MongoDB Aggregate
MongoDB Aggregate
In MongoDB, the aggregate
operation is used to process data records and return computed results. This command allows you to perform complex data aggregation tasks, such as filtering, grouping, and sorting, within MongoDB collections.
Syntax
db.collection.aggregate(pipeline, options)
The aggregate
method uses a pipeline
of stages to process documents and return aggregated results. The options
parameter can be used to customize the aggregation.
Example MongoDB Aggregate
Let's look at some examples of how to use the aggregate
method in the programGuru
collection in MongoDB:
1. Basic Aggregation
db.programGuru.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: "$age", count: { $sum: 1 } } },
{ $sort: { _id: 1 } }
])
This command filters documents where the age
is greater than 25, groups them by age
, and sorts the results by age
in ascending order.
2. Aggregation with Projection
db.programGuru.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $project: { name: 1, age: 1, _id: 0 } }
])
This command filters documents where the age
is greater than 25 and projects only the name
and age
fields.
3. Aggregation with Multiple Stages
db.programGuru.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: "$age", averageAge: { $avg: "$age" } } },
{ $sort: { averageAge: 1 } }
])
This command filters documents where the age
is greater than 25, groups them by age
, calculates the average age
, and sorts the results by the average age
in ascending order.
Full Example
Let's go through a complete example that includes switching to a database, creating a collection, inserting documents, and using the aggregate
method.
Step 1: Switch to a Database
This step involves switching to a database named myDatabase
.
use myDatabase
In this example, we switch to the myDatabase
database.
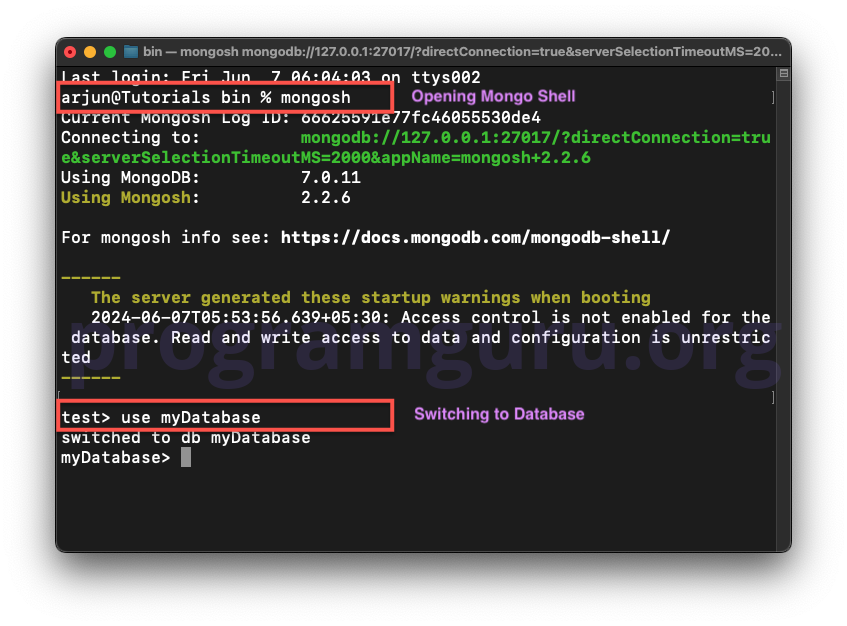
Step 2: Create a Collection
This step involves creating a new collection named programGuru
in the myDatabase
database.
db.createCollection("programGuru")
Here, we create a collection named programGuru
.
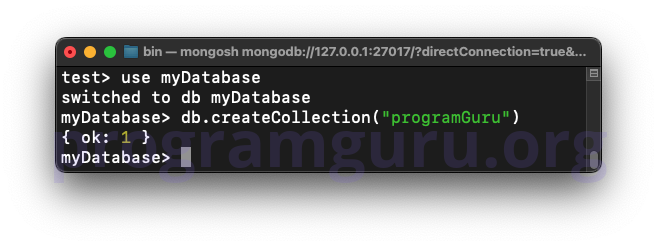
Step 3: Insert Documents into the Collection
This step involves inserting documents into the programGuru
collection.
db.programGuru.insertMany([
{ name: "John Doe", age: 30, email: "john.doe@example.com" },
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
We insert multiple documents into the programGuru
collection.
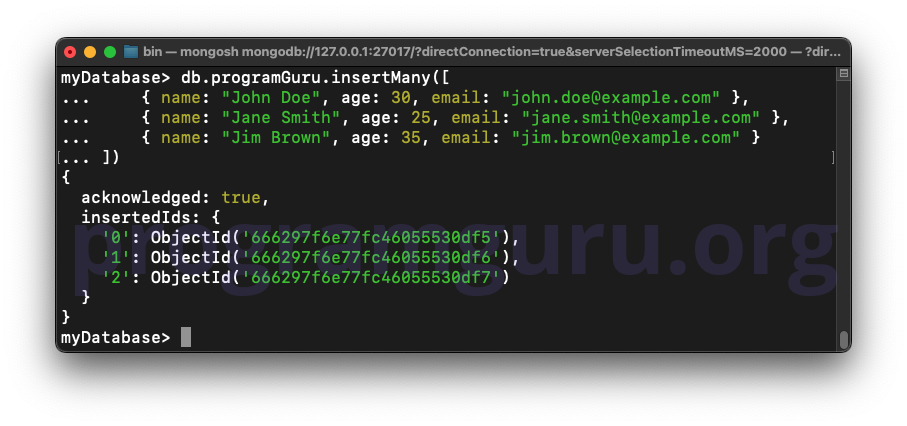
Step 4: Use the Aggregate Method
This step involves using the aggregate
method to process documents in the programGuru
collection.
Basic Aggregation
db.programGuru.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: "$age", count: { $sum: 1 } } },
{ $sort: { _id: 1 } }
]).pretty()
We filter documents where the age
is greater than 25, group them by age
, and sort the results by age
in ascending order.
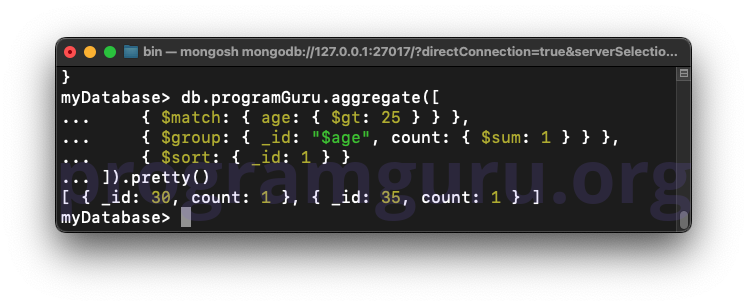
Aggregation with Projection
db.programGuru.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $project: { name: 1, age: 1, _id: 0 } }
]).pretty()
We filter documents where the age
is greater than 25 and project only the name
and age
fields.
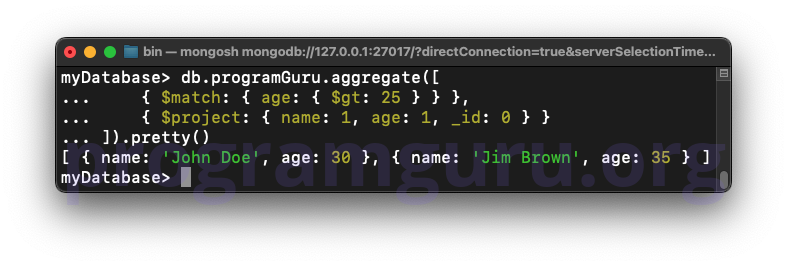
Aggregation with Multiple Stages
db.programGuru.aggregate([
{ $match: { age: { $gt: 25 } } },
{ $group: { _id: "$age", averageAge: { $avg: "$age" } } },
{ $sort: { averageAge: 1 } }
]).pretty()
We filter documents where the age
is greater than 25, group them by age
, calculate the average age
, and sort the results by the average age
in ascending order.
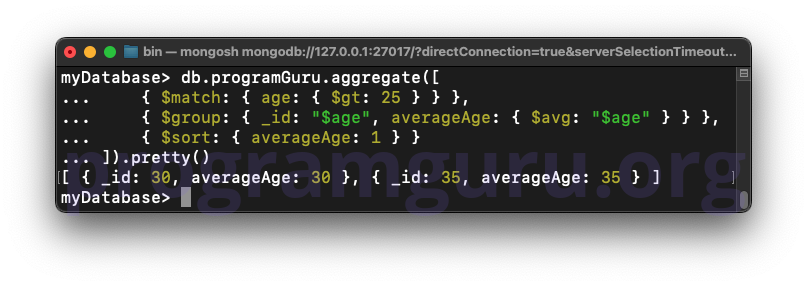
Conclusion
The MongoDB aggregate
operation is crucial for performing complex data processing tasks. Understanding how to use the aggregation framework allows you to efficiently filter, group, and transform data within MongoDB collections.