Python Text-to-Speech
In this tutorial, you’ll learn how to convert text into speech using Python. We’ll use the gTTS (Google Text-to-Speech) library, which is a simple and effective tool for generating speech from text.
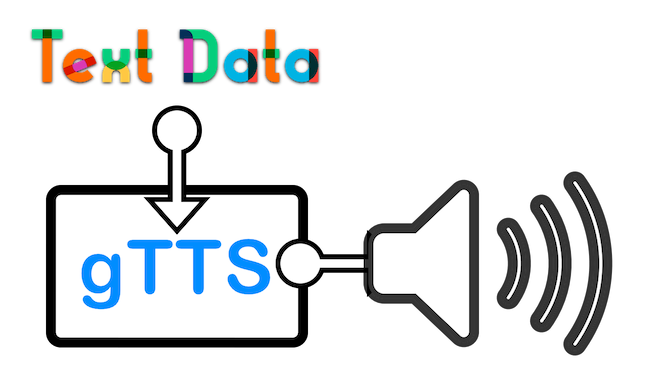
Steps to convert Text to Speech
1. Import gTTS
Import gTTS class from gtts module.
from gtts import gTTS
2. Get input text
Now, we need to take a text, which we would like to convert to speech. Let us take the text in text variable.
text = "Hello World"
You can get this text from user, or hard code a string value yourself, or read text from a file, and then use this text value to convert it into a sound file.
3. Create gTTS object
In this step, we create an instance of gTTS object with the given text passed as argument. Store the instance in a variable, say tts.
tts = gTTS(text)
4. Convert to speech (audio) file
Call save() method on the gTTS instance, and pass the name of the speech file as argument. The save() method creates an audio file with the converted text to speech data in it.
tts.save("output.mp3")
Program
In this program, we will take a sample input text, say “Hello World”, and convert this text into speech using the gTTS library by following the steps.
Python Program
from gtts import gTTS
import os
# Get input text from the user
text = input("Enter the text you want to convert to speech: ")
# Create a gTTS object
tts = gTTS(text)
# Save the generated speech to a file
tts.save("output.mp3")
Output
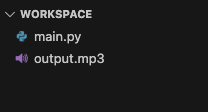
The program created output.mp3 next to our program, just as specified in the code. If you play this audio file, you will hear a voice saying "Hello World"
.
Summary
In this Python Advanced Programs tutorial, we learned how to use the gTTS library in Python to convert text into speech, with well detailed steps, and a program.