MongoDB Query Documents
MongoDB Query Documents
In MongoDB, querying documents from a collection is done using the find
method. This method allows you to retrieve documents that match specified criteria, enabling data extraction and analysis.
Syntax
db.collection.find(query, projection)
The find
method retrieves documents from a collection that match the query
criteria. The optional projection
parameter specifies the fields to return in the matching documents.
Example MongoDB Query Documents
Let's look at some examples of how to query documents in the programGuru
collection in MongoDB:
1. Query All Documents
db.programGuru.find()
This command retrieves all documents in the programGuru
collection.
2. Query Documents with a Filter
db.programGuru.find({ age: { $gt: 30 } })
This command retrieves documents where the age
is greater than 30.
3. Query Specific Fields
db.programGuru.find({ age: { $gt: 30 } }, { name: 1, age: 1, _id: 0 })
This command retrieves documents where the age
is greater than 30, returning only the name
and age
fields.
Full Example
Let's go through a complete example that includes switching to a database, creating a collection, inserting documents, querying them, and verifying the results.
Step 1: Switch to a Database
This step involves switching to a database named myDatabase
.
use myDatabase
In this example, we switch to the myDatabase
database.
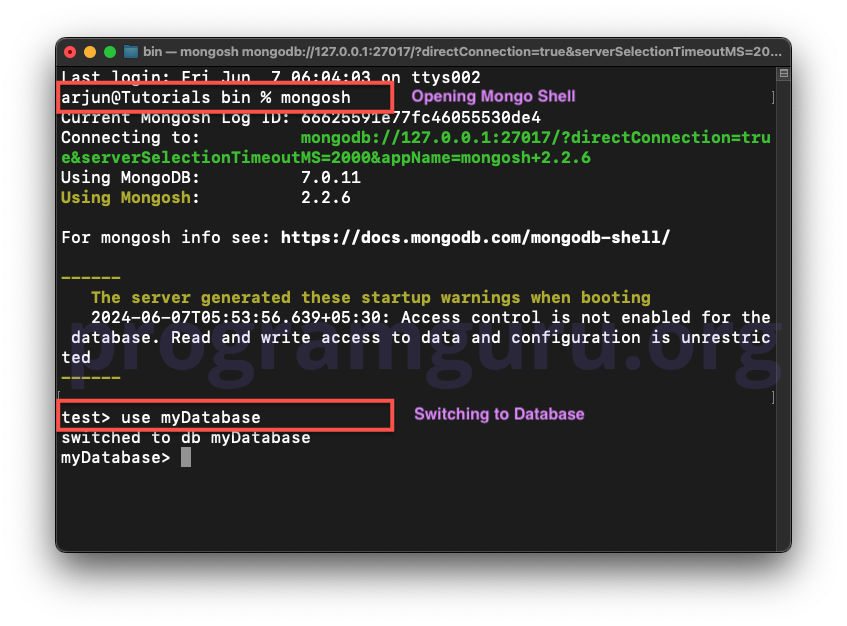
Step 2: Create a Collection
This step involves creating a new collection named programGuru
in the myDatabase
database.
db.createCollection("programGuru")
Here, we create a collection named programGuru
.
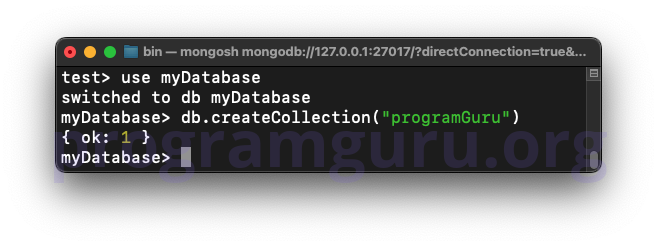
Step 3: Insert Documents into the Collection
This step involves inserting documents into the programGuru
collection.
db.programGuru.insertMany([
{ name: "John Doe", age: 30, email: "john.doe@example.com" },
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
We insert multiple documents into the programGuru
collection.
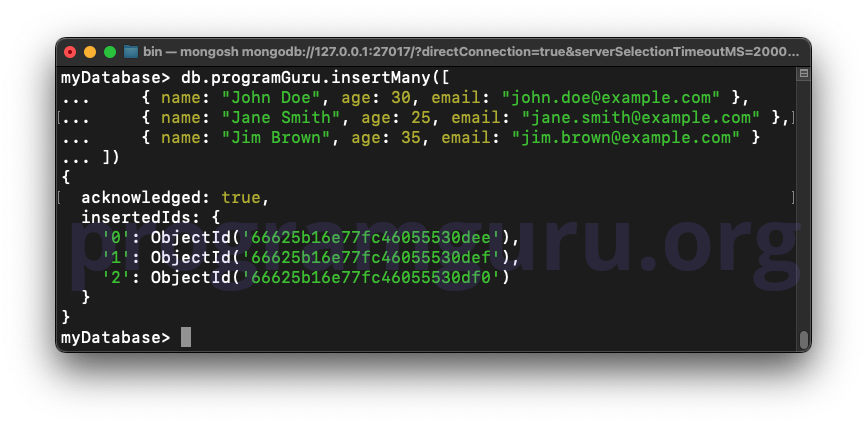
Step 4: Query Documents in the Collection
This step involves querying documents in the programGuru
collection.
Query All Documents
db.programGuru.find().pretty()
We retrieve all documents in the programGuru
collection.
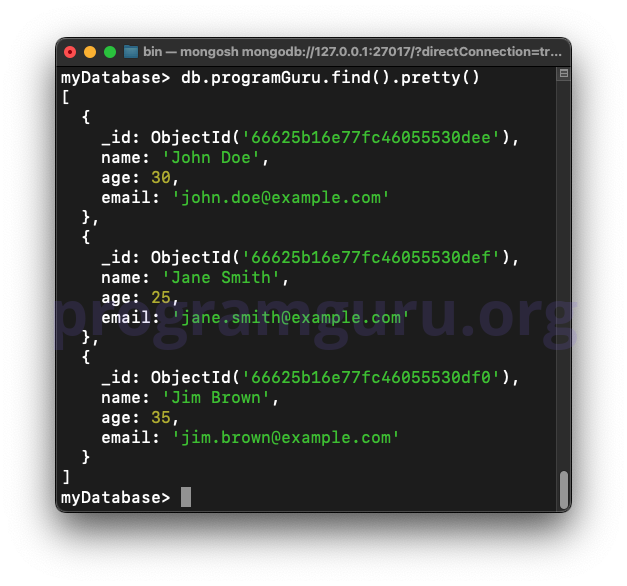
Query Documents with a Filter
db.programGuru.find({ age: { $gt: 30 } }).pretty()
We retrieve documents where the age
is greater than 30.
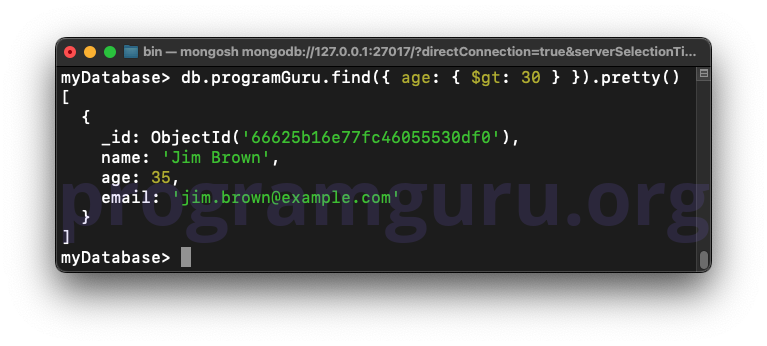
Query Specific Fields
db.programGuru.find({ age: { $gt: 30 } }, { name: 1, age: 1, _id: 0 }).pretty()
We retrieve documents where the age
is greater than 30, returning only the name
and age
fields.
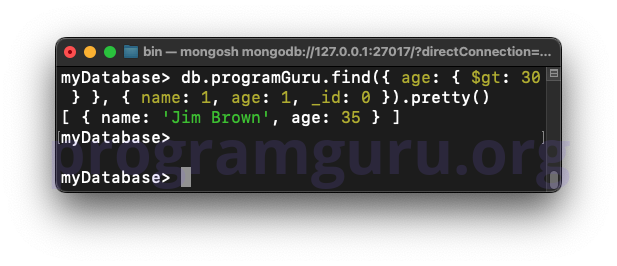
Conclusion
The MongoDB query documents
operation is crucial for extracting and analyzing data within collections. Understanding how to query single and multiple documents, as well as specific fields, is essential for effective data retrieval in MongoDB.