MongoDB Insert Document(s)
MongoDB Insert Document
In MongoDB, inserting documents into a collection is done using the insertOne
and insertMany
methods. These methods allow you to add one or more documents to a collection, respectively.
Syntax
db.collection.insertOne(document)
db.collection.insertMany([document1, document2, ...])
The insertOne
method inserts a single document, while the insertMany
method inserts multiple documents at once.
Example MongoDB Insert Document
Let's look at some examples of how to insert documents into a collection in MongoDB:
1. Insert a Single Document
db.programGuru.insertOne({ name: "John Doe", age: 30, email: "john.doe@example.com" })
This command inserts a single document into the programGuru
collection.
2. Insert Multiple Documents
db.programGuru.insertMany([
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
This command inserts multiple documents into the programGuru
collection.
Full Example
Let's go through a complete example that includes switching to a database, creating a collection, inserting documents, and verifying the insertions.
Step 1: Switch to a Database
This step involves switching to a database named myDatabase
.
use myDatabase
In this example, we switch to the myDatabase
database.
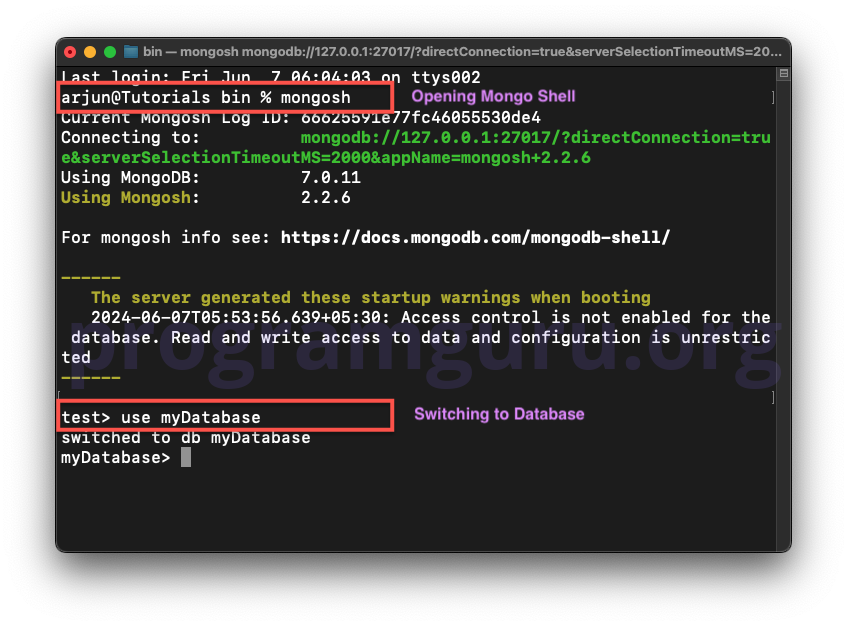
Step 2: Create a Collection
This step involves creating a new collection named programGuru
in the myDatabase
database.
db.createCollection("programGuru")
Here, we create a collection named programGuru
.
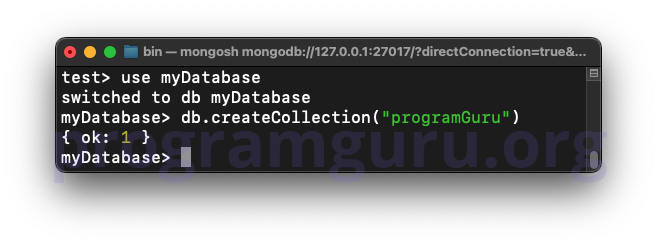
Step 3: Insert Documents into the Collection
This step involves inserting documents into the programGuru
collection.
Insert a Single Document
db.programGuru.insertOne({ name: "John Doe", age: 30, email: "john.doe@example.com" })
We insert a single document into the programGuru
collection.
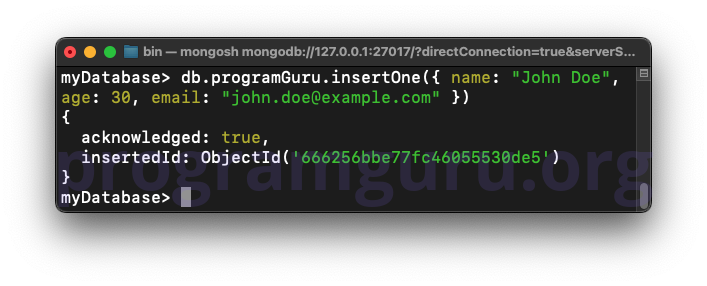
Insert Multiple Documents
db.programGuru.insertMany([
{ name: "Jane Smith", age: 25, email: "jane.smith@example.com" },
{ name: "Jim Brown", age: 35, email: "jim.brown@example.com" }
])
We insert multiple documents into the programGuru
collection.
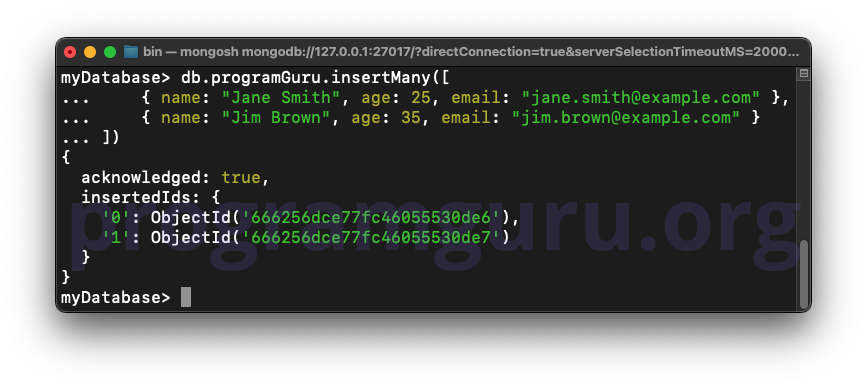
Step 4: Verify the Insertions
This step involves querying the programGuru
collection to verify that the documents have been inserted.
db.programGuru.find().pretty()
This command retrieves all documents from the programGuru
collection and displays them in a readable format.
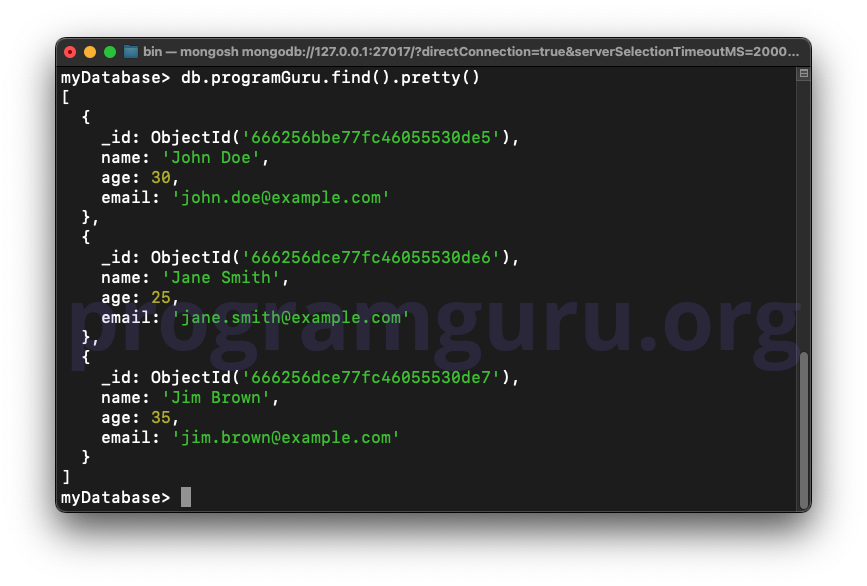
Conclusion
The MongoDB insert document
operation is essential for adding data to collections. Understanding how to insert single and multiple documents allows you to effectively manage your data in MongoDB.