Contents
Lambda Function with filter() in Python
In Python, you can use a lambda function in a filter() function to filter the elements based on a condition defined in the lambda function.
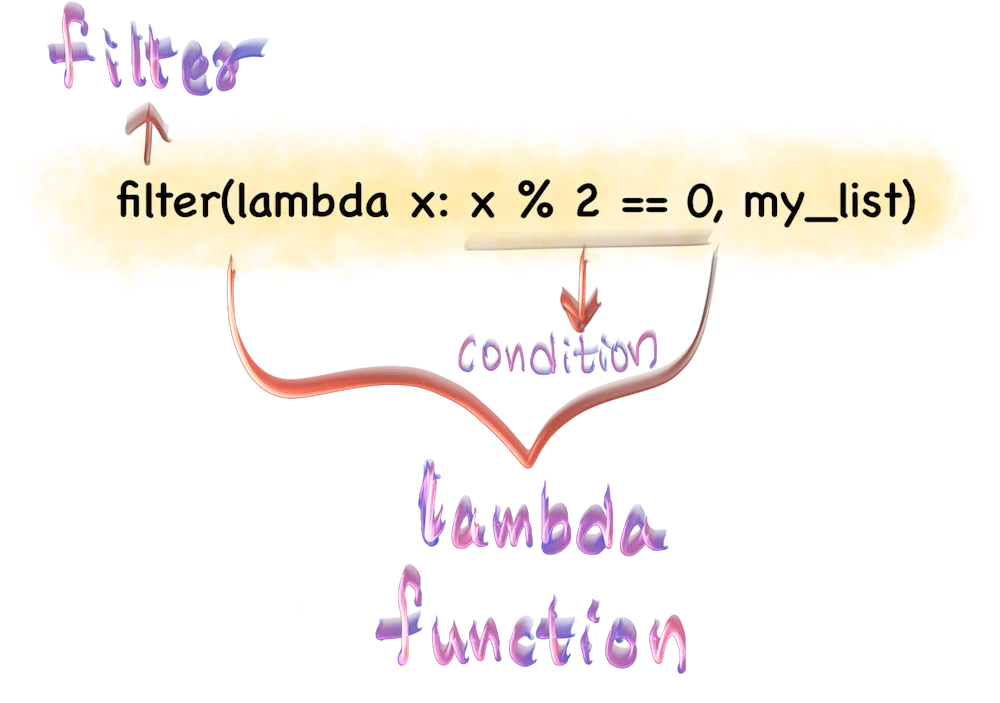
For example, consider the following lambda function with filter().
filter(lambda x: x % 2 == 0, my_list)
This lambda function filters out only the even numbers from the list my_list.
Observation
Please observe that the lambda function returns a boolean value. This is because the function that we use for a filter() must return a boolean value.
Reference: filter() builtin function.
Examples
1. Filter even numbers in list using lambda function
In the following program, we define a lambda function in the filter(), to filter out only even numbers from the list my_list.
Since the filter() returns an iterable, we convert the iterable to a list.
Python Program
my_list = [1, 2, 7, 4, 6, 9, 12, 16, 3]
# Lamdba function with filter()
result = filter(lambda x: x % 2 == 0, my_list)
# Converting the returned iterable to a list
result = list(result)
print(result)
Run Code CopyOutput
[2, 4, 6, 12, 16]
2. Filter non empty string using lambda function with filter()
In the following program, we define a lambda function with filter() that filters out only non-empty strings from the given list of strings my_list.
Python Program
my_list = ['apple', '', '', 'banana', 'cherry', '']
# Lamdba function with filter()
result = filter(lambda x: len(x) != 0, my_list)
# Converting the returned iterable to a list
result = list(result)
print(result)
Run Code CopyOutput
['apple', 'banana', 'cherry']
Summary
In this tutorial of Python Lambda Functions, we learned how to use a lambda function with filter(), with the help of some example programs.