Python - Reverse String
Reverse String in Python
There is no standard function from Python to reverse a string, But we can use other methods to reverse a string, through slicing, for loop, etc.
In this tutorial, we will go through the above mentioned methods to reverse a String in Python.
Examples
1. Reverse string using slicing in Python
To reverse a string in Python, we will use slicing with negative step value. The slice expression would be as shown below.
[::-1]
Here we have not provided the start and stop, therefore slicing would happen across the whole string. step value of -1 would make the slicing happen in reverse order in steps of one, i.e., from end to start. And x[::-1]
returns required reversed string.
Python Program
# Given string
x = "Hello World"
# Reverse string using slicing
x_reversed = x[::-1]
print(f"Original string : {x}")
print(f"Reversed string : {x_reversed}")
Output
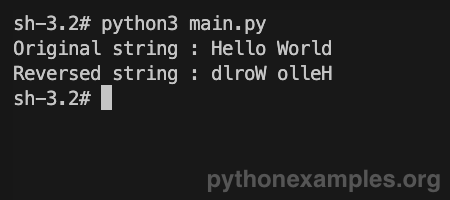
2. Reverse string using For loop in Python
In this example, we will reverse a string using Python For Loop. With for loop, we iterate over characters in string and append each character at the front of a new string(initially empty). By the end of the for loop, we should have a reversed string.
Python Program
# Given string
x = "Hello World"
# Reverse string using For loop
x_reversed = ''
for c in x:
x_reversed = c + x_reversed
# Print reversed string
print(x_reversed)
Output
dlroW olleH
3. Reverse string using While loop in Python
In this example, we use a Python While Loop statement, and during each iteration, we decrement the length. We use this length variable as index to extract values from string. With every step of the loop, we extract a character from decreasing end of the string.
Python Program
# Given string
x = "Hello World"
# Reverse string using While loop
x_reversed = ''
index = len(x) - 1
while index >= 0:
x_reversed = x_reversed + x[index]
index = index - 1
# Print reversed string
print(x_reversed)
Output
D:\>python example.py
.selpmaxE nohtyP ot emocleW
4. Reverse string using List reverse() method in Python
Steps
- Given a string in x.
- Convert string x into a list of characters x_chars.
- Use List reverse() method to reverse the order of characters in x_chars. Call reverse() method on x_chars, and the method reverses the list in place.
- Join the characters in the returned list using string join() method. The resulting string is a reversed string of the original string x.
Program
The complete program to reverse a string using List reverse() method.
Python Program
# Given string
x = "Hello World"
# Convert string into list of chars
x_chars = list(x)
# Reverse the list
x_chars.reverse()
# Join the characters in the list
x_reversed = ''.join(x_chars)
# Print reversed string
print(x_reversed)
Output
dlroW olleH
Summary
In this tutorial of Python Examples, we learned how to reverse a String in Python language with the help of well detailed example programs.