Python – Filter List
In this tutorial, you will learn what filtering a list means, how can we filter a list, different ways to filter a list, with steps and detailed examples.
What does filtering a list mean in Python
It means that you are trying to get the items from the list, that satisfy a given condition or function.
How do we filter a list in Python
There are many ways in which you can filter a list in Python. We will discuss three ways in this tutorial.
- Filter list using For loop and if-statement
- Filter list using List Comprehension
- Filter list using filter() built-in function
1. Filter list using For loop and If statement in Python
This first way is to iterate over the list, and then check the condition for each item in the list using an if-statement.
For example, consider a scenario where we are given a list of numbers, and we need to filter even numbers.
Let us use For loop and if-statement to filter even numbers in the list.
Python Program
nums = [5, 8, 2, 3, 9, 44, 21]
# Take an empty list to store the filtered items
filtered_nums = []
# For each item in the list
for n in nums:
# Check if the item satisfies the filter condition
if n % 2 == 0:
# If the item satisfies the conditon, then add it to the filtered list
filtered_nums.append(n)
# Print the filtered list
print(f"Filtered list : {filtered_nums}")
Output
Filtered list : [8, 2, 44]
The comments in the program should suffice to explain what we are doing. Anyways, let us see once again what we did in the program.
- Take the given list of numbers in nums.
- Take an empty list filtered_nums to store the filtered items of the given list.
- Use a For loop to iterate over the items in the list nums.
- Use an if-statement to check if the item is an even number. If True, then append the item to the filtered list filtered_nums using List append() method.
- All the filtered items are collected in the filtered list filtered_nums. You may print it to output.
This way of filtering a list is very basic and simple. Because it uses a looping statement and a conditional statement, to filter the given list. No advanced concepts.
2. Filter list using List Comprehension in Python
Using List Comprehension is the second way to filter a list in Python.
We shall consider the scenario of filtering even numbers in the given list, that we used in the previous example.
We have to use list comprehension containing if condition to filter the list.
Let us write the program to filter even numbers in the list using list comprehension.
Python Program
nums = [5, 8, 2, 3, 9, 44, 21]
# Filter even numbers in the nums list
filtered_nums = [n for n in nums if n % 2 == 0]
# Print the filtered list
print(f"Filtered list : {filtered_nums}")
Output
Filtered list : [8, 2, 44]
This code looks like a concise version of the previous example where we used For loop and If-statement. Just compare the code in the previous example, with that of in this example.
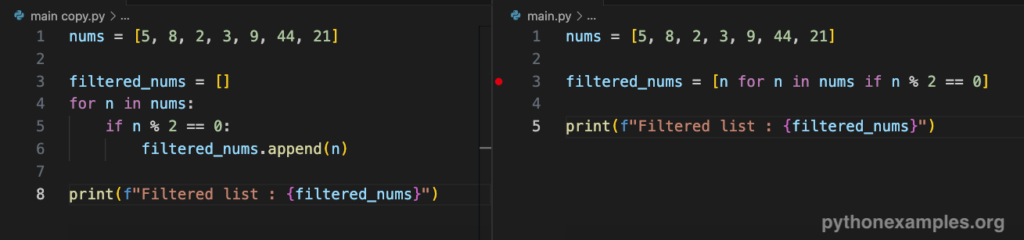
This way of filtering a list makes the program concise. Because, if you have an idea how List comprehension works, the code to filter a list becomes more readable to you in a single statement.
3. Filter list using filter() built-in function in Python
Coming to the third way of filtering a list in Python, you can use filter() built-in function.
The syntax of filter() built-in function is given below.
filter(function, iterable)
The function parameter takes a function as argument. The function takes the list item as argument, and returns a boolean value. If the returned value is True, then the item is included in the filtered list, or if the returned value is False, then the item is not included in the filtered list.
We shall consider the scenario of filtering even numbers in the given list, that we used in the previous examples.
For the first argument of filter(), we have to define a function that takes a number as argument and returns True if the argument is an even number, or returns False if the argument is an odd number. Let us name this function as is_even().
def is_even(n):
return n % 2 == 0
And for the second argument to filter(), we have to pass our given list of numbers.
The filter() function, with the given two arguments, returns a filter object containing only even numbers. Please note that filter() function returns a filter object not the list. But we can convert this filter object to a list using list(), which we shall see in the program.
Let us write the program to filter even numbers in the list using filter() function.
Python Program
nums = [5, 8, 2, 3, 9, 44, 21]
def is_even(n):
return n % 2 == 0
# Filter even numbers in the nums list
filtered_nums = filter(is_even, nums)
# Print the filtered list
print(f"Filtered list : {list(filtered_nums)}")
Output
Filtered list : [8, 2, 44]
We have successfully filtered a given list using filter() function.
This way of filtering a list gives modularity to the program. Because, we have a custom function that defines how to filter items in a list. Also, for the filtering function, you can have as much complexity as required.
Summary
Summarizing this tutorial on Python Lists, we have gone through three different ways to filter a list in Python. You may choose whichever way that suits you based on simplicity, concise code, or modularity.