Flask – Accept only POST Request
In a flask application, we can specify a route with specific URL to accept only POST request using methods parameter of route() decorator.
The following is a simple code snippet to write route() decorator which specifies that the route must accept only POST request.
@app.route("/page-url", methods=["POST"])
You may replace page-url
with the required URL.
methods parameter takes an array of allowed HTTP requests. In this case as our URL must accept only POST request, we specify only one element i.e., "POST"
in the array.
POST request is usually used to post an update or insert a new information (like a record) into the server.
Example
In this example, we build a flask application where we have following project structure.
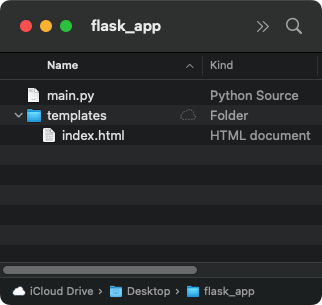
We define a route with the URL "/books"
to accept only POST request, as shown in the following Python program.
main.py
from flask import Flask, request, render_template
app = Flask(__name__)
@app.route("/")
def home_page():
return render_template("index.html")
@app.route("/books", methods=["POST"])
def insert_book():
book_name = request.args["name"]
book_price = request.args["price"]
book_category = request.args["category"]
#you may work with the received data
#like inserting into database, or updating a column for the record
return {"status" : "Book added to library."}
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080, debug=True)
In the route() decorator for “/books” URL, we read the URL parameters using request.args[]. You may use this data to insert the recored, or update a record on the server, what a POST request usually does. Then we return a response in the form of JSON.
Run Application
Open terminal or command prompt at the root of the application, and run the Python file main.py.
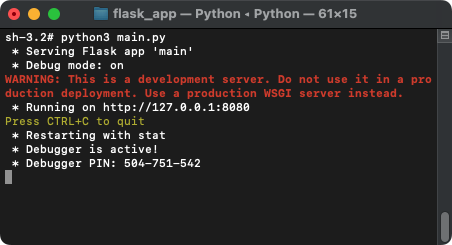
The flask application is up and running at URL http://127.0.0.1:8080/
.
1. Send POST Request
We have used POSTMAN application, and sent a POST request to the URL http://127.0.0.1:8080/books
.
The server responded with JSON string as instructed in the Python code, with a status code of 200.
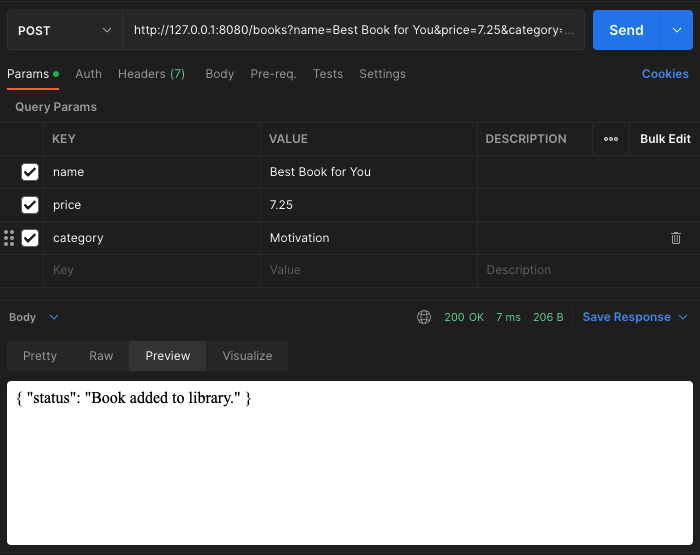
2. Send GET Request
Now, let us try sending a GET request to the same URL, and observe the output.
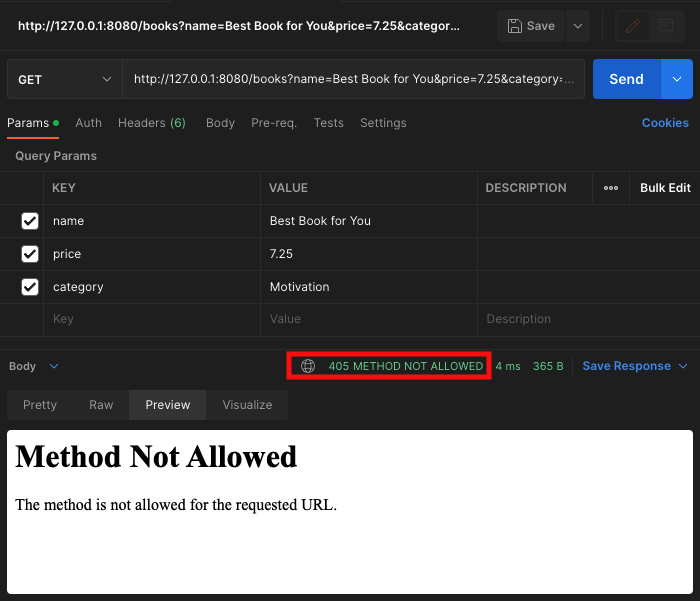
The server responded with 405 METHOD NOT ALLOWED as shown in the above screenshot.
Project ZIP
Summary
In this Python Flask Tutorial, we learned how to define a route in Flask Application to accept only POST requests.