Contents
Lambda Function that returns Multiple Values in Python
In Python, Lambda Functions are designed to return the value of a single expression. But, if we would like to return multiple values from a lambda function, we can do so by returning these multiple values in a single expression.
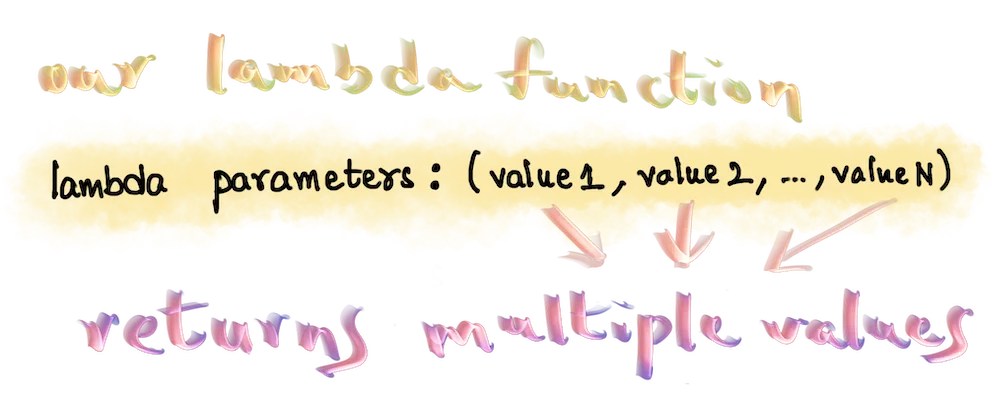
How do we send multiple values in a single expression?
The answer is by using a tuple or list. Send these multiple values as items of a tuple or list. Since a tuple or a list is just a single expression, lambda function has no problem returning it.
For example, consider the following lambda function that takes two parameters: x and y. And we would like to return the result of their addition, subtraction, and multiplication. To return these multiple values, return the addition, subtraction, and multiplication as elements of a tuple.
lambda x,y: (x+y, x-y, x*y)
To return these values in a list, just use list notation of square brackets, as shown in the following.
lambda x,y: [x+y, x-y, x*y]
Examples
1. Calculator lambda function that returns multiple values
In the following program, we define a lambda function assigned to a variable calculator. This lambda function takes two numbers via the parameters x and y, and returns the sum x+y, difference x-y, and product x*y packed in a tuple.
Since the filter() returns an iterable, we convert the iterable to a list.
Python Program
calculator = lambda x,y: (x+y, x-y, x*y)
# Call lambda function
result = calculator(5, 4)
print(result)
Run Code CopyOutput
(9, 1, 20)
2. Unpack multiple values returned by lambda function into individual variables
In the above program, we returned multiple values in a tuple. Now, we shall unpack those values into individual variables.
Python Program
calculator = lambda x,y: (x+y, x-y, x*y)
# Call lambda function, and unpack values into multiple variables
addition, subtraction, multiplication = calculator(5, 4)
print(f"Addition : {addition}")
print(f"Subtraction : {subtraction}")
print(f"Multiplication : {multiplication}")
Run Code CopyOutput
Addition : 9
Subtraction : 1
Multiplication : 20
Summary
In this tutorial of Python Lambda Functions, we learned how to return multiple values from a lambda function using list or tuple.