Contents
Get Shape of Pandas DataFrame
To get the shape of Pandas DataFrame, use DataFrame.shape. The shape property returns a tuple representing the dimensionality of the DataFrame. The format of shape would be (rows, columns).
In this tutorial, we will learn how to get the shape, in other words, number of rows and number of columns in the DataFrame, with the help of examples.
Examples
1. Get shape of DataFrame
In the following example, we will find the shape of DataFrame. Also, you can get the number of rows or number of columns using index on the shape.
Python Program
import pandas as pd
import numpy as np
# Initialize a DataFrame
df = pd.DataFrame(
[[21, 72, 67.1],
[23, 78, 69.5],
[32, 74, 56.6],
[52, 54, 76.2]],
columns=['a', 'b', 'c'])
print('The DataFrame is :\n', df)
# Get DataFrame shape
shape = df.shape
print('\nDataFrame Shape :', shape)
print('\nNumber of rows :', shape[0])
print('\nNumber of columns :', shape[1])
Run Code CopyOutput
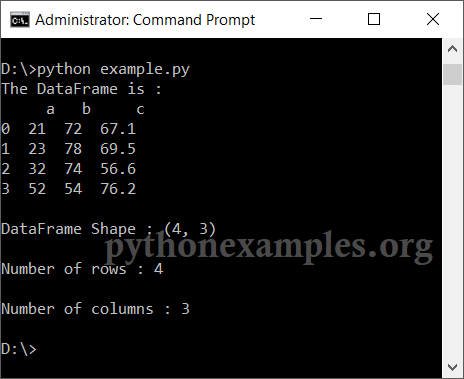
2. Shape of empty DataFrame
In this example we will initialize an empty DataFrame and try to find the shape of it. Of course, the DataFrame.shape would return (0, 0).
Python Program
import pandas as pd
# Initialize a DataFrame
df = pd.DataFrame()
print('The DataFrame is :\n', df)
# Get DataFrame shape
shape = df.shape
print('DataFrame Shape :', shape)
print('Number of rows :', shape[0])
print('Number of columns :', shape[1])
Run Code CopyOutput
The DataFrame is :
Empty DataFrame
Columns: []
Index: []
DataFrame Shape : (0, 0)
Number of rows : 0
Number of columns : 0
The number of rows is zero and the number of columns is zero.
Summary
In this tutorial of Python Examples, we learned how to find the shape of dimension of DataFrame, in other words, the number of rows and the number of columns.