Flask API Example
In this tutorial, we will write a simple flask application to demonstrate how to build an API.
API stands for Application Programming Interface. In simple terms, client gives some input in the request and asks for a response. The server works on the inputs and provides a response to the client.
In the flask API that we are going to build, the server has a set of fruit names and their quantities {(name, quantity), (name, quantity), ...}
. The client makes a request to the server for the quantity given the fruit name. The server upon getting the request, searches for the fruit name and responds with the associated quantity. If the requested name is not present, the API responds with value of zero for the quantity.
The server reads the fruit name from URL query parameter named fruitname
.
main.py
from flask import Flask, request
app = Flask(__name__)
@app.route('/get-quantity', methods=['GET'])
def get_quantity():
#we are taking a hardcoded data, but you may choose a database or another data source
fruits = {('apple', 25), ('banana', 48), ('cherry', 146)}
if request.method == 'GET':
#read URL query parameter
fruitname = request.args['fruitname']
quantity = 0
for item in fruits:
if item[0] == fruitname:
#if a match is found set the quantity and come out of the loop
quantity = item[1]
break
return {fruitname: quantity}
else:
return 'Invalid request.'
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080, debug=True)
Note: This example flask application contains the above main.py
file only, in its root directory.
For the sake of simplicity, we have taken a list of tuples in fruits
variable, and search for the fruit name in this fruits
using a For loop. Instead of this set up, you can either query from a database table or another data source.
Now let us make a request to this application from a browser (which acts as a client).
Open the browser of your choice and hit the following URL.
http://127.0.0.1:8080/get-quantity?fruitname=apple
The server finds the quantity for the requested fruit name and returns the response.
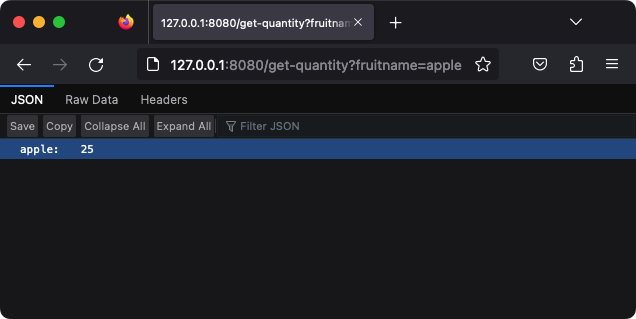
Let us test the API with a fruit name that is not present.
We have sent mango for the fruit name in the request. Since the given fruit name is not present in the data, the server responded with zero for the quantity.
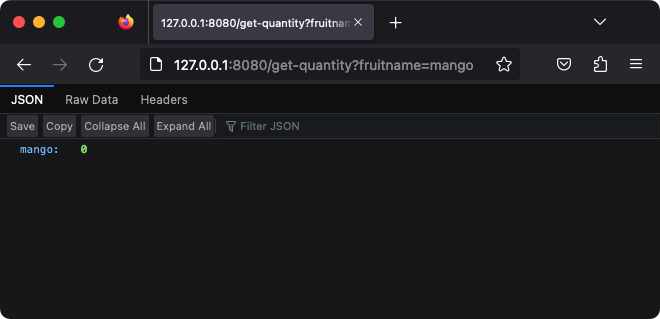
Summary
This is a simple API where the client sends some inputs in the request. Server reads input from the client, makes a search operation in the data, and responds with a value.