Convert Pandas DataFrame to NumPy Array
Convert Pandas DataFrame to NumPy Array
You can convert a Pandas DataFrame to Numpy Array to perform some high-level mathematical functions supported by Numpy package.
To convert Pandas DataFrame to Numpy Array, use the function DataFrame.to_numpy()
. to_numpy() is applied on this DataFrame and the method returns object of type Numpy ndarray. Usually the returned ndarray is 2-dimensional.
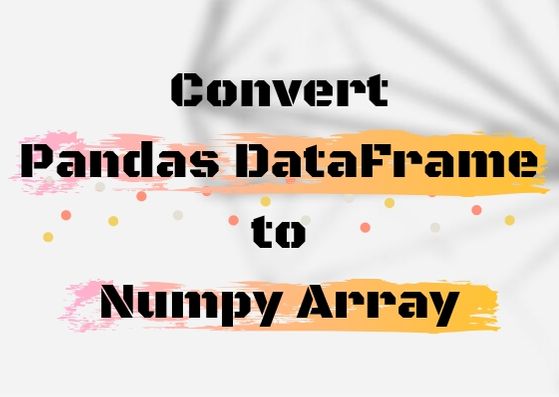
Examples
1. Convert DataFrame to Numpy Array
In the following example, we convert the DataFrame to numpy array.
Python Program
import pandas as pd
# Initialize a DataFrame
df = pd.DataFrame(
[[21, 72, 67],
[23, 78, 69],
[32, 74, 56],
[52, 54, 76]],
columns=['a', 'b', 'c'])
print('DataFrame\n----------\n', df)
# Convert DataFrame to numpy array
arr = df.to_numpy()
print('\nNumpy Array\n----------\n', arr)
df.to_numpy()
statement converts the dataframe to numpy array and returns the numpy array.
Output
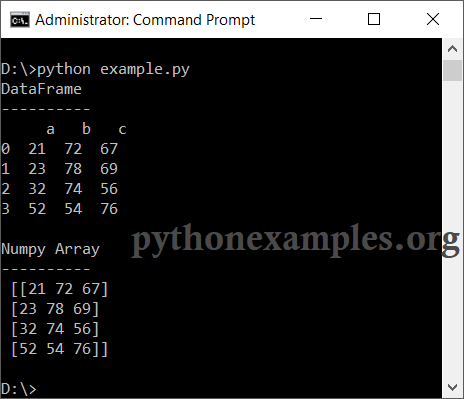
2. Convert DataFrame to Numpy Array when DataFrame has different datatypes
When you have a DataFrame with columns of different datatypes, the returned NumPy Array consists of elements of a single datatype. The lowest datatype of DataFrame is considered for the datatype of the NumPy Array.
In the following example, the DataFrame consists of columns of datatype int64 and float64. When this DataFrame is converted to NumPy Array, the lowest datatype of int64 and float64, which is float64 is selected.
Python Program
import pandas as pd
import numpy as np
# Initialize a DataFrame
df = pd.DataFrame(
[[21, 72, 67.1],
[23, 78, 69.5],
[32, 74, 56.6],
[52, 54, 76.2]],
columns=['a', 'b', 'c'])
print('DataFrame\n----------\n', df)
print('\nDataFrame datatypes :\n', df.dtypes)
# Convert DataFrame to numpy array
arr = df.to_numpy()
print('\nNumpy Array\n----------\n', arr)
print('\nNumpy Array Datatype :', arr.dtype)
Output
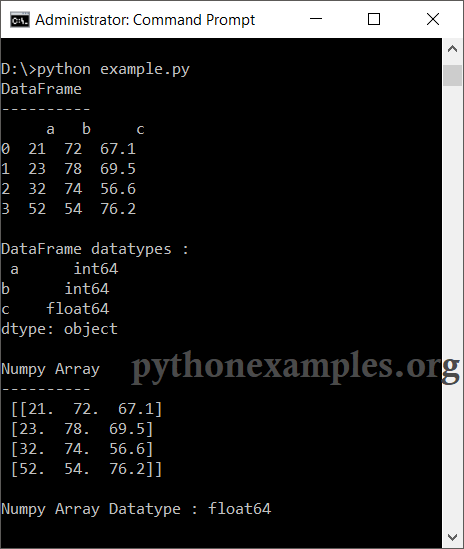
The returned Numpy Array is of type float64.
Summary
In this tutorial of Python Examples, we have learned how to convert Pandas DataFrame to Numpy Array.