Flask – Read Form Data from Request
In this tutorial, we will learn how to read the form data, in flask application, that is entered by the user in HTML form.
In Python flask application, we can access the form data using request object. Request.form
contains the form data from POST or PUT request.
Example
In this example, we will build a flask application where the homepage contains a form to read user ID and password from user.
When user submits the form data in HTML page, we send a POST request to the server via JavaScript.
Inside the flask application, we read the user ID and password from the request object using Request.form
property.
Project Structure
The following is the structure of our flask application.
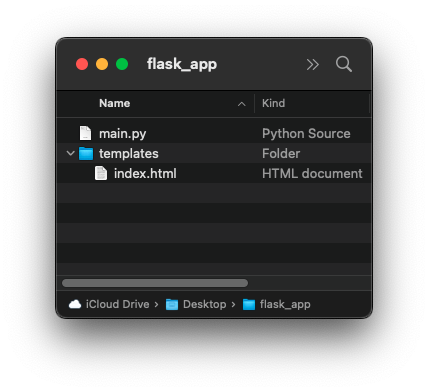
main.py
from flask import Flask, render_template, request
app = Flask(__name__)
@app.route("/")
def index():
return render_template("index.html")
@app.route("/validate-user", methods=["POST"])
def validate_user():
if request.method == "POST":
print(request.form)
print(request.form['userid'])
print(request.form['password'])
return 'Hello ' + request.form['userid']
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080, debug=True)
index.html
<!DOCTYPE html>
<html>
<body>
<form action="/validate-user" method="post">
<label for="userid">User ID</label><br>
<input type="text" name="userid" placeholder="userid@mail.com"><br><br>
<label for="password">Password</label><br>
<input type="password" name="password" placeholder="Hello@123"><br>
<br>
<button type="submit">Login</button>
</form>
</body>
</html>
Terminal
Open terminal at the root of the application, and run the Python file main.py.
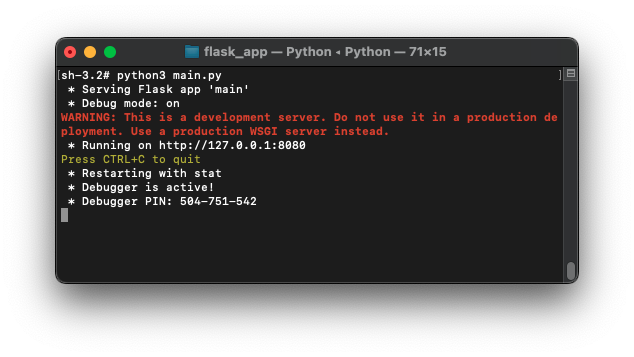
Now, open a browser of your choice and hit the home URL of this flask application: http://127.0.0.1:8080.
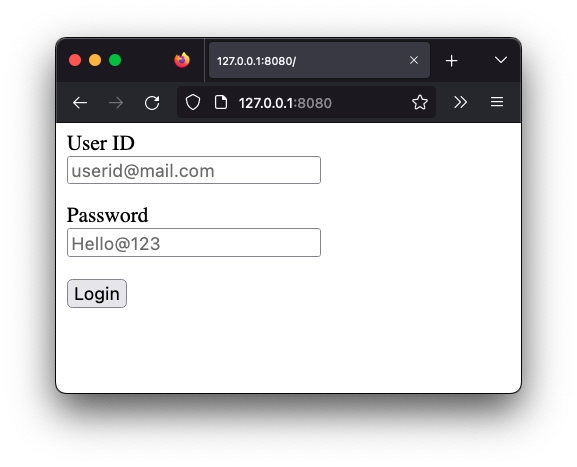
Enter some values for User ID and Password and click on Login button.
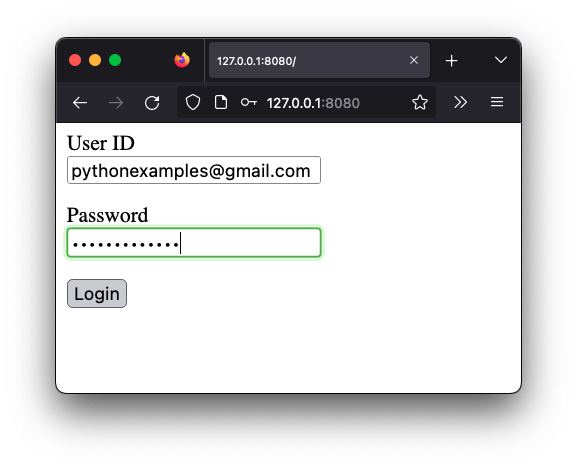
When user clicks on the Login button, a POST request is sent to the URL /validate-user
link, which is mentioned for action
attribute in form element in index.html.
When the request is sent to the server, validate_user()
function is executed. Inside this function, we are printing the userid
and password
to standard output.
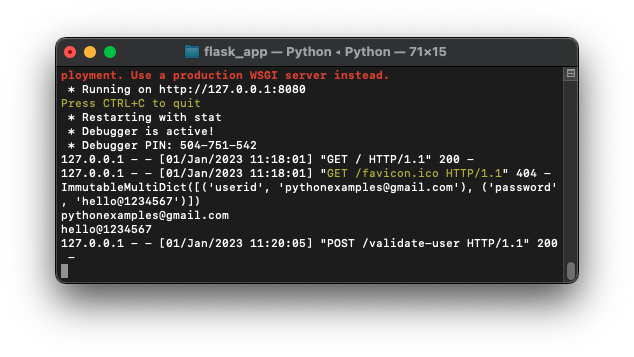
Also, we are returning a string value of 'Hello userid'
in the response, where userid is the value entered by user in the form.
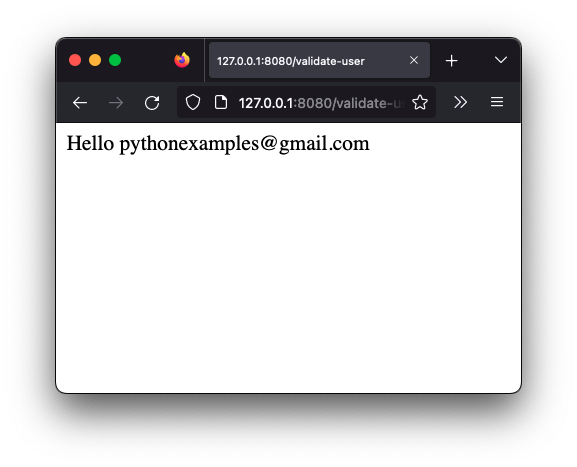
Thus, we have read the form data, in our Python flask application.
Summary
In this Python Flask Tutorial, we learned how to read the form data in flask application, with the help of an example.