MongoDB Update Documents
MongoDB Update Documents
In MongoDB, updating documents in a collection is done using the updateOne
, updateMany
, and replaceOne
methods. These methods allow you to modify one or more documents within a collection based on specified criteria.
Syntax
db.collection.updateOne(filter, update, options)
db.collection.updateMany(filter, update, options)
db.collection.replaceOne(filter, replacement, options)
The updateOne
method updates a single document that matches the filter, updateMany
updates multiple documents, and replaceOne
replaces an entire document.
Example MongoDB Update Documents
Let's look at some examples of how to update documents in the programGuru
collection in MongoDB:
1. Update a Single Document
db.programGuru.updateOne(
{ name: "John Doe" },
{ $set: { age: 31 } }
)
This command updates the age
field to 31 for the document where the name
is John Doe
.
2. Update Multiple Documents
db.programGuru.updateMany(
{ age: { $gt: 25 } },
{ $set: { status: "Active" } }
)
This command updates the status
field to Active
for all documents where the age
is greater than 25.
3. Replace a Document
db.programGuru.replaceOne(
{ name: "Jane Smith" },
{ name: "Jane Smith", age: 26, email: "jane.smith@example.com", status: "Active" }
)
This command replaces the entire document where the name
is Jane Smith
with the new document.
Full Example
Let's go through a complete example that includes switching to a database, creating a collection, inserting documents, updating them, and verifying the updates.
Step 1: Switch to a Database
This step involves switching to a database named myDatabase
.
use myDatabase
In this example, we switch to the myDatabase
database.
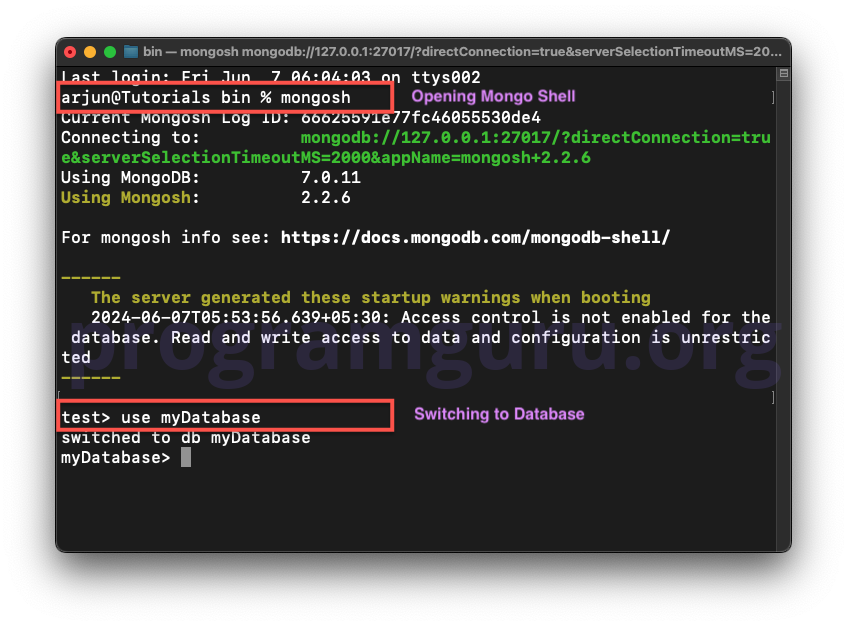
Step 2: Create a Collection
This step involves creating a new collection named programGuru
in the myDatabase
database.
db.createCollection("programGuru")
Here, we create a collection named programGuru
.
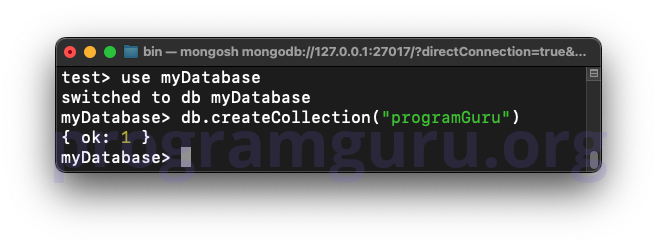
Step 3: Insert Documents into the Collection
This step involves inserting documents into the programGuru
collection.
db.programGuru.insertMany([
{ name: "John Doe", age: 30, email: "john.doe@example.com" },
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
We insert multiple documents into the programGuru
collection.
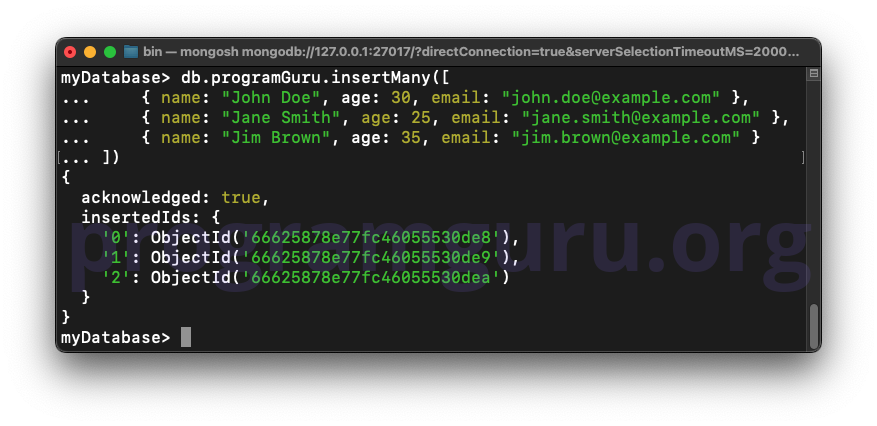
Step 4: Update Documents in the Collection
This step involves updating documents in the programGuru
collection.
Update a Single Document
db.programGuru.updateOne(
{ name: "John Doe" },
{ $set: { age: 31 } }
)
We update the age
field to 31 for the document where the name
is John Doe
.
Verify the Update
db.programGuru.find({ name: "John Doe" }).pretty()
This command retrieves the document where the name
is John Doe
to verify the update.
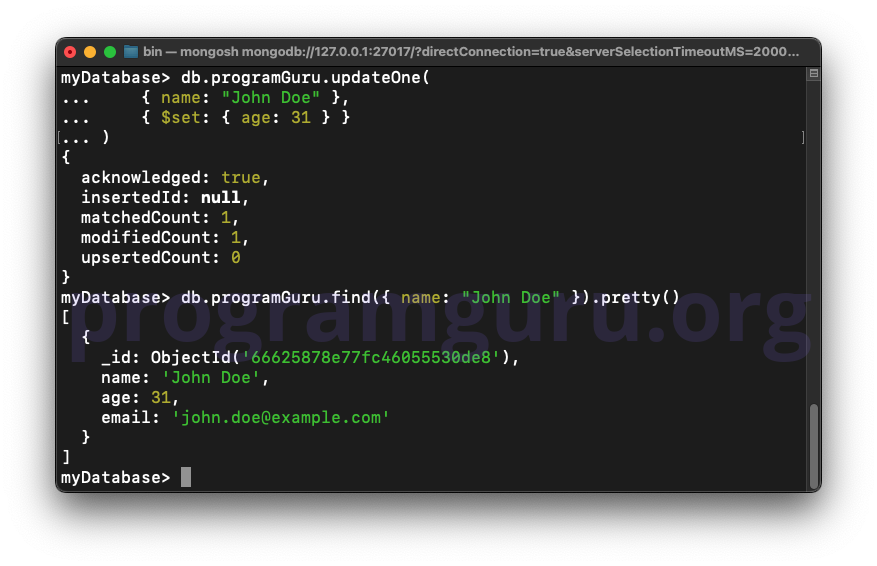
Update Multiple Documents
db.programGuru.updateMany(
{ age: { $gt: 25 } },
{ $set: { status: "Active" } }
)
We update the status
field to Active
for all documents where the age
is greater than 25.
Verify the Update
db.programGuru.find({ age: { $gt: 25 } }).pretty()
This command retrieves all documents where the age
is greater than 25 to verify the update.
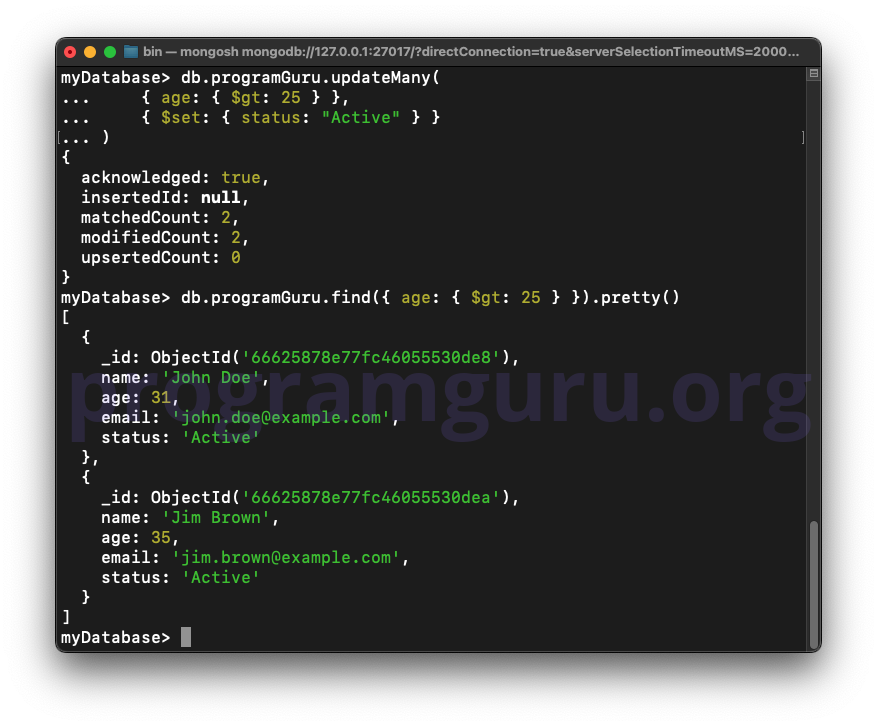
Replace a Document
db.programGuru.replaceOne(
{ name: "Jane Smith" },
{ name: "Jane Smith", age: 26, email: "jane.smith@example.com", status: "Active" }
)
We replace the entire document where the name
is Jane Smith
with the new document.
Verify the Replacement
db.programGuru.find({ name: "Jane Smith" }).pretty()
This command retrieves the document where the name
is Jane Smith
to verify the replacement.
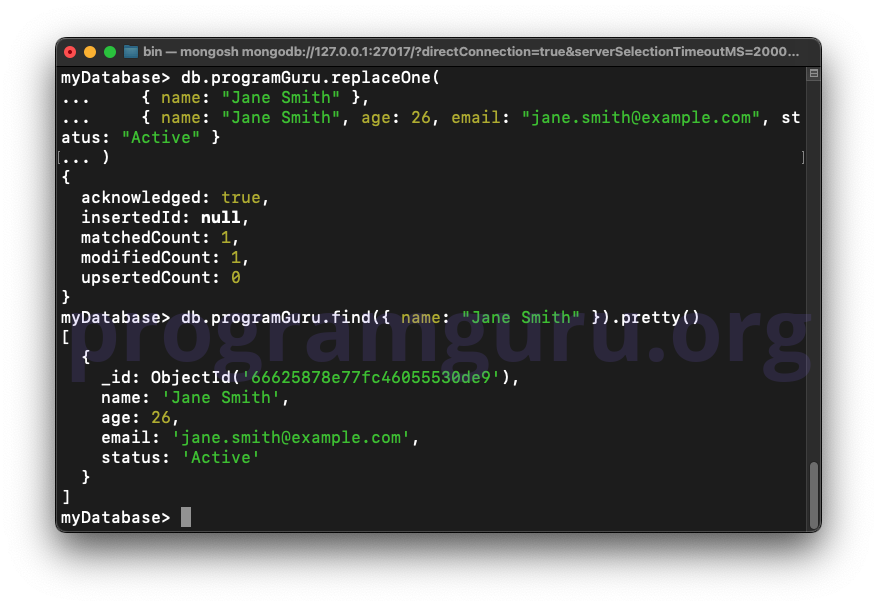
Conclusion
The MongoDB update documents
operation is crucial for modifying data within collections. Understanding how to update single and multiple documents, as well as replace entire documents, is essential for effective data management in MongoDB.