Python Flask – Upload File
In a flask web application, we can upload a file to server from HTML form, and save it in desired location in server.
In this tutorial, we will write a simple flask application with HTML template that contains form element to upload the file, and a server side Python code to save the file in server.
Flask Application to Upload File
In this example, we build a flask application to enable the user to upload a file to server.
The flask application project structure is as shown in the following.
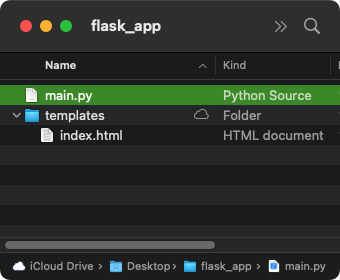
index.html
<!DOCTYPE html>
<html>
<body>
<h1>Upload File</h1>
<p>Welcome to sample flask application by <a href="https://pythonexamples.org/">PythonExamples.org</a>.</p>
<div>
<h3>Upload</h3>
<form action = "/upload-file" method = "POST"
enctype = "multipart/form-data">
<input type="file" name="file">
<input type="Submit" value="Upload">
</form>
<div style="color:orange">{{ message }}</div>
</div>
</body>
</html>
In the form element,
- When user clicks on the submit button, post request is sent to
/upload-file
URL. - Please note that the encryption type
enctype
for the form must be"multipart/form-data"
to upload a file.
main.py
from flask import Flask, render_template, request
import os
app = Flask(__name__)
app.config['UPLOAD_FOLDER'] = 'uploads'
@app.route("/")
def home_page():
return render_template("index.html")
@app.route('/upload-file', methods=['POST'])
def upload_file():
if request.method == 'POST':
if 'file' not in request.files:
return 'There is file is submitted form.'
file = request.files['file']
file.save(os.path.join(app.config['UPLOAD_FOLDER'], file.filename))
return 'File upload successful.'
else:
return 'Invalid request.'
if __name__ == "__main__":
app.run(host="127.0.0.1", port=8080, debug=True)
In upload_file()
function,
- we save the uploaded files to
uploads
folder. request.files['file']
accesses the file from the form data.file.save
is used to save the file to specific folder.file.filename
returns are original name of the uploaded file.
Run Application
Open terminal or command prompt at the root of the application, and run the Python file main.py.
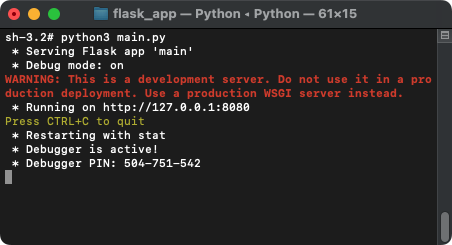
The flask application is up and running at URL http://127.0.0.1:8080/
.
Now, open a browser of your choice and hit the URL http://127.0.0.1:8080/
.
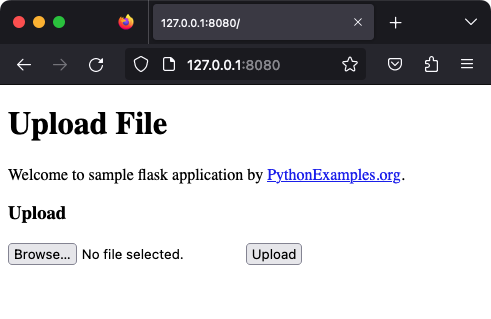
If you click on the Browse button, a window appears to select a file.
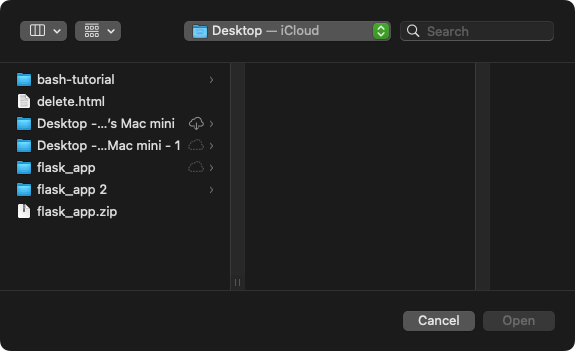
Select a file for upload and click on Open button.
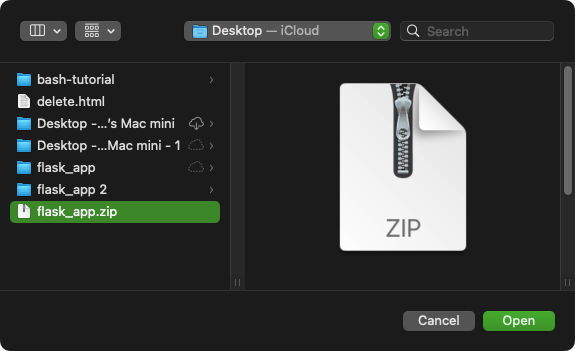
The file name appears next to the Browse button.
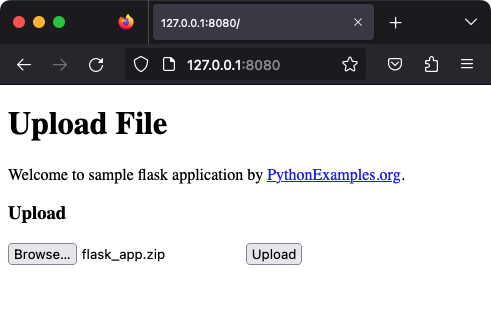
Now click on Upload button in the browser.
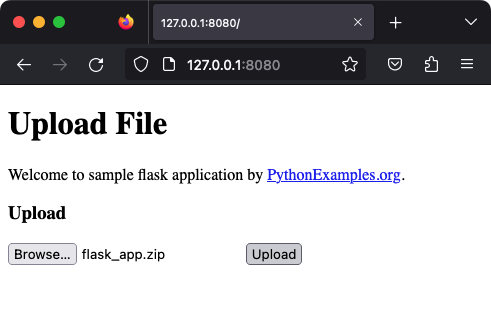
When you click on Upload button, the form element sends POST request to the URL /upload-file
as specified in the HTML template.
At the server side, we can access the uploaded file from request
object.
The expression: request.files['file']
, returns the file. You can use file.save()
method to save this file to specific location on the server.
In the following screenshot, you can observe that the flask_app.zip
has been uploaded to uploads
folder.
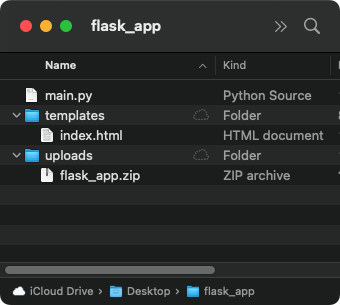
If the file upload is successful, the server responds with a string "File upload successful."
.
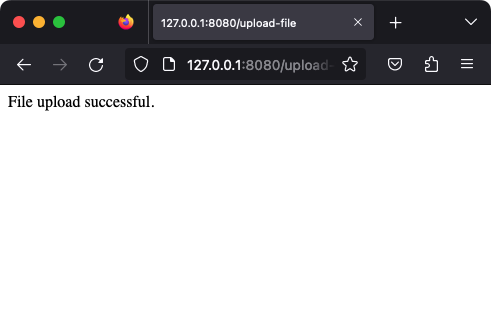
Instead of returning a string, you can render a template or redirect to another URL.
Project ZIP
Summary
In this Python Flask Tutorial, we learned how to upload a file in a flask application.