How to delete column(s) of Pandas DataFrame? - 4 Python Examples
Pandas DataFrame - Delete Column(s)
You can delete one or multiple columns of a DataFrame.
To delete or remove only one column from Pandas DataFrame, you can use either del
keyword, pop()
function, or drop()
function on the dataframe.
To delete multiple columns from Pandas Dataframe, use drop()
function on the DataFrame.
In this tutorial, you'll learn how to delete one or more columns in a DataFrame, with the help of example programs.
Examples
1. Delete a column using del keyword
In this example, we will create a DataFrame and then delete a specified column using del keyword. The column is selected for deletion, using the column label.
Python Program
import pandas as pd
mydictionary = {'names': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]}
#create dataframe
df_marks = pd.DataFrame(mydictionary)
print('Original DataFrame\n--------------')
print(df_marks)
#delete a column
del df_marks['chemistry']
print('\n\nDataFrame after deleting column\n--------------')
print(df_marks)
Explanation
- The program imports the
pandas
library, which is essential for handling and manipulating data in tabular formats. - A dictionary named
mydictionary
is defined, containing keys ('names'
,'physics'
,'chemistry'
, and'algebra'
) with corresponding lists of values for each key. - A DataFrame named
df_marks
is created using thepd.DataFrame()
function, takingmydictionary
as its input to organize the data into rows and columns. - The original DataFrame is printed to the console, showing all columns and their respective rows of data.
- The
del
statement is used to remove the column'chemistry'
from the DataFrame, altering its structure. - The modified DataFrame, which no longer includes the
'chemistry'
column, is printed to display the updated structure with the remaining columns:'names'
,'physics'
, and'algebra'
.
Output
Original DataFrame
--------------
names physics chemistry algebra
0 Somu 68 84 78
1 Kiku 74 56 88
2 Amol 77 73 82
3 Lini 78 69 87
DataFrame after deleting column
--------------
names physics algebra
0 Somu 68 78
1 Kiku 74 88
2 Amol 77 82
3 Lini 78 87
We have deleted chemistry column from the dataframe.
2. Delete a column using pop() function
In this example, we will create a DataFrame and then use pop() function on the dataframe to delete a specific column. The column is selected for deletion, using the column label.
Python Program
import pandas as pd
mydictionary = {'names': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]}
#create dataframe
df_marks = pd.DataFrame(mydictionary)
print('Original DataFrame\n--------------')
print(df_marks)
#delete column
df_marks.pop('chemistry')
print('\n\nDataFrame after deleting column\n--------------')
print(df_marks)
Explanation
- This Python program demonstrates the creation and manipulation of a Pandas DataFrame.
- The dictionary
mydictionary
contains data with keys as column names ('names', 'physics', 'chemistry', 'algebra') and values as lists representing the column data. - The
pd.DataFrame()
function converts the dictionary into a DataFrame nameddf_marks
. - The program prints the original DataFrame using the
print()
function. - The
pop()
method is used to remove the 'chemistry' column from the DataFrame. - The modified DataFrame (after removing the 'chemistry' column) is printed to show the changes.
Output
Original DataFrame
--------------
names physics chemistry algebra
0 Somu 68 84 78
1 Kiku 74 56 88
2 Amol 77 73 82
3 Lini 78 69 87
DataFrame after deleting column
--------------
names physics algebra
0 Somu 68 78
1 Kiku 74 88
2 Amol 77 82
3 Lini 78 87
We have deleted chemistry column from the dataframe.
3. Delete a column using drop() function
In this example, we will use drop() function on the dataframe to delete a specific column. We use column label to select a column for deletion.
Python Program
import pandas as pd
mydictionary = {'names': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]}
#create dataframe
df_marks = pd.DataFrame(mydictionary)
print('Original DataFrame\n--------------')
print(df_marks)
#delete column
df_marks = df_marks.drop(['chemistry'], axis=1)
print('\n\nDataFrame after deleting column\n--------------')
print(df_marks)
Explanation
- The program begins by importing the
pandas
library for data manipulation. - A dictionary
mydictionary
is created with keys ('names'
,'physics'
,'chemistry'
, and'algebra'
) and corresponding lists of values. - A DataFrame
df_marks
is created using thepd.DataFrame()
function withmydictionary
as the input. - The original DataFrame is printed to display all columns and rows.
- The
drop()
method is used to delete the column'chemistry'
. The argumentaxis=1
specifies that the operation is being performed on columns (not rows). - The updated DataFrame, with the column
'chemistry'
removed, is printed to display the modified structure.
Output
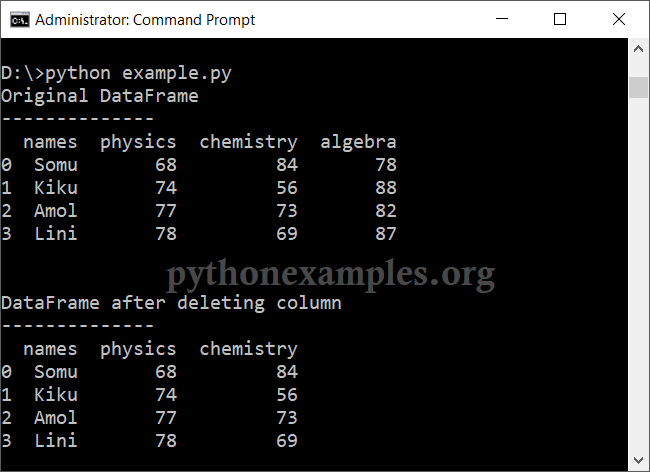
4. Delete multiple columns using drop() function
In this example, we will use drop() function on the dataframe to delete multiple columns. We use array of column labels to select columns for deletion.
Python Program
import pandas as pd
mydictionary = {'names': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]}
#create dataframe
df_marks = pd.DataFrame(mydictionary)
print('Original DataFrame\n--------------')
print(df_marks)
#delete columns
df_marks = df_marks.drop(['algebra', 'chemistry'], axis=1)
print('\n\nDataFrame after deleting column\n--------------')
print(df_marks)
Explanation
- The program imports the
pandas
library for data manipulation. - A dictionary named
mydictionary
is created, which contains keys ('names'
,'physics'
,'chemistry'
, and'algebra'
) with corresponding lists of values. - A DataFrame named
df_marks
is created using thepd.DataFrame()
function withmydictionary
as input. - The original DataFrame is printed to show all columns and rows.
- The
drop()
method is used to delete the columns'algebra'
and'chemistry'
. The argumentaxis=1
specifies that columns are being removed. - The modified DataFrame, with the remaining columns
'names'
and'physics'
, is printed to display the updated structure.
Output
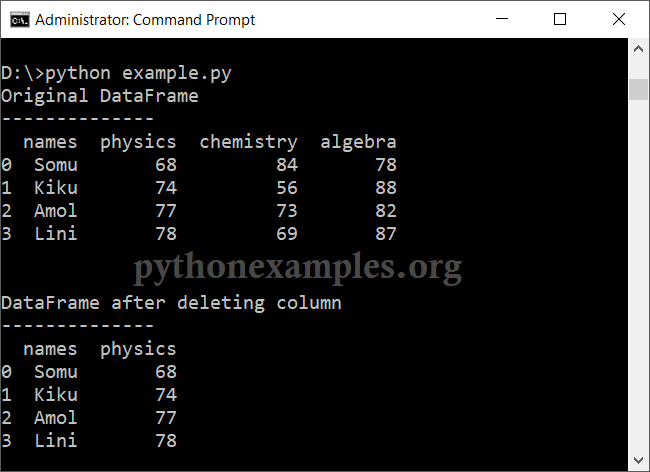
Summary
In this Pandas Tutorial, we learned how to delete a column from Pandas DataFrame using del keyword, pop() method and drop() method, with the help of well detailed Python Examples.