Python MySQL – Rename table
To rename a table in MySQL from a Python program,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get cursor object to the database using cursor() function.
- Call execute() function on the cursor object, and pass the ALTER table query with RENAME TO clause and old table name, new table name. The ALTER query renames the table to the new name.
Example
Consider that there is a schema named mydatabase
in MySQL. The credentials to access this database are, user: root
and password: admin1234
, and there is a table named fruits
in mydatabase
.
In the following program, we rename the table from fruits
to stocks
using RENAME query.
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
try:
mycursor.execute("ALTER TABLE fruits RENAME TO stocks")
print('Renamed table successfully.')
except:
print('An exception occurred while renaming table.')
mydb.commit()
Output
Renamed table successfully.
Table before RENAME
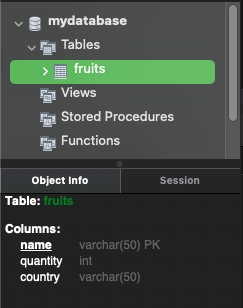
Table after RENAME
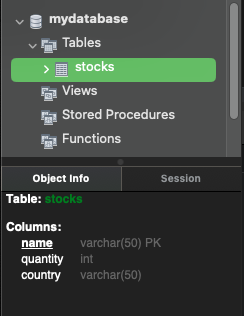
Summary
In this tutorial of Python Examples, we learned how to rename a table in MySQL, from a Python program.