Delete all Rows from Table in Python MySQL
Python MySQL - Delete all rows from table
To delete all the rows from a MySQL table in Python program,
- Create a connection to the MySQL database with user credentials and database name, using connect() function.
- Get cursor object to the database using cursor() function.
- Call execute() function on the cursor object, and pass the DELETE FROM table query. The DELETE query deletes all the rows from table.
Example
Consider that there is a schema named mydatabase
in MySQL. The credentials to access this database are, user: root
and password: admin1234
, and there is a table named fruits
in mydatabase
.
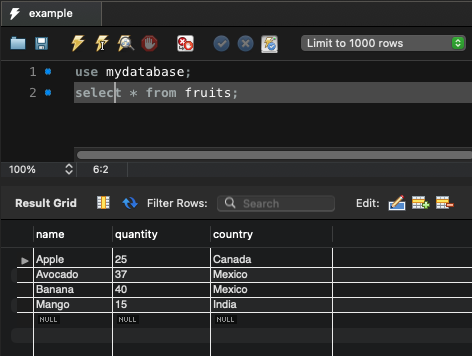
In the following program, we delete all the rows from fruits
table.
Python Program
import mysql.connector
mydb = mysql.connector.connect(
host="localhost",
user="root",
password="admin1234",
database="mydatabase"
)
mycursor = mydb.cursor()
try:
mycursor.execute("DELETE FROM fruits")
print('All rows are deleted.')
except:
print('An exception occurred while deleting rows.')
mydb.commit()
Output
All rows are deleted.
Table data after DELETE operation
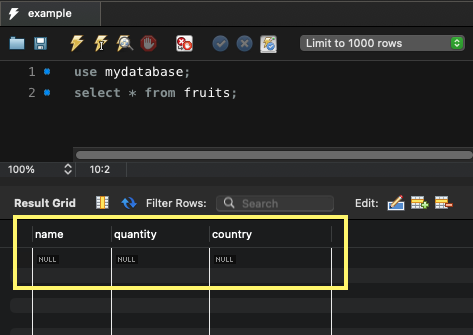
Summary
In this tutorial of Python Examples, we learned how to delete all the rows from a table, from a Python program.