Python Pillow - Write Text on Image
Python Pillow - Write Text on Image
You can write text on an image using Pillow library in Python.
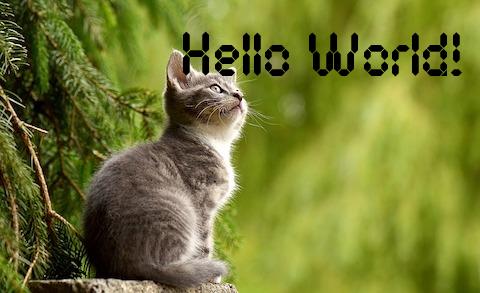
We can control the many parameters while writing text on an image file. Some of the them are given below.
- Color of the text
- Position of the text on image
- Font of the text
- Size of the text
- Text spacing
- Alignment of the text
- Direction of the text
- Stroke width of the text
Now, let us go through some examples, that demonstrate how we can write text on an image using some of the above properties.
We shall use the following image as input image to write text on.
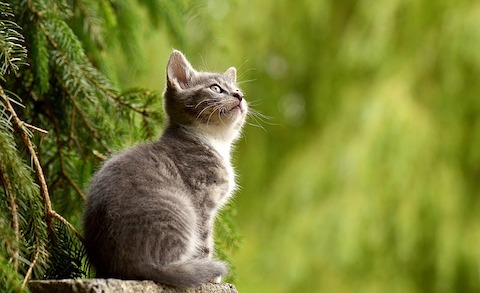
1. Basic example to write text on image using Pillow in Python
In this example, we shall write a simple Python program that uses Pillow library to write some text on an image.
There are three mandatory things that we need to write some text on an image.
- The image itself.
- The text content as a string.
- The position of the text on the image as a tuple of (float, float).
The input image that we would like to use is already mentioned above (a cat image). At the local store, we named it as input_image.jpg.
Hello World is the text that we are going to write on the image.
(180, 20) is the position of the text on the image. 180 is the position of the text from the left of image, and 20 is the position of the text from the top of image.
Now, let us write the program. The comments in the program, explain what is happening for each step.
Python Program
from PIL import Image, ImageDraw
# Open an image file (you can replace 'input_image.jpg' with your image file)
input_image = Image.open('input_image.jpg')
# Create a drawing object
draw = ImageDraw.Draw(input_image)
# Specify the position where you want to write the text (x, y)
text_position = (180, 20)
# Text to write on the image
text = "Hello World!"
# Write the text on the image
draw.text(text_position, text)
# Save the modified image (you can replace 'output_image.jpg' with your desired output filename)
input_image.save('output_image.jpg')
# Close the image
input_image.close()
Output Image
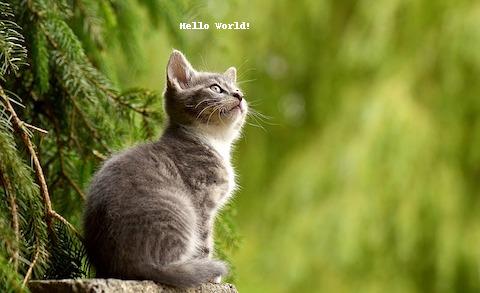
The pillow library has written the text with default font values: font file, font size, font color, etc.
Let us see how to change these text properties.
2. Specific Font File for writing text on image using Pillow in Python
We can specify required font for the text on image using ImageFont.truetype().
Download the font file with .ttf file extension into your Python project.
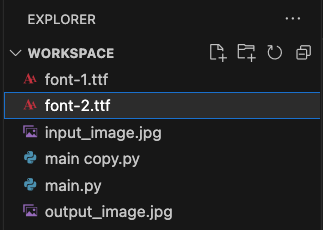
Use it to create a font object using ImageFont.truetype().
font = ImageFont.truetype(font='font-2.ttf')
Then pass the returned font object for the font parameter to draw.text() as shown in the following program.
Python Program
from PIL import Image, ImageDraw
# Open an image file (you can replace 'input_image.jpg' with your image file)
input_image = Image.open('input_image.jpg')
# Create a drawing object
draw = ImageDraw.Draw(input_image)
# Specify the position where you want to write the text (x, y)
text_position = (180, 20)
# Text to write on the image
text = "Hello World!"
# Write the text on the image
draw.text(text_position, text)
# Save the modified image (you can replace 'output_image.jpg' with your desired output filename)
input_image.save('output_image.jpg')
# Close the image
input_image.close()
Output Image
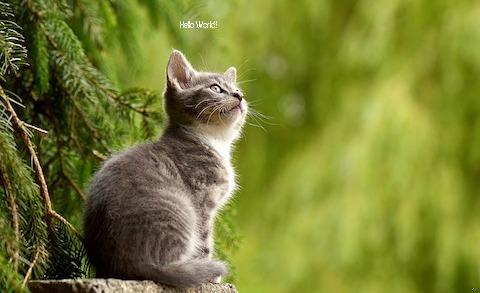
3. Specific Font Size for writing text on image using Pillow in Python
We can specify required font size for the text using ImageFont.truetype(). Specify required size as an integer value for the size parameter.
font = ImageFont.truetype(font='font-2.ttf', size=50)
In the following program, we shall set the font size to 50 for the text we write on the image.
Python Program
from PIL import Image, ImageDraw, ImageFont
# Open an image file (you can replace 'input_image.jpg' with your image file)
input_image = Image.open('input_image.jpg')
# Create a drawing object
draw = ImageDraw.Draw(input_image)
# Choose a font and size
font = ImageFont.truetype(font='font-2.ttf', size=50)
# Specify the position where you want to write the text (x, y)
text_position = (180, 20)
# Text to write on the image
text = "Hello World!"
# Write the text on the image
draw.text(text_position, text, font=font)
# Save the modified image (you can replace 'output_image.jpg' with your desired output filename)
input_image.save('output_image.jpg')
# Close the image
input_image.close()
Output Image
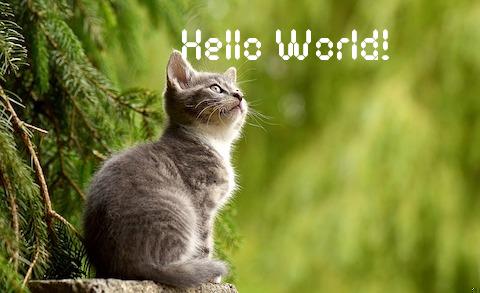
Similarly, if you set font size to 70, you would get the following output image.
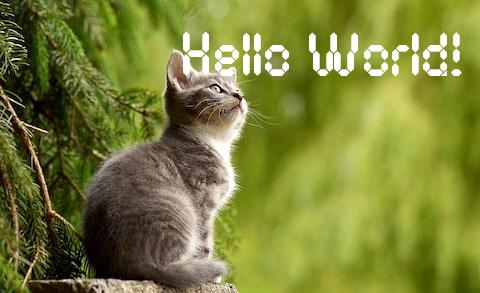
4. Specific Font Color for writing text on image using Pillow in Python
We can specify required color for the text using fill parameter of the ImageDraw.text().
Take a tuple with RGB values, that produces required color, and pass it to the fill parameter.
text_color = (255, 0, 0)
draw.text(text_position, text, fill=text_color, font=font)
(255, 0, 0) produces Red color. (0, 255, 0) produces Green color. (0, 0, 255) produces Blue color.
In the following program, we shall set the font color to red while writing text on the image.
Python Program
from PIL import Image, ImageDraw, ImageFont
# Open an image file (you can replace 'input_image.jpg' with your image file)
input_image = Image.open('input_image.jpg')
# Create a drawing object
draw = ImageDraw.Draw(input_image)
# Choose a font and size
font = ImageFont.truetype(font='font-2.ttf', size=70)
# Specify the position where you want to write the text (x, y)
text_position = (180, 20)
# Text to write on the image
text = "Hello World!"
# Choose text color
text_color = (255, 0, 0) # Red color
# Write the text on the image
draw.text(text_position, text, fill=text_color, font=font)
# Save the modified image (you can replace 'output_image.jpg' with your desired output filename)
input_image.save('output_image.jpg')
# Close the image
input_image.close()
Output Image
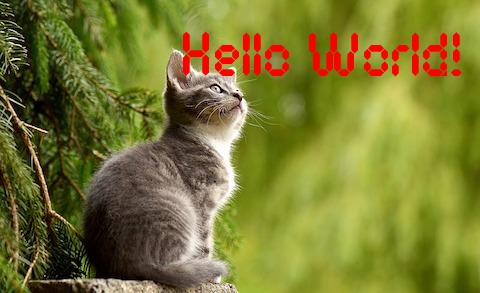
You can try changing the RGB components in the tuple to get whatever color you need. For example, if we use (40, 10, 150) for the fill parameter, then we would get the following output image.
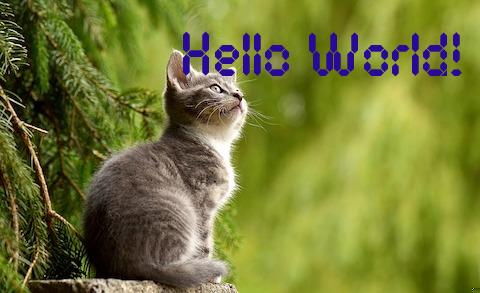
Similarly, for black color, you can use (0, 0, 0) for the fill parameter.
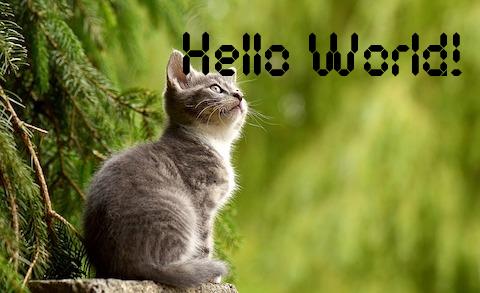
Summary
In this tutorial, we learned how to write text on an image using Pillow library in Python, with examples. We have also seen how to change the font type face, font size, and color, with examples.