Create Table in Python MySQL
Python MySQL - Create Table
To create a table in Python MySQL,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get the cursor to the database using cursor() function.
- Take a CREATE TABLE statement and execute it using execute() function.
Example
Consider that we have a schema named mydatabase in MySQL. We have access to the database with credentials: user - root
and password - admin1234
.
In the following program, we create a table named fruits
, with columns: name
, quantity
, and country
, where name
is the PRIMARY KEY.
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "CREATE TABLE fruits (name VARCHAR(50) NOT NULL, quantity INT DEFAULT 0, country VARCHAR(50), PRIMARY KEY (name))"
mycursor.execute(sql)
print('Table created successfully.')
mydb.commit()
Database before table creation
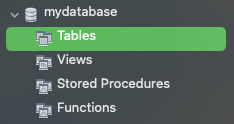
Database after table creation
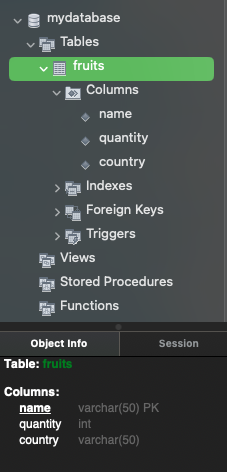
Summary
In this tutorial of Python Examples, we learned how to create a table in MySQL database.