MongoDB Create Indexes
MongoDB Create Indexes
In MongoDB, the createIndexes
operation is used to create multiple indexes on a collection in a single command. This method allows you to optimize query performance and ensure efficient data retrieval within MongoDB collections.
Syntax
db.collection.createIndexes(indexes)
The createIndexes
method takes an array of index specification documents.
Example MongoDB Create Indexes
Let's look at an example of how to use the createIndexes
method in the programGuru
collection in MongoDB:
1. Create Multiple Indexes
db.programGuru.createIndexes([
{ key: { name: 1 }, name: "name_index" },
{ key: { age: -1 }, name: "age_index" },
{ key: { email: 1 }, name: "email_index", unique: true }
])
This command creates three indexes: an ascending index on the name
field, a descending index on the age
field, and a unique index on the email
field in the programGuru
collection.
Full Example
Let's go through a complete example that includes switching to a database, creating a collection, inserting documents, and creating multiple indexes.
Step 1: Switch to a Database
This step involves switching to a database named myDatabase
.
use myDatabase
In this example, we switch to the myDatabase
database.
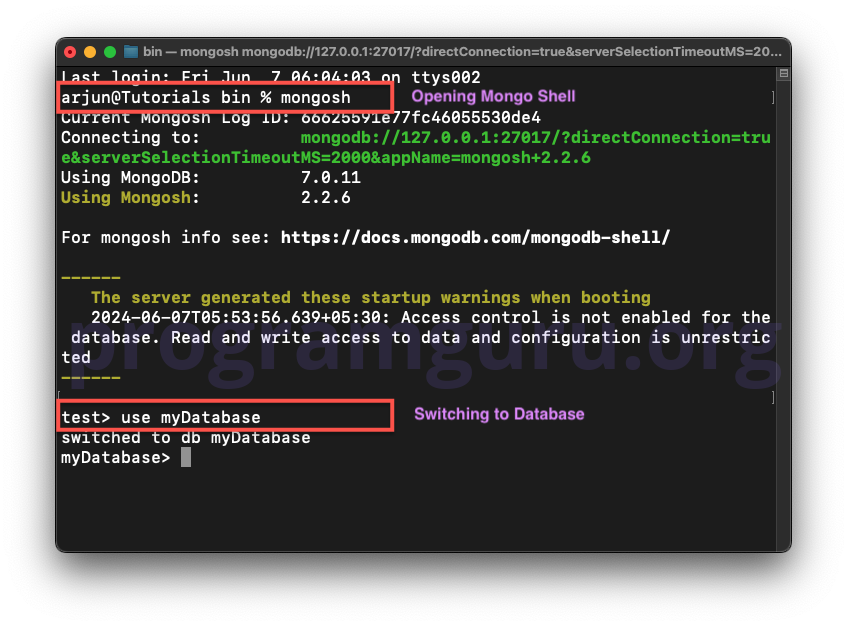
Step 2: Create a Collection
This step involves creating a new collection named programGuru
in the myDatabase
database.
db.createCollection("programGuru")
Here, we create a collection named programGuru
.
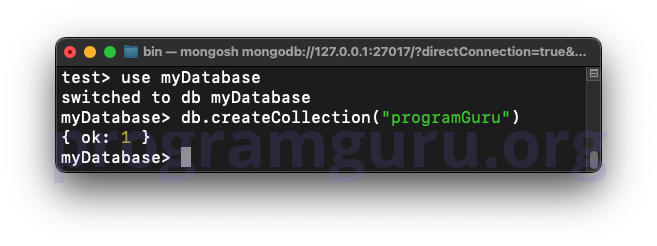
Step 3: Insert Documents into the Collection
This step involves inserting documents into the programGuru
collection.
db.programGuru.insertMany([
{ name: "John Doe", age: 30, email: "john.doe@example.com" },
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
We insert multiple documents into the programGuru
collection.
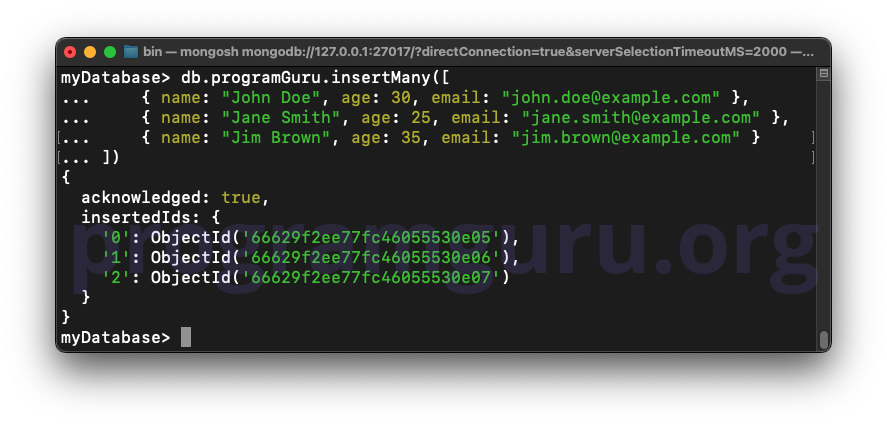
Step 4: Create Multiple Indexes in the Collection
This step involves using the createIndexes
method to create multiple indexes in the programGuru
collection.
db.programGuru.createIndexes([
{ key: { name: 1 }, name: "name_index" },
{ key: { age: -1 }, name: "age_index" },
{ key: { email: 1 }, name: "email_index", unique: true }
])
We create three indexes: an ascending index on the name
field, a descending index on the age
field, and a unique index on the email
field.

Conclusion
The MongoDB createIndexes
operation is crucial for optimizing query performance and ensuring efficient data retrieval. Understanding how to create multiple indexes in a single command allows you to enhance the performance and scalability of your MongoDB applications.