Python – Find Index of Element in a List
To find the index of a specific element in a given list in Python, you can call the list.index() method on the list object and pass the specific element as argument.
index = mylist.index(element)
The list.index() method returns an integer that represents the index of first occurrence of specified element in the List.
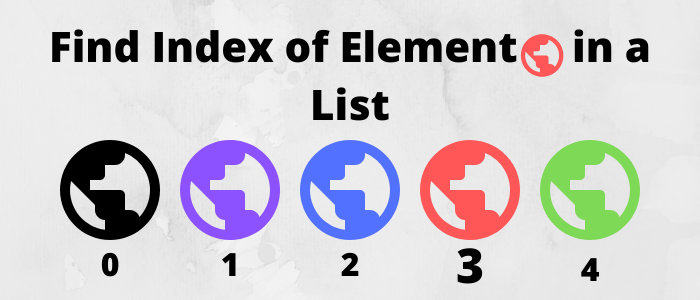
You can also provide start and end positions in the List, to specify the bounds where the search has to happen in the list.
Syntax of list.index()
The syntax of index() function to find the index of element x
in the list mylist
is
index = mylist.index(x, [start[,end]])
start
parameter is optional. If you provide a value for start
, then end
is optional.
Examples
In the following examples, we take a list, and an element. We then get the index of the element in the list with following use cases.
- Get index of element in the list.
- Get index of element in the list, where searching for the element must happen from a specific starting position in the list.
- Get index of element in the list, where searching for the element must happen in the [starting position, ending position] bounds.
- The item whose index we are trying to find out, is not in the list.
1. Find index of a specific item in the list
In the following example, we have taken a list with numbers. Using index() method we will find the index of item 8
in the list.
Python Program
#list
mylist = [21, 5, 8, 52, 21, 87]
#search item
item = 8
#find index of item in list
index = mylist.index(item)
#print output
print(f"index of {item} in the list : {index}")
Output
index of 8 in the list : 2
The element is present at 3rd position, so mylist.index()
function returned 2.
2. Find index of a specific item in the list in [start, end] index bounds
In the following example, we have taken a list with numbers.
Using index() method we will find the index of item 8
in the list.
Also, we shall pass start and end indices/positions. index() function considers only those elements in the list starting from start
index, till end
index in mylist
.
Python Program
mylist = [21, 8, 67, 52, 8, 21, 87]
item = 8
start=2
end=7
#search for the item
index = mylist.index(item, start, end)
#print output
print(f"index of {item} in the list : {index}")
Output
index of 8 in the list : 4
Explanation
mylist = [21, 8, 67, 52, 8, 21, 87]
----------------- only this part of the list is considered for searching
^ index() finds the element here
0 1 2 3 4 => 4 is returned by index()
3. Find index of item in list where item has multiple occurrences
A Python List can contain multiple occurrences of an element. If we try to find the index of an element which has multiple occurrences in the list, then index() function returns the index of first occurrence of specified element only.
Python Program
mylist = [21, 5, 8, 52, 21, 87, 52]
item = 52
#search for the item
index = mylist.index(item)
#print output
print(f"index of {item} in the list : {index}")
Output
index of 52 in the list : 3
The element 52
is present two times, but only the index of first occurrence is returned by index() method.
Let us understand how index() method works. The function scans the list from starting. When the item matches the argument, the function returns that index. The later occurrences are ignored.
4. Find index of item in list where item is not in the list
If the element that we are searching in the List is not present, you will get a ValueError
with the message item is not in list
.
In the following program, we have taken a list and shall try to find the index of an element that is not present in the list.
Python Program
mylist = [21, 5, 8, 52, 21, 87, 52]
item = 67
#search for the item/element
index = mylist.index(item)
print('The index of', item, 'in the list is:', index)
Output
Traceback (most recent call last):
File "example.py", line 5, in <module>
index = mylist.index(item)
ValueError: 67 is not in list
As index() can throw ValueError, use Python Try-Except while using index(). In the following example, we shall learn how to use try-except statement to handle this ValueError.
Python Program
mylist = [21, 5, 8, 52, 21, 87, 52]
item = 67
try:
#search for the item
index = mylist.index(item)
print(f"index of {item} in the list is : {index}")
except ValueError:
print("item not present in list")
Output
item not present in list
The item, whose index we are trying to find, is not present in the list. Therefore, mylist.index(item)
throws ValueError. except ValueError:
block catches this Error and the corresponding block is executed. Since we have surrounded the index() function call with try-except, the program is not terminated abruptly, even when there was ValueError thrown.
Instead of using a try-except, we can first check if the element is present in the list or not. We find the index of the element in the list, if only the element is present. We shall demonstrate this using the following program.
Python Program
mylist = [21, 5, 8, 52, 21, 87, 52]
item = 67
if item in mylist:
index = mylist.index(item)
print(f"index of {item} in the list is : {index}")
else:
print("item not present in list")
Output
item not present in list
Summary
In this Python Tutorial, we learned how to find the index of an element/item in a list using list.index() method, with the help of well detailed examples.