Contents
Python – Sort List of Strings based on String Length
To sort a list of strings based on string length in Python, call sort() method on the list object, and specify the key named argument of the sort() method with a function that returns the length of the given string.
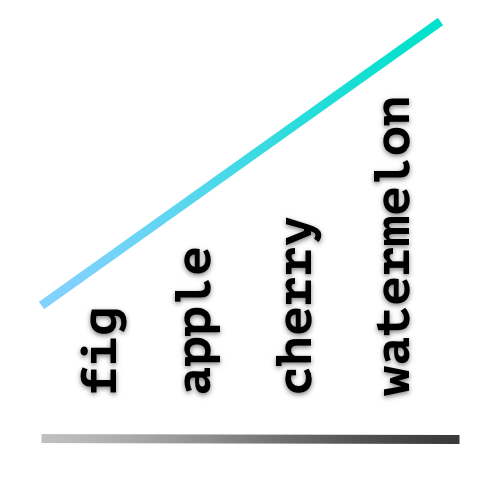
For example, when sorting strings based on length, 'fig' is less than 'apple', because length of 'fig' is 3 whereas length of 'apple' is 5. But if you take the actual value 'fig' is greater than 'apple', because 'fig' starts with f which is greater than that of a in 'apple'.
By default sort() method of a list instance sorts the list in ascending order based on the actual value. But, we need to sort the list of strings based on their length. Therefore, we need to specify the key function that is used for comparing the items in the list while sorting.
You may refer Python List sort() method for complete information on syntax and examples.
Examples
1. Sort list of strings based on string length in Python
In the following program, we take a list of strings in my_list, and sort them based on their string length using list sort() method.
We can define a function string_length that takes a string as argument and returns its length. Pass this function as value to the key named argument when calling sort() method on the list.
Python Program
def string_length(x):
return len(x)
my_list = ['cherry', 'watermelon', 'fig', 'apple']
my_list.sort(key=string_length)
print(my_list)
Run Code CopyOutput
['fig', 'apple', 'cherry', 'watermelon']
The list is sorted based on the string length.
Instead of defining a function that returns the string length, we can also specify a lambda function, as shown in the following program.
Python Program
my_list = ['cherry', 'watermelon', 'fig', 'apple']
my_list.sort(key=lambda x: len(x))
print(my_list)
Run Code CopyOutput
['fig', 'apple', 'cherry', 'watermelon']
Summary
In this Python Lists tutorial, we learned how to sort a list in descending order using list.sort() method.