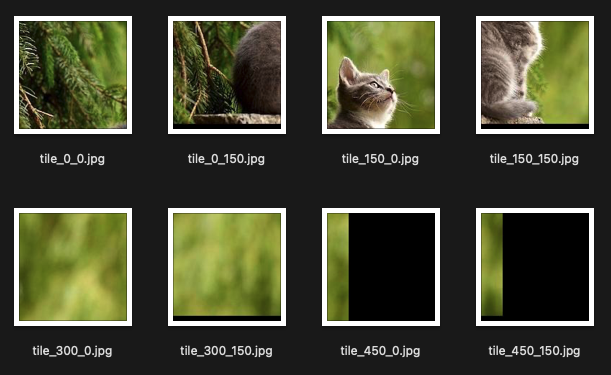
Summary
In this Python Pillow tutorial, we learned how to split an image into tiles using Pillow library in Python, with examples. We have taken an input image and split the image into tiles of dimension 100x100
, and also into tiles of dimension 150x150
. You can specify whatever tile dimension you want, and also different values for width and height.