Accept GET or POST Requests in Flask
Flask - Accept GET or POST Requests
In a flask application, we can specify a route with specific URL to accept GET or POST requests using methods parameter of route() decorator.
The following is a simple code snippet to write route() decorator which specifies that the route can accept GET or POST requests.
@app.route("/page-url", methods=["GET", "POST"])
You may replace page-url
with the required URL.
methods parameter takes an array of allowed HTTP requests. In this case as our URL must accept GET or POST requests, we specify these two request types i.e., "GET"
and "POST"
in the array.
GET request is usually used to get information from the server.
POST request is usually used to post an update or insert a new information (like a record) into the server.
Example
In this example, we build a flask application where we have following project structure.
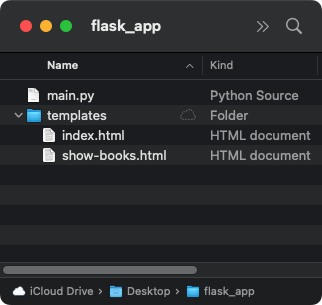
We define a route with the URL "/books"
to accept GET or POST requests, as shown in the following Python program.
If server receives a GET request for the URL, then we render the template show-books.html.
If server receives a POST request for the URL, then we read the parameters, do something with the parameters, and return a JSON.
main.py
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route("/")
def home_page():
return render_template("index.html")
@app.route("/books", methods=["GET", "POST"])
def books():
if request.method == "GET":
return render_template("show-books.html")
elif request.method == "POST":
book_name = request.args["name"]
book_price = request.args["price"]
book_category = request.args["category"]
#you may work with the received data
#like inserting into database, or updating a column for the record
return {"status" : "Book added to library."}
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080, debug=True)
show-books.html
<!DOCTYPE html>
<html>
<body>
<h1>Show Books Page</h1>
<p>Flask application by <a href="/">PythonExamples.org</a>.</p>
<p>This is "show books" page.</p>
</body>
</html>
Run Application
Open terminal or command prompt at the root of the application, and run the Python file main.py.
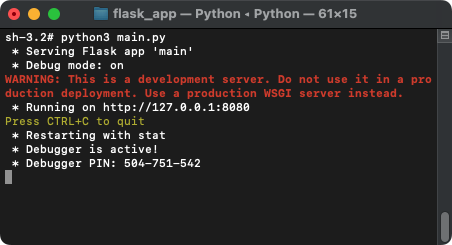
The flask application is up and running at URL http://127.0.0.1:8080/
.
1. Send GET Request
We have used POSTMAN application, and sent a GET request to the URL http://127.0.0.1:8080/books
.
The server responded with "show-books.html"
template as instructed in the Python code, with a status code of 200.
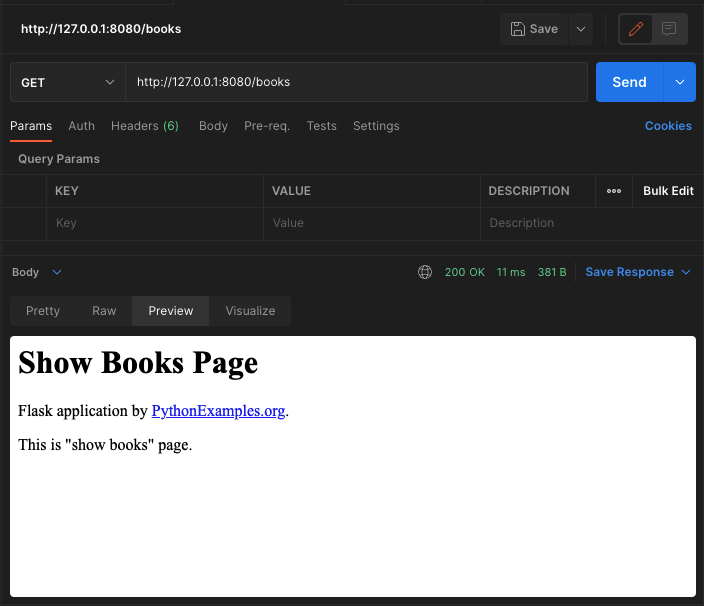
2. Send POST Request
Now, let us try sending a POST request to the same URL, and observe the output.
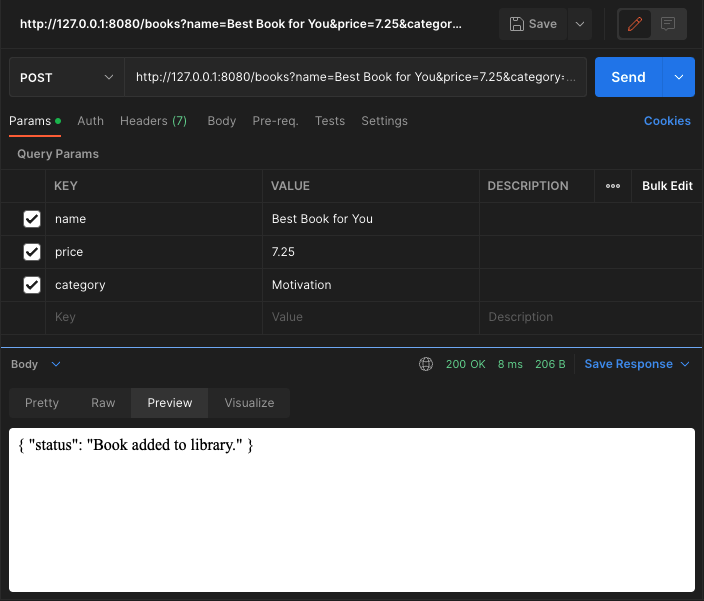
The server responded with JSON string as instructed in the Python code, with a status code of 200.
Project ZIP
Summary
In this Python Flask Tutorial, we learned how to define a route in Flask Application to accept GET or POST requests for a URL.