Pandas – Render DataFrame as HTML Table
You can convert DataFrame to a table in HTML, to represent the DataFrame in web pages.
To render a Pandas DataFrame to HTML Table, use pandas.DataFrame.to_html()
method.
The total DataFrame is converted to <table>
html element, while the column names are wrapped under <thead>
table head html element. And, each row of DataFrame is converted to a row <tr>
in HTML table.
Examples
1. Render DataFrame as HTML table
In this example, we will initialize a DataFrame and render it into HTML Table.
Python Program
import pandas as pd
# create dataframe
df_marks = pd.DataFrame({'name': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]})
# render dataframe as html
html = df_marks.to_html()
print(html)
Output
<table border="1" class="dataframe">
<thead>
<tr style="text-align: right;">
<th></th>
<th>name</th>
<th>physics</th>
<th>chemistry</th>
<th>algebra</th>
</tr>
</thead>
<tbody>
<tr>
<th>0</th>
<td>Somu</td>
<td>68</td>
<td>84</td>
<td>78</td>
</tr>
<tr>
<th>1</th>
<td>Kiku</td>
<td>74</td>
<td>56</td>
<td>88</td>
</tr>
<tr>
<th>2</th>
<td>Amol</td>
<td>77</td>
<td>73</td>
<td>82</td>
</tr>
<tr>
<th>3</th>
<td>Lini</td>
<td>78</td>
<td>69</td>
<td>87</td>
</tr>
</tbody>
</table>
Let us write the html data to a file using Python.
Python Program
import pandas as pd
#create dataframe
df_marks = pd.DataFrame({'name': ['Somu', 'Kiku', 'Amol', 'Lini'],
'physics': [68, 74, 77, 78],
'chemistry': [84, 56, 73, 69],
'algebra': [78, 88, 82, 87]})
#render dataframe as html
html = df_marks.to_html()
#write html to file
text_file = open("index.html", "w")
text_file.write(html)
text_file.close()
The file will be created with html data in the current working directory.
Now, open the html file with browser. The output should look similar to the following screenshot.
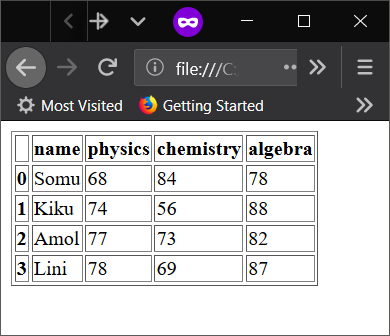
Summary
In this Pandas Tutorial, we have rendered/converted a Pandas DataFrame to HTML Table.