MongoDB Delete Documents
MongoDB Delete Documents
In MongoDB, deleting documents from a collection is done using the deleteOne
and deleteMany
methods. These methods allow you to remove one or more documents from a collection based on specified criteria.
Syntax
db.collection.deleteOne(filter)
db.collection.deleteMany(filter)
The deleteOne
method deletes a single document that matches the filter, while the deleteMany
method deletes multiple documents that match the filter.
Example MongoDB Delete Documents
Let's look at some examples of how to delete documents in the programGuru
collection in MongoDB:
1. Delete a Single Document
db.programGuru.deleteOne({ name: "John Doe" })
This command deletes a single document where the name
is John Doe
.
2. Delete Multiple Documents
db.programGuru.deleteMany({ age: { $gt: 30 } })
This command deletes all documents where the age
is greater than 30.
Full Example
Let's go through a complete example that includes switching to a database, creating a collection, inserting documents, deleting them, and verifying the deletions.
Step 1: Switch to a Database
This step involves switching to a database named myDatabase
.
use myDatabase
In this example, we switch to the myDatabase
database.
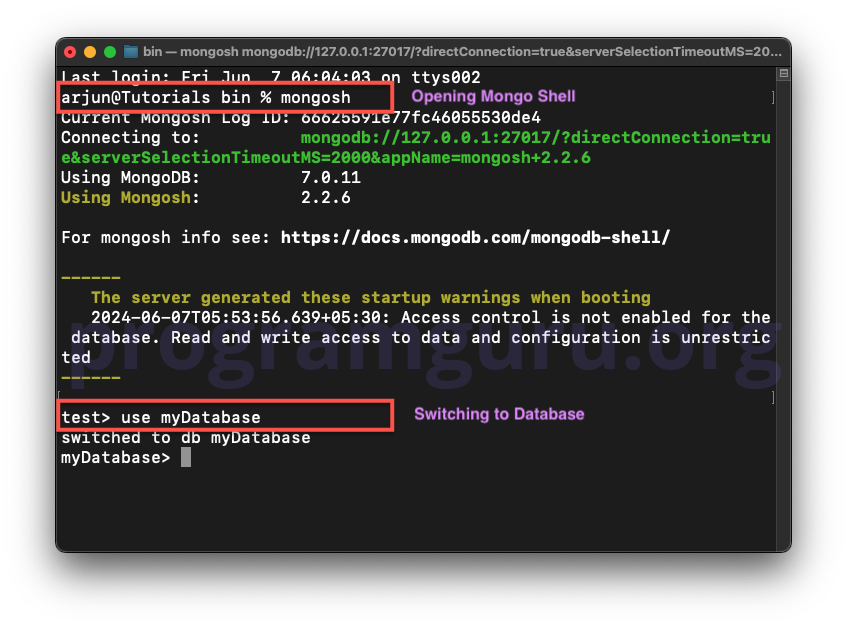
Step 2: Create a Collection
This step involves creating a new collection named programGuru
in the myDatabase
database.
db.createCollection("programGuru")
Here, we create a collection named programGuru
.
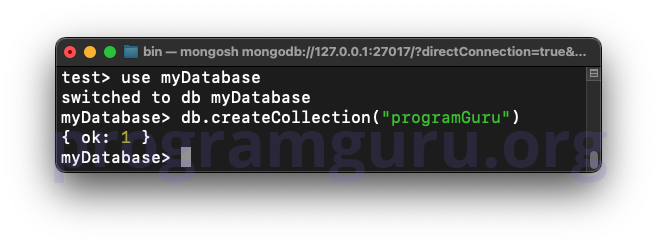
Step 3: Insert Documents into the Collection
This step involves inserting documents into the programGuru
collection.
db.programGuru.insertMany([
{ name: "John Doe", age: 30, email: "john.doe@example.com" },
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
We insert multiple documents into the programGuru
collection.
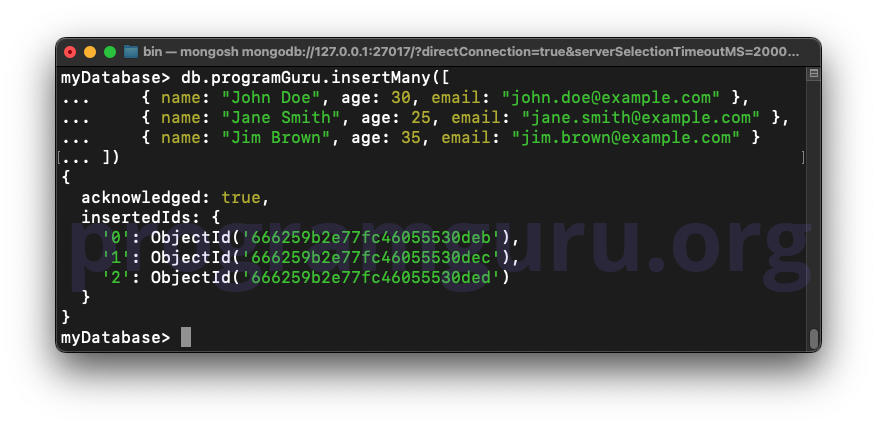
Step 4: Delete Documents in the Collection
This step involves deleting documents from the programGuru
collection.
Delete a Single Document
db.programGuru.deleteOne({ name: "John Doe" })
We delete a single document where the name
is John Doe
.
Verify the Deletion
db.programGuru.find({ name: "John Doe" }).pretty()
This command retrieves the document where the name
is John Doe
to verify the deletion.
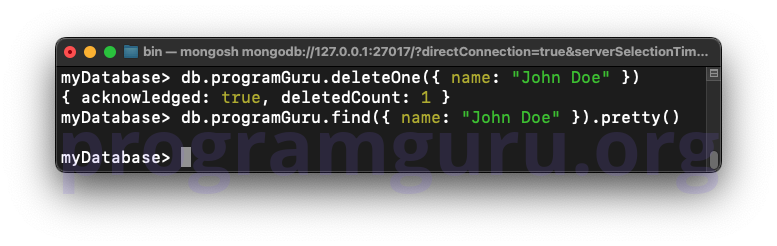
Delete Multiple Documents
db.programGuru.deleteMany({ age: { $gt: 30 } })
We delete all documents where the age
is greater than 30.
Verify the Deletion
db.programGuru.find({ age: { $gt: 30 } }).pretty()
This command retrieves all documents where the age
is greater than 30 to verify the deletion.
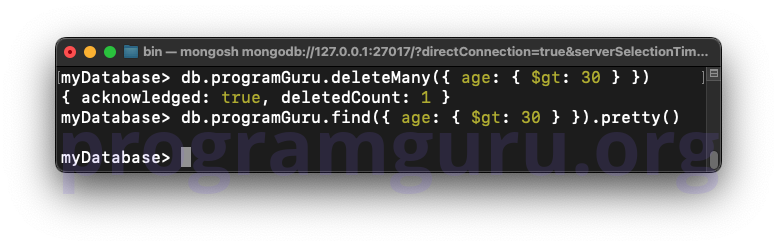
Conclusion
The MongoDB delete documents
operation is crucial for managing data within collections. Understanding how to delete single and multiple documents is essential for effective data management in MongoDB.