Pandas Append DataFrame DataFrame.append()
pandas.DataFrame.append() function creates and returns a new DataFrame with rows of second DataFrame to the end of caller DataFrame.
In this tutorial, you’ll learn how to append a DataFrame to another DataFrame, using DataFrame.append() method, with examples.
Examples
1. Append a DataFrame to another DataFrame
In this example, we take two dataframes, and append second dataframe to the first.
Python Program
import pandas as pd
# Initialize a DataFrame
df_1 = pd.DataFrame(
[['Somu', 68, 84, 78, 96],
['Kiku', 74, 56, 88, 85],
['Ajit', 77, 73, 82, 87]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
# Initialize another DataFrame
df_2 = pd.DataFrame(
[['Amol', 72, 67, 91, 83],
['Lini', 78, 69, 87, 92]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
# Append DataFrames
df = df_1.append(df_2, ignore_index=True)
# Print DataFrames
print("df_1\n------\n",df_1)
print("\ndf_2\n------\n",df_2)
print("\ndf\n--------\n",df)
Output
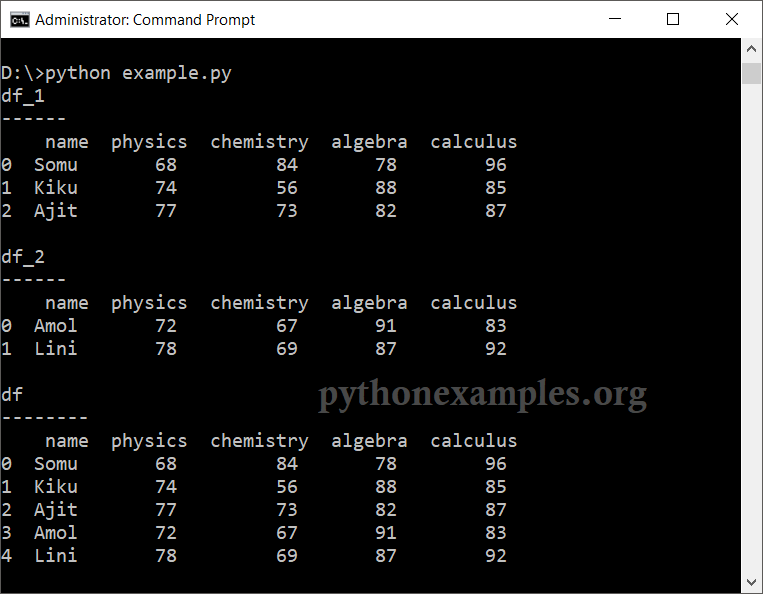
In this pandas dataframe.append() example, we passed argument ignore_index=Ture
. This helps to reorder the index of resulting dataframe. If ignore_index=False
, the output dataframe’s index looks as shown below.
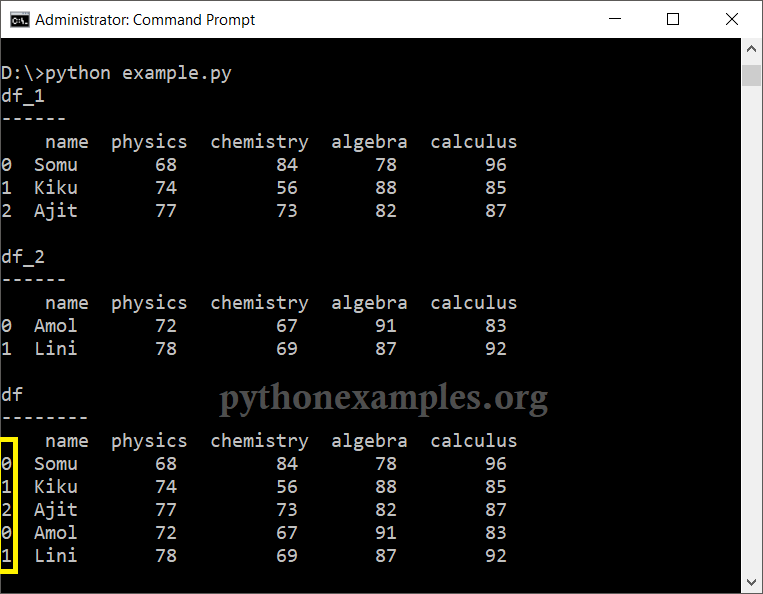
2. Append DataFrames with different column names
Now, let us take two DataFrames with different columns and append the DataFrames.
Python Program
import pandas as pd
# Initialize a DataFrame
df_1 = pd.DataFrame(
[['Somu', 68, 84, 78, 96],
['Kiku', 74, 56, 88, 85],
['Ajit', 77, 73, 82, 87]],
columns=['name', 'physics', 'chemistry','algebra','calculus'])
# Initialize another DataFrame
df_2 = pd.DataFrame(
[['Amol', 72, 67, 91, 83],
['Lini', 78, 69, 87, 92]],
columns=['name', 'physics', 'chemistry','science','calculus'])
# Append DataFrames
df = df_1.append(df_2, ignore_index=True, sort=False)
# Print DataFrames
print("df_1\n------\n",df_1)
print("\ndf_2\n------\n",df_2)
print("\ndf\n--------\n",df)
Output
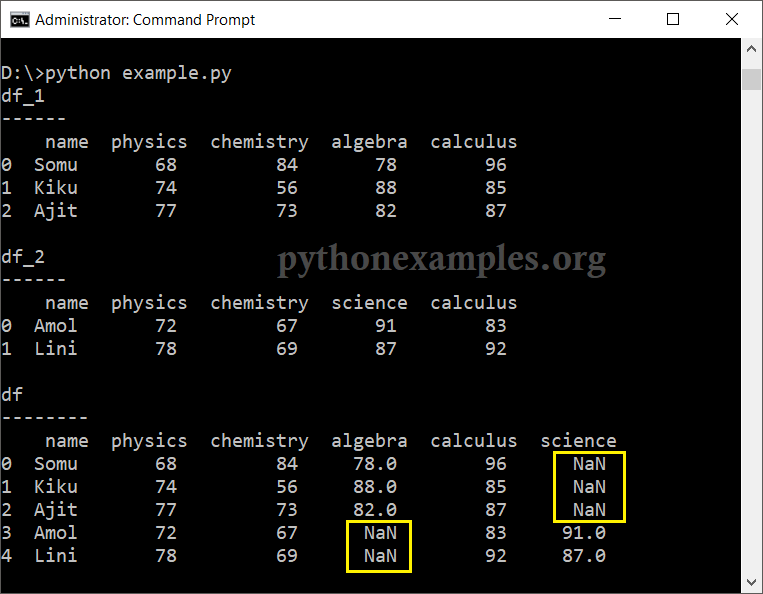
For those rows, whose corresponding column is not present, the value is defaulted to NaN. And also, the other values in the column are hosting floating values.
Summary
In this Pandas Tutorial, we learned how to append Pandas DataFrames using append() method, with the help of well detailed Python example programs.