Python String center() method
Python String center() method is used to center align the given string in the specified length, with specified fill character.
Consider that given string is in x.
x = "Hello World"
Then the return value of x.center(21) is
" Hello World "
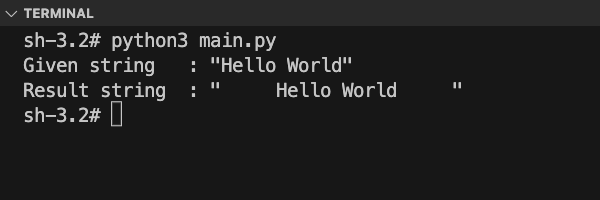
Syntax of center() method
The syntax of String center() method in Python is given below.
string.center(length, character)
The center() method takes two parameters.
Parameter | Description |
---|---|
length | [Mandatory] An integer value. Required length of the resulting string. |
character | [Optional] A character string value. Fill character to be used at the edges of the original string. The default fill character is single space ” “. |
center() method returns a new string created using the given string and appending equal number of fill characters at the edges till the length of resulting string reaches the given length.
The original string is not modified in value, and remains the same.
Examples
1. Center given Python string to a length of 21
In the following program, we take a string in variable x, and center the string to a resulting length of 21 using String center() method. We shall store the center() returned value in result variable. And then print both the original string x and centered string result.
Since fill character (second argument) is not specified, single space shall be used as fill character.
Python Program
x = "Hello World"
result = x.center(21)
print(f"Given string : \"{x}\"")
print(f"Centered string : \"{result}\"")
Output
Given string : "Hello World"
Centered string : " Hello World "
2. Center given Python string to a length of 21 with fill character hyphen
In the following program, we take a string in variable x, and center the string to a resulting length of 21 with fill character “-“ using String center() method.
Python Program
x = "Hello World"
result = x.center(21, "-")
print(f"Given string : \"{x}\"")
print(f"Centered string : \"{result}\"")
Output
Given string : "Hello World"
Centered string : "-----Hello World-----"
Summary
In this tutorial of Python String Methods, we learned about String center() method, its syntax, and examples.