Python print() – Print string to console output
Most often, we print data to console output when developing an application. Be it the result we want to display, or intermediate results that we want to check while debugging our program.
To print a string to console output, you can use Python print() built-in function.
In this tutorial, we will learn how to print a string to standard console output with some well detailed examples.
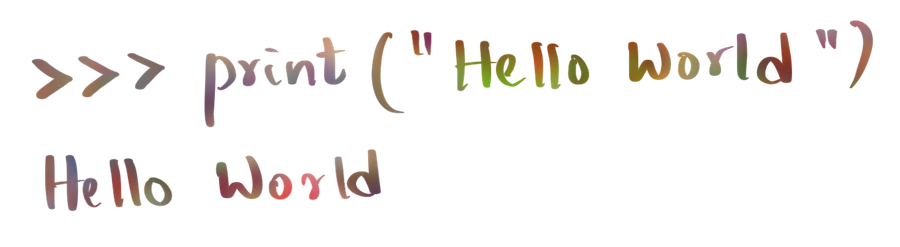
Syntax of print()
The syntax of Python print() function is:
print(*objects, sep=' ', end='\n', file=sys.stdout, flush=False)
where
- objects are comma separated objects that has to be printed to the file.
- sep is the separator used between objects. sep can be a string or a character. By default sep is space.
- end is the character or a string that has to appended after the objects.
- file is the place where our print function has to write the objects. By default it is sys.stdout, in other words system standard output which is console output.
If you would like to override the default values of sep, end, file or flush parameters, you can do so by giving the corresponding argument. We will see more about them in the following examples.
Examples
1. Print a string value to console in Python
The following is a basic example, where we print the string Hello World
to console.
Python Program
print("Hello World")
Output
Hello World
2. Print multiple values to console in Python
You can also specify multiple strings separated by comma to the print function. All those strings will be considered as *objects
parameter.
Python Program
print("Hello", "World")
Output
Hello World
In this example, we have provided two objects to the print() statement. Since the default separator is ‘ ‘ and the end character is new line, we got Hello and World printed with space between them and a new line after the objects.
3. Print values with separator between them in Python
Now, we will provide a separator to the print function.
Python Program
print("Hello", "World", sep='-')
Output
Hello-World
We have overridden the default separator with a character. You can also use a string for a separator.
Python Program
print("Hello", "World", sep=', - ')
Output
Hello, - World
4. Print with different ending character in Python
May be you do not want a new line ending. So, with print()
function’s end
parameter, you can specify your own end
character or string when it prints to the console.
Python Program
print("Hello", "World", end='.')
Output
Hello World.
Summary
In this tutorial of Python Examples, we learned how to print a string to console, how to specify a separator or ending to print() function, with the help of well detailed examples.