How to Create Tensors with Random Values in PyTorch
Create PyTorch Tensor with Ramdom Values
To create a random tensor with specific shape, use torch.rand() function with shape passed as argument to the function. torch.rand() function returns tensor with random values generated in the specified shape.
Example
Following is a simple example, where in we created a tensor of specific size filled with random values.
import torch
#create tensor with random data
rand_tensor = torch.rand((2, 5))
#print tensor
print(rand_tensor)
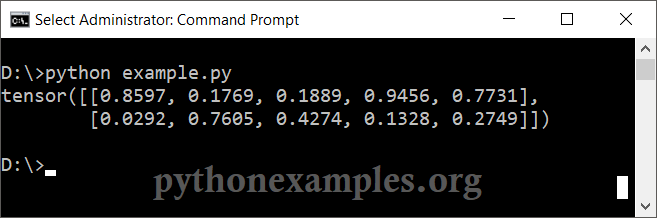
Create PyTorch Tensor with Random Values less than a Specific Maximum Value
By default, pytorch.rand() function generates tensor with floating point values ranging between 0 and 1.
Example
In the following example, we will create a tensor with random values that are less than 8.
import torch
#create tensor with random data, and multiply with a scalar
rand_tensor = 8*torch.rand((2, 5))
#print tensor
print(rand_tensor)
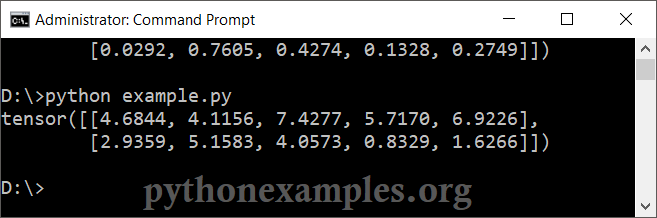
Create Tensor with Random Values from a Range
You can also create a PyTorch Tensor with random values belonging to a specific range (min, max).
Example
In the following example, we have taken a range of (4, 8) and created a tensor, with random values being picked from the range (4, 8).
import torch
#range
max = 8
min = 4
#create tensor with random values in range (min, max)
rand_tensor = (max-min)*torch.rand((2, 5)) + min
#print tensor
print(rand_tensor)
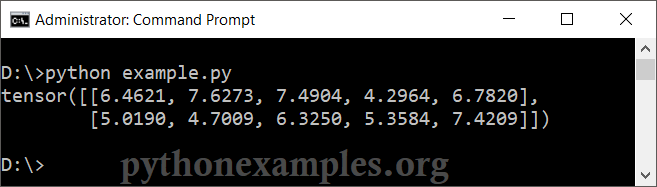