Get First Row in Table in Python MySQL
Python MySQL - Get first row in table
To get the first row in a MySQL table in Python,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get cursor object to the database using cursor() function.
- Take SELECT from table query, with LIMIT clause set to 1.
- Call execute() function on the cursor object, and pass the select query as argument to execute() function.
- Now, fetch the records from the cursor object, which contains only the first row, if there is at least one row in the table.
Example
Consider that there is a schema named mydatabase
in MySQL. The credentials to access this database are, user root
and password admin1234
, and there is a table named fruits
in mydatabase
.
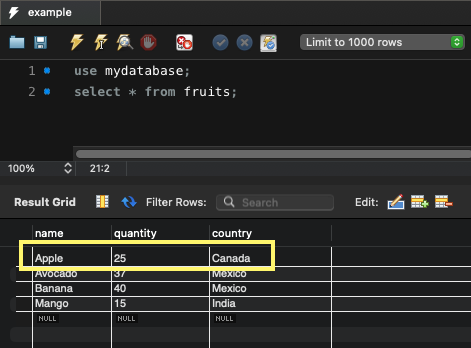
In the following program, we get the first record from fruits
table.
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
sql = "SELECT * FROM fruits LIMIT 1"
mycursor.execute(sql)
myresult = mycursor.fetchall()
first = myresult[0] if len(myresult) else None
print(first)
Output
('Apple', 25, 'Canada')
Summary
In this tutorial of Python Examples, we learned how to get the first record from table in MySQL database, from a Python program.