Contents
Python Pillow – Stitch Images
You can stitch two or more images into a single image using Pillow library in Python.
To stitch or combine multiple images together using Python Pillow (PIL), you can create a new image and paste the individual images onto it.
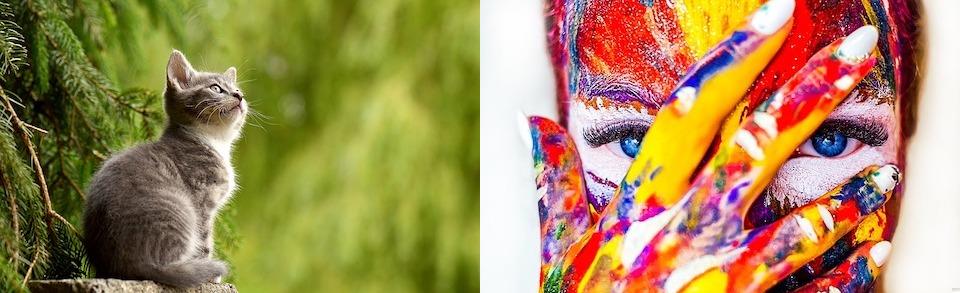
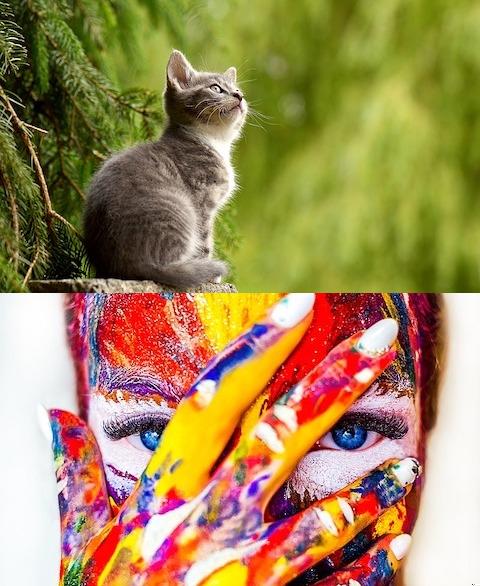
Let us go through some examples of stitching two or more images into a single image.
1. Stitch two images horizontally using Image.paste() in Pillow
We are given two images: image_1.jpg and image_2.jpg. We have to stitch these images horizontally into a single image.
Steps
- Open the input images using Image.open().
image_1 = Image.open('image_1.jpg')
image_2 = Image.open('image_2.jpg')
- Compute the width and height of resulting stitched image. Since we are stitching the images horizontally, width is the sum of the widths of input images, and height is the maximum of the input images.
width = image_1.width + image_2.width
height = max(image_1.height, image_2.height)
- Create a new blank image with the calculated dimensions in the above step.
stitched_image = Image.new('RGB', (width, height))
- Paste the input images onto the stitched image using Image.paste().
stitched_image.paste(image_1, (0, 0))
stitched_image.paste(image_2, (image_1.width, 0))
- Save the stitched image to local storage.
stitched_image.save('stitched_image.jpg')
Program
The complete program to stitch two images horizontally into a single image using Python Pillow library is given below.
Python Program
from PIL import Image
# Open the input images
image_1 = Image.open('image_1.jpg')
image_2 = Image.open('image_2.jpg')
# Determine the width and height of the stitched image
width = image_1.width + image_2.width
height = max(image_1.height, image_2.height)
# Create a new blank image with the calculated dimensions
stitched_image = Image.new('RGB', (width, height))
# Paste the individual images onto the stitched image
stitched_image.paste(image_1, (0, 0))
stitched_image.paste(image_2, (image_1.width, 0))
# Save the stitched image
stitched_image.save('stitched_image.jpg')
# Close all images
image_1.close()
image_2.close()
Copyimage_1.jpg
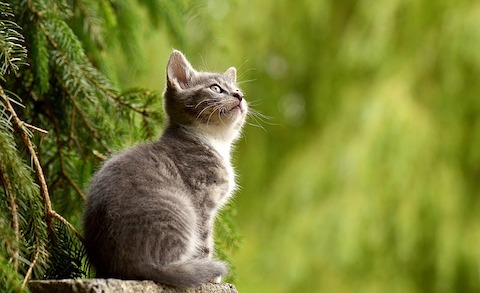
image_2.jpg
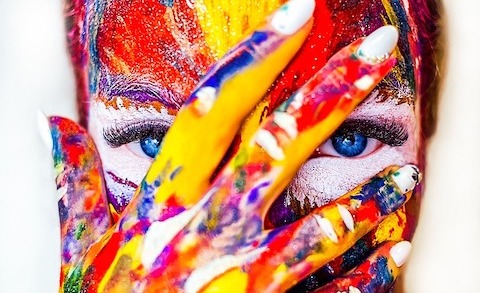
stitched_image.jpg
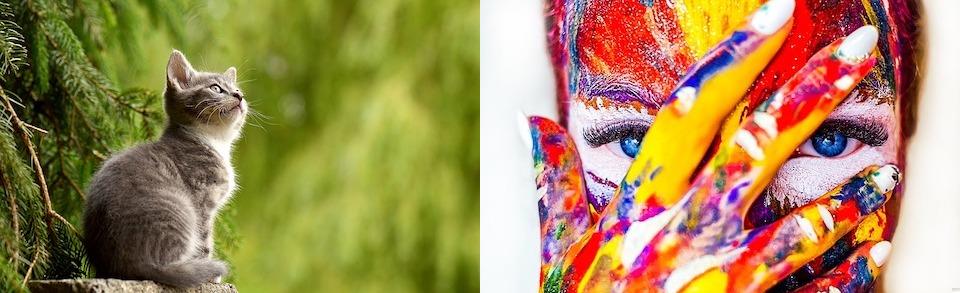
2. Stitch two images vertically using Image.paste() in Pillow
We are given two images: image_1.jpg and image_2.jpg. We have to stitch these images vertically into a single image.
Steps
- Open the input images using Image.open().
image_1 = Image.open('image_1.jpg')
image_2 = Image.open('image_2.jpg')
- Compute the width and height of resulting stitched image. Since we are stitching the images vertically, width is the maximum of the widths of two input images, and height is the sum of the two input images.
width = max(image_1.width, image_2.width)
height = image_1.height + image_2.height
- Create a new blank image with the calculated dimensions in the above step.
stitched_image = Image.new('RGB', (width, height))
- Paste the input images onto the stitched image using Image.paste().
stitched_image.paste(image_1, (0, 0))
stitched_image.paste(image_2, (0, image_1.height))
- Save the stitched image to local storage.
stitched_image.save('stitched_image.jpg')
Program
The complete program to stitch two images vertically into a single image using Python Pillow library is given below.
Python Program
from PIL import Image
# Open the input images
image_1 = Image.open('image_1.jpg')
image_2 = Image.open('image_2.jpg')
# Determine the width and height of the stitched image
width = max(image_1.width, image_2.width)
height = image_1.height + image_2.height
# Create a new blank image with the calculated dimensions
stitched_image = Image.new('RGB', (width, height))
# Paste the individual images onto the stitched image
stitched_image.paste(image_1, (0, 0))
stitched_image.paste(image_2, (0, image_1.height))
# Save the stitched image
stitched_image.save('stitched_image.jpg')
# Close all images
image_1.close()
image_2.close()
Copyimage_1.jpg
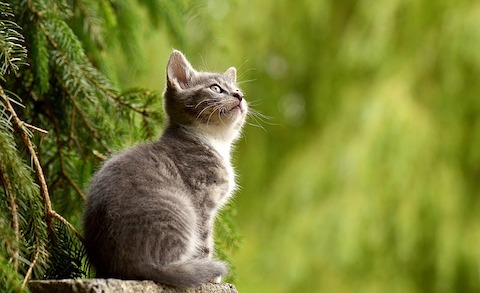
image_2.jpg
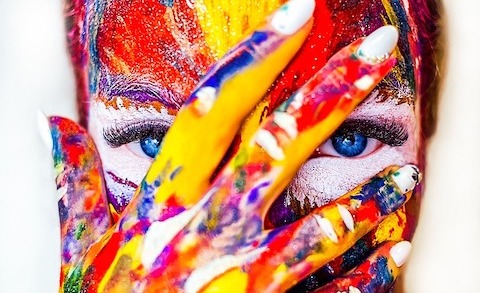
stitched_image.jpg
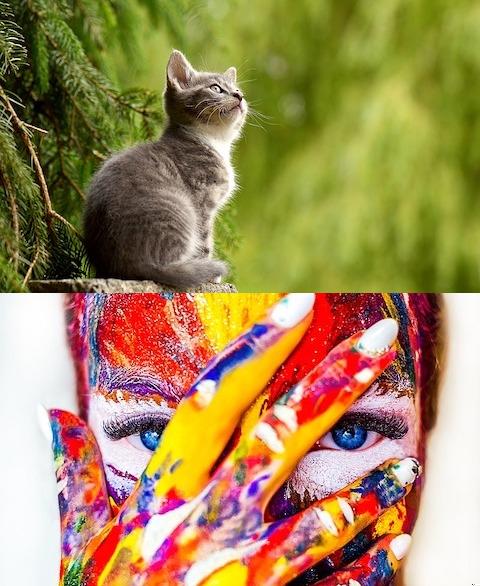
3. Stitch multiple images horizontally using Image.paste() in Pillow
We are given three images: image_1.jpg, image_2.jpg, and image_3.jpg. We have to stitch these images horizontally into a single image.
The following steps hold for stitching any number of images horizontally.
Steps
- Given paths to input images in a list.
images_paths = ['image_1.jpg', 'image_2.jpg', 'image_3.jpg']
- Open the input images using Image.open() and store all of them in a list.
images_list = [Image.open(image) for image in images_paths]
- Compute the width and height of resulting stitched image. Since we are stitching the images horizontally, width is the sum of the widths of all the input images, and height is the maximum of all the input images.
width = sum([image.width for image in images_list])
height = max([image.height for image in images_list])
- Create a new blank image with the calculated dimensions in the above step.
stitched_image = Image.new('RGB', (width, height))
- Paste the input images one by one onto the stitched image using Image.paste() from left to right.
cummulative_width = 0
for image in images_list:
stitched_image.paste(image, (cummulative_width, 0))
cummulative_width += image.width
- Save the stitched image to local storage.
stitched_image.save('stitched_image.jpg')
Program
The complete program to stitch multiple images horizontally into a single image using Python Pillow library is given below.
Python Program
from PIL import Image
# List of images
images_paths = ['image_1.jpg', 'image_2.jpg', 'image_3.jpg']
# Open the input images
images_list = [Image.open(image) for image in images_paths]
# Determine the width and height of the stitched image
width = sum([image.width for image in images_list])
height = max([image.height for image in images_list])
# Create a new blank image with the calculated dimensions
stitched_image = Image.new('RGB', (width, height))
# Paste the individual images onto the stitched image
cummulative_width = 0
for image in images_list:
stitched_image.paste(image, (cummulative_width, 0))
cummulative_width += image.width
# Save the stitched image
stitched_image.save('stitched_image.jpg')
# Close all images
for image in images_list:
image.close()
Copyimage_1.jpg
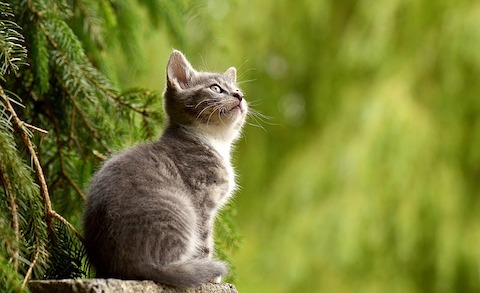
image_2.jpg
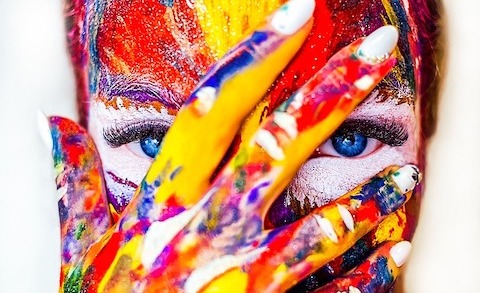
image_3.jpg
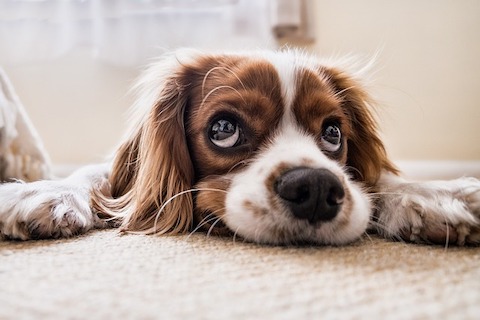
stitched_image.jpg
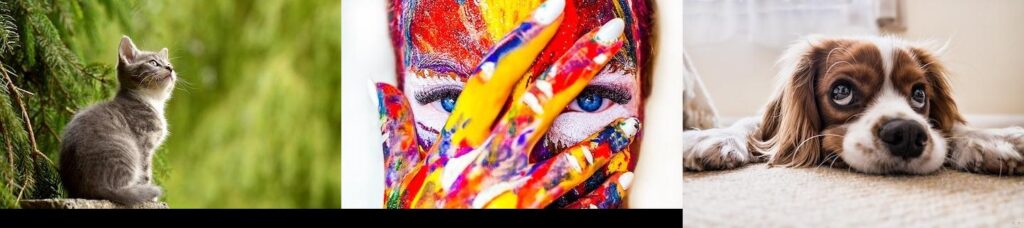
4. Stitch multiple images vertically using Image.paste() in Pillow
We are given three images: image_1.jpg, image_2.jpg, and image_3.jpg. We have to stitch these images vertically into a single image.
The following steps hold for stitching any number of images vertically.
Steps
- Given paths to input images in a list.
images_paths = ['image_1.jpg', 'image_2.jpg', 'image_3.jpg']
- Open the input images using Image.open() and store all of them in a list.
images_list = [Image.open(image) for image in images_paths]
- Compute the width and height of resulting stitched image. Since we are stitching the images vertically, width is the maximum of the widths of all the input images, and height is the sum of all the input images.
width = max([image.width for image in images_list])
height = sum([image.height for image in images_list])
- Create a new blank image with the calculated dimensions in the above step.
stitched_image = Image.new('RGB', (width, height))
- Paste the input images one by one onto the stitched image using Image.paste() from top to bottom.
cummulative_height = 0
for image in images_list:
stitched_image.paste(image, (0, cummulative_height))
cummulative_height += image.height
- Save the stitched image to local storage.
stitched_image.save('stitched_image.jpg')
Program
The complete program to stitch multiple images vertically into a single image using Python Pillow library is given below.
Python Program
from PIL import Image
# List of images
images_paths = ['image_1.jpg', 'image_2.jpg', 'image_3.jpg']
# Open the input images
images_list = [Image.open(image) for image in images_paths]
# Determine the width and height of the stitched image
width = max([image.width for image in images_list])
height = sum([image.height for image in images_list])
# Create a new blank image with the calculated dimensions
stitched_image = Image.new('RGB', (width, height))
# Paste the individual images onto the stitched image
cummulative_height = 0
for image in images_list:
stitched_image.paste(image, (0, cummulative_height))
cummulative_height += image.height
# Save the stitched image
stitched_image.save('stitched_image.jpg')
# Close all images
for image in images_list:
image.close()
Copyimage_1.jpg
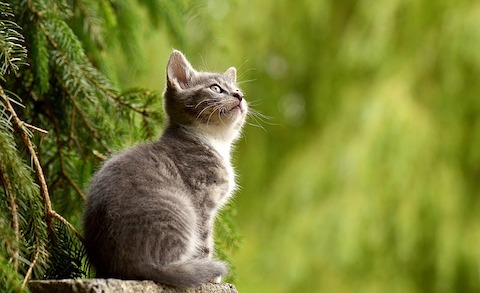
image_2.jpg
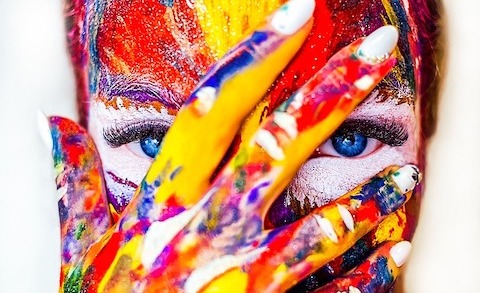
image_3.jpg
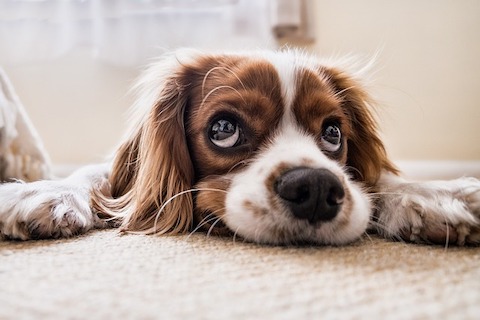
stitched_image.jpg
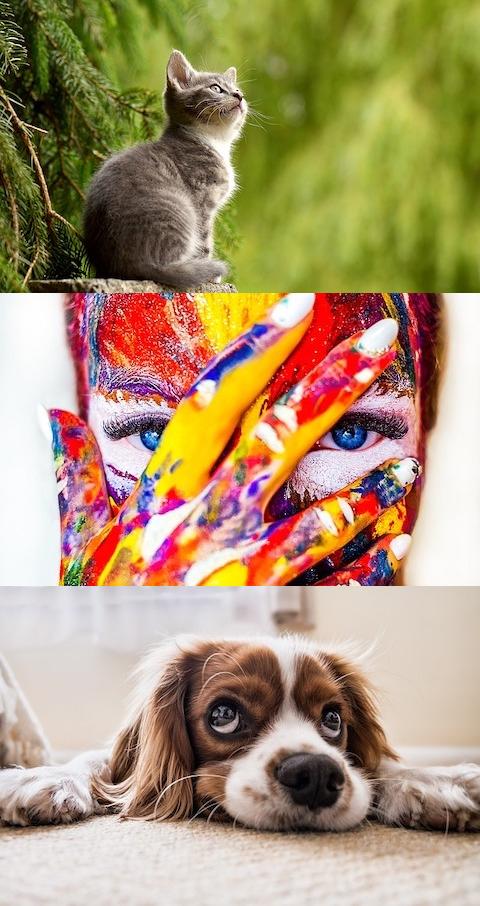
What are the uses of Stitching Images?
Stitching images is generally used in the following scenarios.
Panoramic Photography
Creating panoramic images by stitching together multiple photos taken from the same location but with different camera positions or angles. This is often used in landscape and architectural photography to capture a wider field of view.
360-Degree Virtual Tours
Building immersive 360-degree virtual tours of locations, real estate properties, or attractions by stitching together a series of images taken from different viewpoints.
Medical Imaging
In medical imaging, stitching together multiple medical images (e.g., X-rays, MRIs, CT scans) to create a comprehensive view of a patient’s anatomy or pathology.
GIS and Mapping
Stitching aerial or satellite images together to create high-resolution maps for geographic information systems (GIS) and cartography.
Document Scanning
Scanning large documents or drawings in smaller segments and then stitching them together to create a complete digital copy.
Astronomy and Astrophotography
Combining multiple images of the night sky to create a larger and more detailed view of celestial objects such as stars, galaxies, and nebulae.
Summary
In this Python Pillow tutorial, we learned how to stitch two or more images into a single image using Pillow library in Python, with examples.