How to print new line after variable in Python?
Python - Print new line after variable
To print a new line after a variable in Python, there are many ways.
- Using formatted string to print a new line after the variable.
- Using Addition Operator to concatenate new line character to the variable.
In this tutorial, we shall go through some examples where we use the above said approaches to print a new line after given variable.
1. Print new line after variable using formatted string in Python
Consider a scenario, where you have a variable x, and you would like to print the value in this variable followed by a new line. You can use the following print statement.
print(f"{x}\nWorld!")
Let us write a program where we take a variable, and print a new line after the variable in standard output.
Python Program
x = "Hello"
print(f"{x}\n")
Output
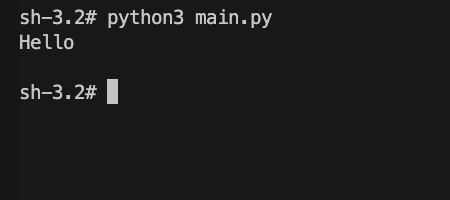
3. Print new line after variable using Addition Operator in Python
Consider this expression.
x + "\n"
In this expression, Addition operator concatenates new line to the variable x, and thus we have a new line after the string. During printing, the variable is printed to output, and then the content after the new line shall be printed in the next new line.
print(x + "\n")
Let us write a program where we take a variable, and print a new line after the variable in standard output using Addition operator +
.
Python Program
x = "Hello"
print(x + "\n")
Output
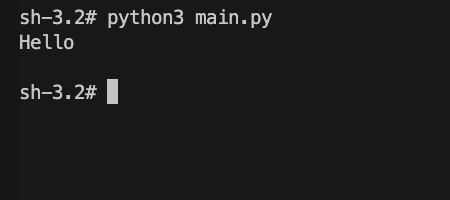
Summary
In this tutorial, we have seen how to print a new line after variable in Python, with examples covering scenarios where we write a new line character in the formatted string after the variable, or we concatenate the new line character to the variable using Addition Operator.