Contents
Pillow – Apply filter on image
We can apply filter on an image in Pillow, by calling Image.filter() function on the given image and passing required filter kernel as argument. The filter can be a predefined filter or custom built filter.
In this tutorial, we will learn how to use predefined image filters, or create a custom filter and apply the filters on an image, with examples.
Syntax of filter() function
The syntax of putpixel() function from PIL.Image module is
PIL.Image.filter(filter_kernel)
where
Parameter | Description |
---|---|
filter_kernel | Filter kernel object. |
Pillow library has some inbuilt image filters which we can use directly without worrying about building a filter from scratch.
The following are the list of predefined Image filters in ImageFilter module.
- PIL.ImageFilter.BLUR
- PIL.ImageFilter.CONTOUR
- PIL.ImageFilter.DETAIL
- PIL.ImageFilter.EDGE_ENHANCE
- PIL.ImageFilter.EDGE_ENHANCE_MORE
- PIL.ImageFilter.EMBOSS
- PIL.ImageFilter.FIND_EDGES
- PIL.ImageFilter.SHARPEN
- PIL.ImageFilter.SMOOTH
- PIL.ImageFilter.SMOOTH_MORE
Or we can provide a custom ImageFilter object created from filter kernel matrix.
For example, the following kernel sharpens an image.
kernel = [-1, -1, -1,
-1, 9, -1,
-1, -1, -1]
The following kernel smoothens an image.
kernel = [1, 1, 1,
1, 3, 1,
1, 1, 1]
1. Apply filter on image using predefined ImageFilter
In the following example, we read an image test_image.jpg into an Image object. Then, we set the pixel at (50, 100) with a pixel value of (255, 255, 255) using Image.putpixel() function.
Python Program
from PIL import Image
# Open the image
image = Image.open("test_image.jpg")
# (x, y) position
xy = (50, 100)
# Pixel value
value = (255, 255, 255)
# Set pixel value
image.putpixel(xy, value)
image.save("resulting_image.jpg")
CopyOriginal Image [test_image.jpg]
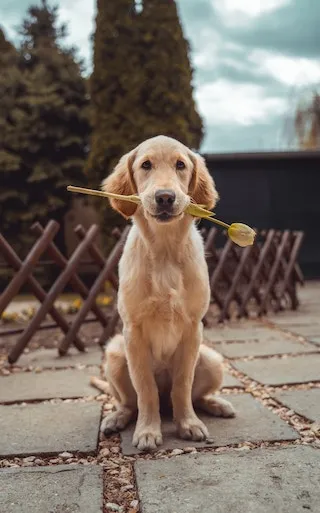
Output Image [resulting_image.jpg]
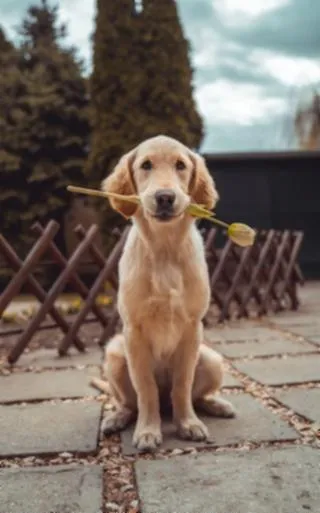
More tutorials on using predefined ImageFilter.
2. Apply filter on image using custom kernel
A custom kernel can be created using ImageFilter.Kernel().
The syntax of ImageFilter.Kernel() is
PIL.ImageFilter.Kernel(size, kernel, scale=None, offset=0)
where
Parameter | Description |
---|---|
size | Size of the kernel. It must be a tuple of (3, 3) or (5, 5) for a 3×3 filter matrix or 5×5 filter matrix respectively. |
kernel | A sequence containing kernel weights. We are addressing this kernel as filter matrix through out this tutorial. |
scale | The result for each pixel is divided by this value. |
offset | This value is added to each pixel, after scale. |
Now, let us start creating a kernel.
Suppose we would like to smoothen an image. To smoothen an image, we need to average out with the surrounding pixels while considering a more weight for the current pixel. We can consider the following sequence.
kernel = [1, 1, 1,
1, 3, 1,
1, 1, 1]
Since, each pixel is a sum of surrounding eight pixels, and three times the current pixel, we have scale the result by 11. Therefore our scale parameter must be set to 11.
scale=11
Since we have taken nine elements for our kernel sequence, the size of the kernel becomes (3, 3). If we have take a kernel sequence with twenty five elements, then the size of the kernel must have been (5, 5).
size=(5,5)
And we want no offset to be added to each pixel. Therefore we set offset to zero.
offset=0
Considering all the parameters that we have finalised, let us create an ImageFilter Kernel object.
kernel = [1, 1, 1,
1, 3, 1,
1, 1, 1]
kernel_filter = ImageFilter.Kernel((3, 3), kernel, scale=11, offset=0)
We can pass this kernel_filter object as argument to Image.filter() function to apply the kernel on this image.
In the following example, we take an image test_image.jpg, and then apply the above kernel filter on this image.
Python Program
from PIL import Image, ImageFilter
# Open the image
image = Image.open("test_image.jpg")
# A kernel sequence
kernel = [1, 1, 1,
1, 3, 1,
1, 1, 1]
kernel_filter = ImageFilter.Kernel((3, 3), kernel, scale=11, offset=0)
# Apply filter on the image
resulting_image = image.filter(kernel_filter)
# Save the resulting image
resulting_image.save("resulting_image.jpg")
CopyOriginal Image [test_image.jpg]
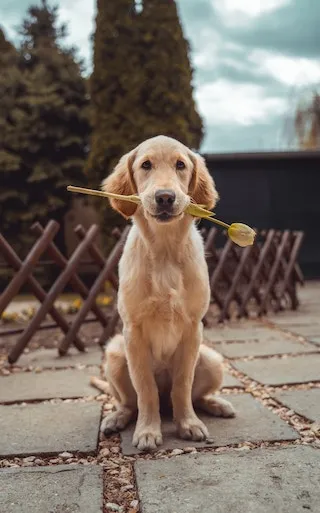
Resulting Image [resulting_image.jpg]
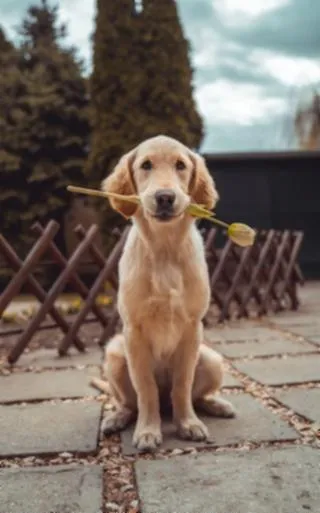
Similarly we can create out own kernel filters based on the requirement of filtering needs we have, for an image.
Summary
In this Python Pillow Tutorial, we learned how to filter an image using PIL.Image.filter() function, what are the different predefined image filters we have, and how to create a custom filter, with the help of examples.