Iterate over Words in String - 2 Python Examples
Python - Iterate Over Words of String
To iterate over words of a string,
- Split the string. The common delimiter between words in a string is space. The split returns an array. Each element in the array is a word.
- Use for loop to iterate over the words present in the array.
Examples
1. Iterate over words in given string
In this example, we will iterate over the words of a string and print them one by one.
Python Program
str = 'Hello! I am Robot. This is a Python example.'
#split string
splits = str.split()
#for loop to iterate over words array
for split in splits:
print(split)
Output
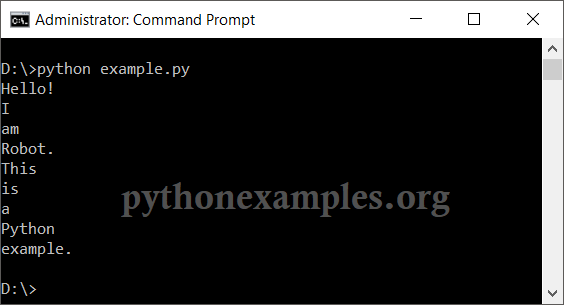
2. Clean given string and iterate over the words
In this example, we will clean the string and remove anything other than alphabets and spaces. Then we split the string and iterate over the words.
Python Program
import re
str = 'Hello! I am Robot. This is a Python example.'
#clean string
pat = re.compile(r'[^a-zA-Z ]+')
str = re.sub(pat, '', str).lower()
#split string
splits = str.split()
#for loop to iterate over words array
for split in splits:
print(split)
Output
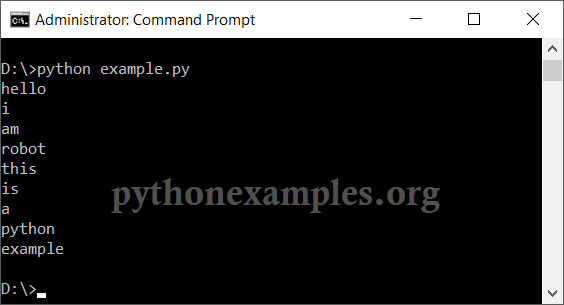
Summary
In this tutorial of Python Examples, we learned how to iterate over the words of given string.